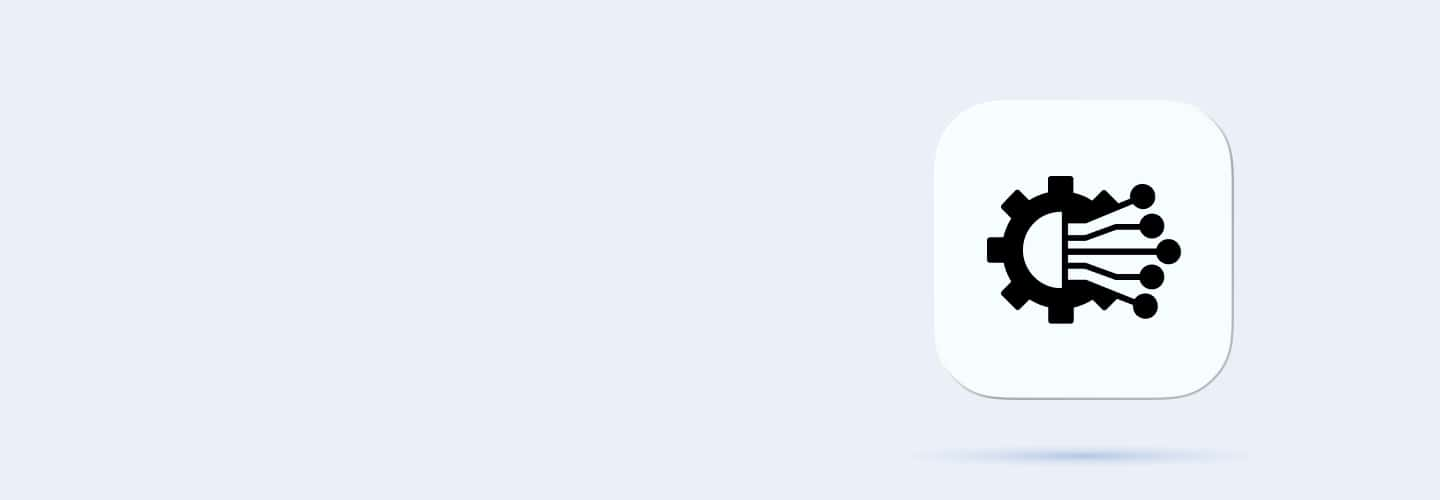
Q121
Q121 Which HTTP header is used to specify the media type of the resource?
Content-Type
Accept
Authorization
Cache-Control
Q122
Q122 How do you set a custom HTTP status code in an Express.js response?
response.setStatus(404);
response.status(404).send('Not Found');
response.httpCode(404);
response.error(404);
Q123
Q123 Why might a browser display a "400 Bad Request" error?
Malformed URL
Large request payload
Invalid syntax in the request
Unsupported protocol
Q124
Q124 Why might an HTTP response return a "500 Internal Server Error"?
Server-side script error
Database connection failure
Misconfigured server
Exceeded memory limit
Q125
Q125 What does REST stand for?
Representational State Transfer
Remote Entity Service Transmission
Resource Entity Service Type
Resource State Transfer
Q126
Q126 Which HTTP method is typically used to update a resource in a RESTful API?
GET
POST
PUT
DELETE
Q127
Q127 What is a key characteristic of RESTful web services?
Stateful communication
Server-side sessions
Stateless communication
Requires SOAP
Q128
Q128 Which of the following is a standard HTTP response code for a successful DELETE request?
200
201
204
400
Q129
Q129 Why are URI endpoints crucial in RESTful APIs?
They define methods
They identify resources
They store server data
They ensure authentication
Q130
Q130 How do you make a GET request to a RESTful API using Fetch API?
fetch('url', { method: 'GET' });
fetch('url');
fetch.get('url');
GET.fetch('url');
Q131
Q131 How do you parse JSON data returned from a RESTful API in JavaScript?
response.data();
response.toJSON();
response.json();
response.parseJSON();
Q132
Q132 How do you send custom headers in a RESTful API request using Axios?
axios.get('url', headers: { Authorization: 'token' });
axios.get('url', { headers: { Authorization: 'token' } });
axios.get('url', { header: 'Authorization: token' });
axios.headers('url', Authorization: 'token');
Q133
Q133 Why might a RESTful API request return a 401 Unauthorized error?
Invalid credentials
Missing authentication token
Token is expired
Invalid URL
Q134
Q134 Why might a RESTful API return a CORS (Cross-Origin Resource Sharing) error?
API URL is invalid
Server not configured for CORS
Browser is outdated
HTTP method is unsupported
Q135
Q135 What is the primary purpose of cookies in web development?
To store session data on the server
To store small amounts of data in the browser
To increase website speed
To prevent XSS attacks
Q136
Q136 What is the main difference between cookies and local storage?
Cookies have larger storage limits
Cookies are sent with each HTTP request
Local storage is server-side
Local storage expires automatically
Q137
Q137 Which attribute of a cookie ensures it is only sent over HTTPS?
Secure
HttpOnly
Domain
Path
Q138
Q138 What is the purpose of the HttpOnly attribute in cookies?
It encrypts cookies
It prevents client-side scripts from accessing cookies
It extends cookie lifespan
It sets the domain of the cookie
Q139
Q139 How do you set a cookie in JavaScript?
document.setCookie("user=John");
document.cookie = "user=John";
set.cookie("user=John");
cookie.document("user=John");
Q140
Q140 How do you store data in local storage using JavaScript?
localStorage.setItem('key', 'value');
localStorage.addItem('key', 'value');
localStorage.store('key', 'value');
localStorage.saveItem('key', 'value');
Q141
Q141 Why might a cookie fail to store on the client?
Cookie exceeds size limit
Domain mismatch
Incorrect attributes
Secure attribute not set
Q142
Q142 Why might local storage fail to retrieve data?
Incorrect key name
Browser does not support local storage
Storage was cleared
Access denied by browser settings
Q143
Q143 What is the primary purpose of web performance optimization?
To increase server capacity
To reduce page load times
To improve SEO
To minimize JavaScript code
Q144
Q144 Which technique can reduce the size of JavaScript files for faster loading?
Minification
Normalization
Caching
Compression
Q145
Q145 How does lazy loading improve performance?
It compresses assets
It loads images and resources only when needed
It prioritizes large files
It minimizes CSS usage
Q146
Q146 What is the purpose of a Content Delivery Network (CDN) in optimization?
To host databases
To distribute content across multiple servers
To compress web pages
To minimize DOM size
Q147
Q147 Which HTML attribute can be used to defer the loading of JavaScript files?
async
defer
lazy
postload
Q148
Q148 How do you enable Gzip compression on an Nginx server?
gzip on;
enable gzip;
nginx gzip enable;
compress gzip on;
Q149
Q149 Why might a website have poor performance despite using a CDN?
CDN is misconfigured
Assets are not optimized
Too many HTTP requests
Insufficient bandwidth
Q150
Q150 Why might browser caching not work as expected?
Incorrect cache-control headers
Cache expiration time not set
Dynamic content not cacheable
User disabled browser cache