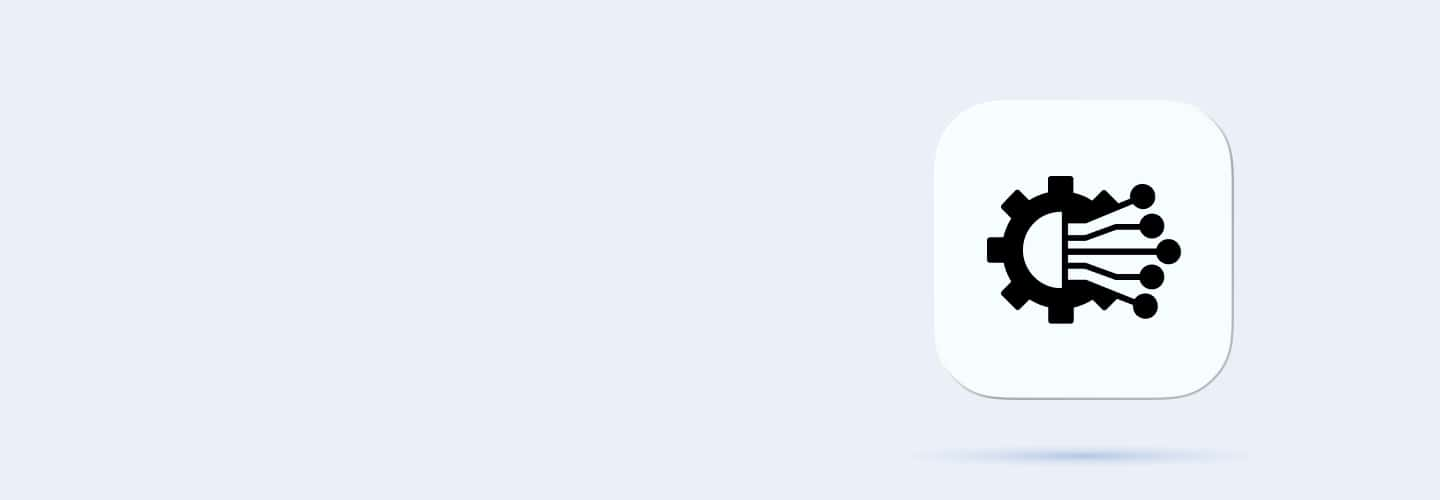
Q91
Q91 What is the correct syntax to write a basic HTTP server in Node.js?
require('server').start();
http.createServer().listen(3000);
node.createServer('3000');
server.listen('3000');
Q92
Q92 In PHP, how do you connect to a MySQL database using mysqli?
mysqli_connect('localhost', 'user', 'password', 'database');
connect_db('localhost', 'user', 'password', 'database');
php_connect('localhost', 'user', 'password', 'database');
db_connect('localhost', 'user', 'password', 'database');
Q93
Q93 How do you define a route in an Express.js application?
app.get('/', (req, res) => { res.send('Hello'); });
route('/', 'GET', 'Hello');
express('/', 'GET', 'Hello');
app.route('/', 'Hello');
Q94
Q94 Why might a server fail to start in Node.js?
Missing modules
Incorrect port configuration
Syntax error
Invalid environment variables
Q95
Q95 Why might a PHP script not connect to a MySQL database?
Incorrect database credentials
MySQL server not running
Missing mysqli extension
All of the above
Q96
Q96 A POST request to an Express.js route does not work. What could be the reason?
Missing middleware
Route method mismatch
Invalid data format
Improperly configured headers
Q97
Q97 What is the primary purpose of web hosting?
To manage DNS settings
To provide servers for storing website files
To configure databases
To design web pages
Q98
Q98 Which of the following is a shared hosting characteristic?
Dedicated server for one user
Limited resources shared among multiple users
Exclusive IP address
High cost
Q99
Q99 What is a CDN in the context of web hosting?
Content Delivery Network
Cloud Data Network
Compressed Data Network
Central Data Node
Q100
Q100 Which of the following hosting types is most suitable for large-scale applications?
Shared hosting
VPS hosting
Dedicated hosting
Free hosting
Q101
Q101 How does cloud hosting differ from traditional hosting?
It is cheaper
It uses multiple servers instead of one
It is only for small websites
It doesn't require a database
Q102
Q102 Which of the following is the correct command to deploy an application to Heroku?
heroku upload app
heroku deploy app
git push heroku main
heroku deploy main
Q103
Q103 How do you configure an Nginx server to host a static website?
Use proxy_pass for the HTML files
Set root to the directory containing the static files
Set listen to 443
Set server_name to localhost
Q104
Q104 What is the correct syntax to configure a custom domain for a deployed Firebase project?
firebase deploy domain example.com
firebase set domain example.com
firebase hosting:domain add example.com
firebase domain add example.com
Q105
Q105 Why might a deployed website not load in the browser?
DNS settings not configured
Server is offline
Incorrect file permissions
Application crash on deployment
Q106
Q106 Why might a static website hosted on GitHub Pages not display properly?
Missing index.html file
Incorrect branch settings
Repository is private
Unresolved relative paths
Q107
Q107 What is the purpose of HTTPS in web security?
To speed up website loading
To encrypt data transmission
To prevent server downtime
To block advertisements
Q108
Q108 What is Cross-Site Scripting (XSS)?
Injecting malicious scripts into websites
Breaking server encryption
Exploiting server downtime
Attacking DNS records
Q109
Q109 Which security measure can mitigate SQL injection attacks?
Input validation
Using secure cookies
Implementing HTTPS
Disabling caching
Q110
Q110 What is the primary purpose of a Content Security Policy (CSP)?
To block cookies
To prevent unauthorized scripts
To optimize page performance
To manage server downtime
Q111
Q111 What is a CSRF (Cross-Site Request Forgery) attack?
A brute-force attack on passwords
An unauthorized action performed on behalf of an authenticated user
A type of DoS attack
A method to steal cookies
Q112
Q112 How do you implement HTTPS on a web server using Nginx?
Add an ssl_certificate and ssl_certificate_key in the server block
Enable proxy_pass
Set listen to 80
Disable server_name
Q113
Q113 How do you set HTTP security headers using Node.js?
response.setHeader('X-Content-Type-Options', 'nosniff');
response.addHeader('X-Content-Type', 'nosniff');
response.header('Content-Security-Policy', 'nosniff');
response.securityHeader('X-Content-Type', 'nosniff');
Q114
Q114 Why might a website show a "Mixed Content" warning in the browser?
Using both HTTP and HTTPS resources
Incorrect server configuration
Missing CSS files
Invalid HTML syntax
Q115
Q115 Why might a web application fail to block an XSS attack?
Improper input validation
No Content Security Policy (CSP)
Lack of secure encoding
Insufficient testing
Q116
Q116 What does HTTP stand for?
HyperText Transfer Protocol
HyperText Transmission Process
High-Tech Transfer Protocol
Hyperlink Transmission Protocol
Q117
Q117 Which HTTP method is used to retrieve data from a server?
GET
POST
PUT
DELETE
Q118
Q118 What is the purpose of the HTTP HEAD method?
To retrieve data
To send data
To fetch headers only
To delete resources
Q119
Q119 What does a 404 HTTP status code indicate?
Successful request
Client error
Server error
Redirection
Q120
Q120 What is the purpose of the HTTP OPTIONS method?
To request supported HTTP methods
To upload files
To redirect a request
To fetch cookies