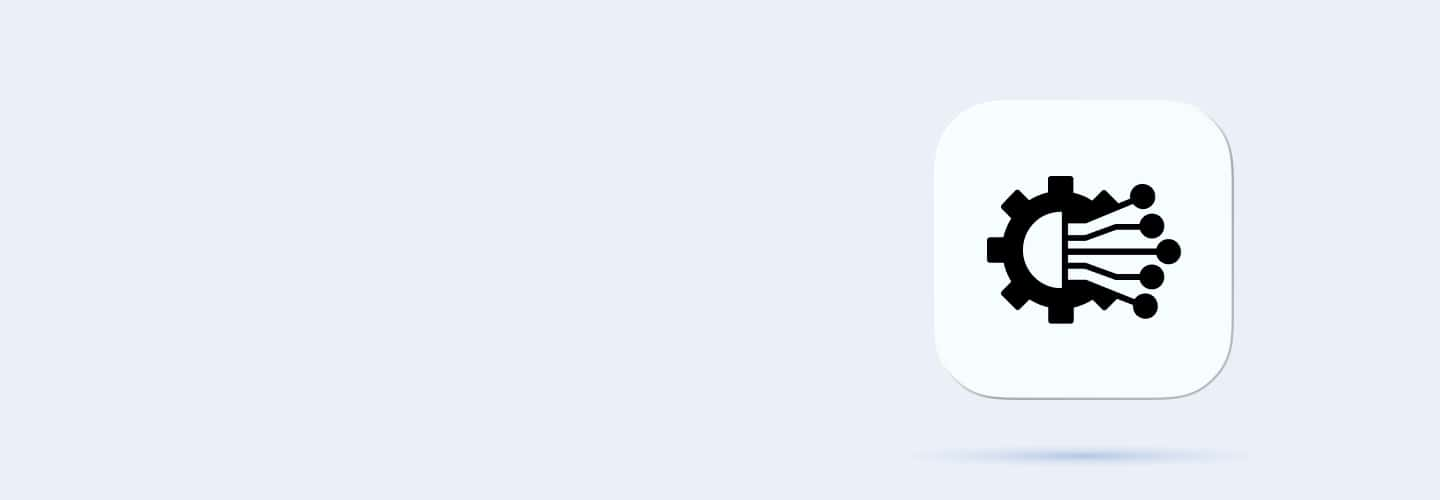
Q61
Q61 Which CSS property is used to make an image responsive?
max-width
position
flex
float
Q62
Q62 How do you implement a flexible grid using CSS?
display: block;
display: grid;
display: flex;
display: inline-flex;
Q63
Q63 Why might a responsive layout not adapt properly on smaller screens?
Missing meta viewport tag
Fixed-width elements
Incorrect media query syntax
Large image sizes
Q64
Q64 A navigation bar overflows on smaller screens. What could be the cause?
Excessive padding
Improper CSS flex properties
Fixed dimensions
Invalid HTML structure
Q65
Q65 Why might flexbox-based layouts break on older browsers?
Flex properties not supported
Incorrect syntax
CSS file not linked
Incompatible images
Q66
Q66 Which of the following frameworks uses a virtual DOM for performance optimization?
Angular
React
Vue
All of the above
Q67
Q67 What is the primary difference between Angular and React?
Angular is a library, React is a framework
Angular uses two-way binding, React uses one-way binding
React supports TypeScript, Angular does not
Angular does not use components
Q68
Q68 Which of the following features is unique to Vue.js?
JSX support
Directives like v-bind
Component lifecycle hooks
Virtual DOM
Q69
Q69 Which framework uses a hierarchical dependency injection system?
React
Angular
Vue
None of the above
Q70
Q70 What is the correct syntax for creating a functional component in React?
function MyComponent() { return <h1>Hello</h1>; }
def MyComponent() { <h1>Hello</h1>; }
function MyComponent => { return <h1>Hello</h1>; }
createComponent MyComponent { <h1>Hello</h1>; }
Q71
Q71 How do you bind a class conditionally in Vue.js?
class="isActive"
:class="{ active: isActive }"
v-class="{ active: isActive }"
bind-class="active"
Q72
Q72 What is the correct syntax to define a two-way data binding in Angular?
[ng-model]="data"
[(ngModel)]="data"
{ngModel: data}
bind="data"
Q73
Q73 A React component fails to update its state. What could be the issue?
State mutation
Improper setState usage
Undefined state variable
Component lifecycle mismatch
Q74
Q74 Why might a Vue.js app fail to display data bindings?
Missing el property
Incorrect Vue instance syntax
Improper use of directives
Unregistered components
Q75
Q75 Why might Angular’s ngModel directive not work as expected?
Missing FormsModule import
Incorrect two-way binding syntax
Component template error
Uninitialized variables
Q76
Q76 What does AJAX stand for?
Asynchronous JavaScript and XML
Advanced Java Application
Automatic Java API XML
Asynchronous Java Action XML
Q77
Q77 Which HTTP method is typically used in AJAX requests to send data to the server?
GET
POST
PUT
DELETE
Q78
Q78 What is a key difference between AJAX and the Fetch API?
Fetch is synchronous
Fetch supports Promises
AJAX is browser-compatible
AJAX does not support XML
Q79
Q79 Why might you prefer Fetch API over XMLHttpRequest in modern web applications?
Simplified syntax
Promise-based architecture
Supports JSON natively
All of the above
Q80
Q80 Which is the correct syntax to make a GET request using Fetch API?
fetch('url').then(response => response.json());
fetch('url', method: 'GET').done(data);
GET.fetch('url').success(data => data.json());
fetch.get('url').json();
Q81
Q81 How do you send JSON data in a POST request using Fetch API?
fetch('url', { method: 'POST', data: JSON.stringify(obj) });
fetch('url', { method: 'POST', body: JSON.stringify(obj) });
fetch.post('url', { body: obj });
fetch('url').post(JSON.stringify(obj));
Q82
Q82 How can you include custom headers in a Fetch request?
fetch('url', { headers: { 'Content-Type': 'application/json' } });
fetch.setHeaders({ 'Content-Type': 'application/json' });
fetch.headers({ 'Content-Type': 'application/json' });
fetch('url').headers = { 'Content-Type': 'application/json' };
Q83
Q83 Why might an AJAX request fail to fetch data?
Incorrect URL
CORS error
Network issues
Invalid HTTP method
Q84
Q84 A Fetch API request throws a "TypeError: Failed to fetch" error. What could be the reason?
Network connectivity issues
CORS restrictions
Invalid URL
Timeout configuration issues
Q85
Q85 Why might a JSON response from a Fetch API request not parse correctly?
Invalid JSON format
Incorrect response headers
Syntax errors in JSON
Response is empty
Q86
Q86 What is the primary purpose of server-side scripting?
To handle database interactions
To design UI
To style web pages
To validate client data
Q87
Q87 Which of the following is a server-side scripting language?
JavaScript
HTML
PHP
CSS
Q88
Q88 What is the primary function of Node.js?
To serve static files
To execute JavaScript on the server
To style content
To handle web hosting
Q89
Q89 What is a middleware in server-side scripting?
A database connection
A function handling requests and responses
A static file
A client-side library
Q90
Q90 How does server-side rendering differ from client-side rendering?
It renders content dynamically in the browser
It improves SEO by rendering content on the server
It reduces server load
It only works offline