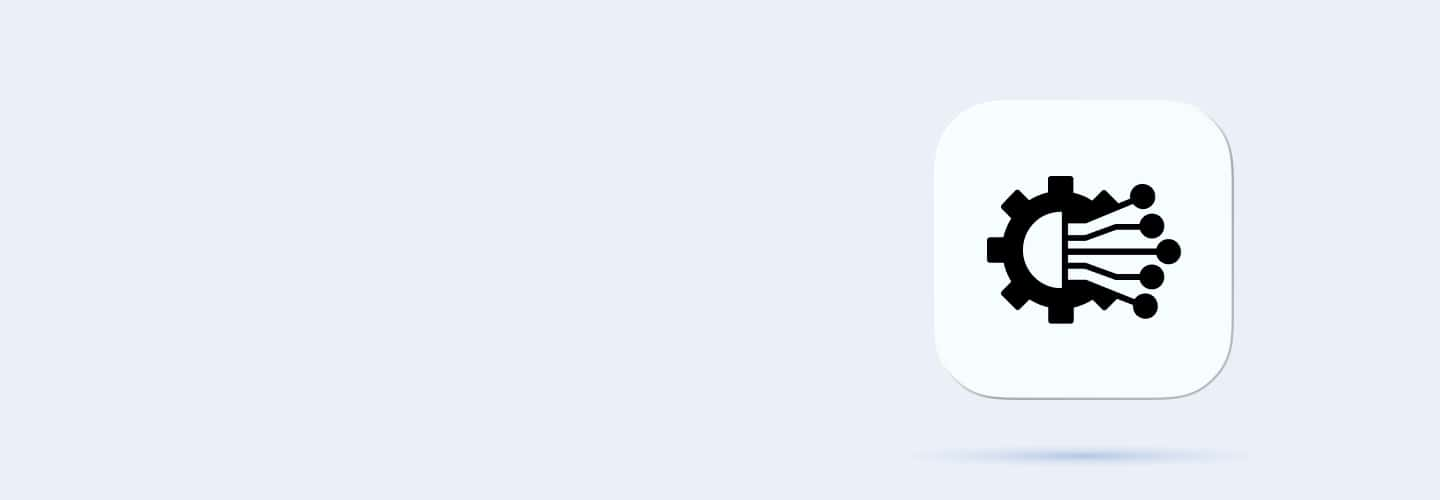
Q31
Q31 A CSS file is linked but styles are not applied. What could be a possible cause?
Missing rel="stylesheet"
Incorrect path in href
CSS syntax error
HTML is not valid
Q32
Q32 Why might a CSS grid layout not work as expected?
Missing display: grid
Incorrect grid-template syntax
Unsupported browser
Improper media queries
Q33
Q33 What is JavaScript primarily used for in web development?
Styling web pages
Creating dynamic web content
Managing databases
Designing UI
Q34
Q34 Which of the following is a valid JavaScript data type?
Integer
Character
Boolean
Float
Q35
Q35 What is the correct syntax to write a comment in JavaScript?
// This is a comment
<!-- This is a comment -->
# This is a comment
/* This is a comment */
Q36
Q36 Which keyword is used to declare a variable in JavaScript?
let
define
create
var
Q37
Q37 What does the typeof operator do in JavaScript?
Determines the type of a variable
Changes the data type
Declares a variable
Checks nullability
Q38
Q38 How do you write a function in JavaScript?
function myFunc() {}
def myFunc() {}
func myFunc() {}
method myFunc() {}
Q39
Q39 What is the correct way to display a message in the browser console?
alert("Hello")
console.log("Hello")
document.write("Hello")
print("Hello")
Q40
Q40 How do you call a function named myFunction in JavaScript?
call myFunction()
myFunction()
execute myFunction()
invoke myFunction()
Q41
Q41 Why might a JavaScript function not run when the page loads?
Function is not defined
Syntax error
Function call is missing
All of the above
Q42
Q42 Why might console.log(variableName) throw an error in JavaScript?
Variable is not defined
Incorrect syntax
Reserved keyword used as variable name
All of the above
Q43
Q43 Why does the following code throw an error: const a; a = 5;?
Missing semicolon
const variables must be initialized
Incorrect syntax
Variable redeclaration
Q44
Q44 What does DOM stand for in web development?
Document Object Model
Data Object Mapping
Dynamic Object Management
Document Outline Mapping
Q45
Q45 Which JavaScript method is used to access an element by its ID in the DOM?
getElementById()
querySelector()
getElementsByClassName()
getIdElement()
Q46
Q46 What is the role of document in DOM manipulation?
To define event listeners
To provide access to the entire HTML document
To store styles
To declare variables
Q47
Q47 What is the difference between innerHTML and textContent in DOM manipulation?
innerHTML sets text and HTML elements
textContent is faster
Both
Neither
Q48
Q48 Which code snippet changes the text of a paragraph with the ID "demo"?
document.querySelector("demo").text = "Hello";
document.getElementById("demo").innerHTML = "Hello";
document.getById("demo").html = "Hello";
document.get("demo").value = "Hello";
Q49
Q49 How do you add a new list item to an unordered list with the ID "myList"?
appendChild("li");
document.getElementById("myList").appendChild(liElement);
addChild("myList", "li");
document.get("myList").addChild("li");
Q50
Q50 Which JavaScript method is used to remove a specific element from the DOM?
deleteElement()
removeChild()
removeElement()
destroyElement()
Q51
Q51 How can you dynamically create a new <div> element in the DOM?
createElement('div')
document.createElement('div')
new Div()
create('div')
Q52
Q52 Why might a DOM manipulation script fail to execute?
Syntax errors in the script
Script is placed before DOM loads
Invalid element selectors
Missing event listeners
Q53
Q53 A dynamically added button does not respond to a click event. What could be the reason?
Incorrect event listener type
Event listener added before element creation
Syntax error in handler
Improper callback function
Q54
Q54 Why might document.querySelector(".myClass").innerHTML return null?
Element does not exist
Class name is misspelled
JavaScript executes before DOM loads
Element is dynamically generated later
Q55
Q55 What is the primary goal of responsive web design?
To create interactive content
To make web pages adapt to different screen sizes
To enhance web security
To improve website loading speed
Q56
Q56 Which of the following is used in responsive design to make layouts flexible?
Grid systems
CSS media queries
Both
None of the above
Q57
Q57 What does the viewport meta tag in HTML control?
Page content width
Browser zoom level
Device orientation
Text alignment
Q58
Q58 Which of the following is a common CSS unit for responsive design?
px
cm
vh
in
Q59
Q59 How do CSS media queries enhance responsive design?
By dynamically loading images
By defining breakpoints for styling
By optimizing performance
By adjusting text readability
Q60
Q60 Which CSS media query will apply styles to devices with a maximum width of 600px?
@media (min-width: 600px)
@media (max-width: 600px)
@media (width: 600px)
@media (device-width: 600px)