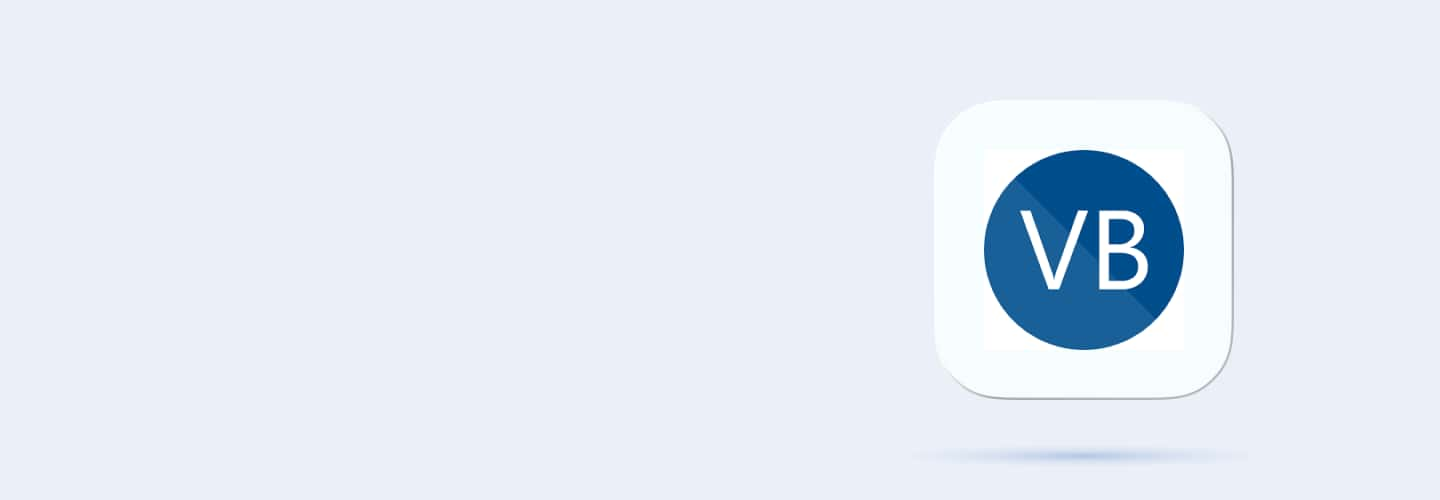
Q91
Q91 Which namespace is essential for ADO.NET database operations?
System.Data
System.IO
System.Db
System.Sql
Q92
Q92 What is the purpose of the SqlConnection class in ADO.NET?
To execute SQL queries
To manage database connections
To handle SQL errors
To create tables
Q93
Q93 What does the ExecuteNonQuery method of SqlCommand return?
The number of rows affected
The first row of the result
The last inserted ID
A Boolean value
Q94
Q94 What is the purpose of the DataAdapter in ADO.NET?
To connect the database to the UI
To manage connections
To fill DataSet and update databases
To execute queries
Q95
Q95 What is the correct way to open a database connection in VB.NET?
connection.Open()
connection.Start()
connection.Execute()
connection.Begin()
Q96
Q96 What is the output of the following code?
Dim query As String = "SELECT COUNT(*) FROM Users" Dim cmd As New SqlCommand(query, connection) Dim count As Integer = cmd.ExecuteScalar() Console.WriteLine(count)
The count of users
Error
Null
Zero
Q97
Q97 Which method is used to populate a DataSet in VB.NET?
Fill
Load
Populate
Execute
Q98
Q98 Identify the issue in the following code:
Dim cmd As New SqlCommand("SELECT * FROM Users", connection) Dim reader As SqlDataReader = cmd.ExecuteReader() reader.Close() connection.Close()
Reader is not properly disposed
Command is not initialized
Connection is already closed
Reader is not used
Q99
Q99 What happens if the database connection string is incorrect?
Connection opens successfully
Throws an exception
Returns null
Closes the application
Q100
Q100 How can you debug a failed SQL query execution in VB.NET?
Check the query syntax
Inspect parameters
Check database connection
All of these
Q101
Q101 Why might a SqlCommand fail to execute in VB.NET?
Incorrect SQL syntax
Closed connection
Incorrect parameters
All of these
Q102
Q102 What does LINQ stand for in VB.NET?
Language Integrated Query
Logic Integrated Query
List Inquiry Query
Language Internal Query
Q103
Q103 Which namespace is required for LINQ in VB.NET?
System.Linq
System.Query
System.Collections
System.IO
Q104
Q104 What is the main advantage of using LINQ in VB.NET?
Simplifies data queries
Increases performance
Eliminates the need for SQL
Improves debugging
Q105
Q105 What does the "Select" keyword do in a LINQ query?
Filters data
Projects data
Groups data
Sorts data
Q106
Q106 How does deferred execution work in LINQ?
Executes the query immediately
Executes the query when results are enumerated
Executes the query at compile time
Executes the query at runtime without conditions
Q107
Q107 What is the output of the following code?
Dim nums As Integer() = {1, 2, 3} Dim query = From n In nums Where n > 1 Select n Console.WriteLine(query.Count)
1
2
3
Error
Q108
Q108 How do you perform sorting in a LINQ query in VB.NET?
Using the Sort function
Using Order By clause
Using Group By clause
Using Filter clause
Q109
Q109 What will the following code output?
Dim nums As Integer() = {1, 2, 2, 3} Dim query = nums.Distinct() Console.WriteLine(query.Count)
4
3
2
Error
Q110
Q110 What happens if a LINQ query references a null object?
Throws a runtime exception
Returns an empty result
Throws a compile-time error
Executes without error
Q111
Q111 How can you debug a LINQ query in VB.NET?
Add logging
Use breakpoints
Inspect variables in the Watch window
All of these
Q112
Q112 What is the purpose of debugging in VB.NET?
To remove unused variables
To find and fix errors in the code
To write test cases
To improve performance
Q113
Q113 Which Visual Studio window displays runtime exceptions during debugging?
Output Window
Immediate Window
Error List
Call Stack
Q114
Q114 What is unit testing in VB.NET?
Testing individual units of code
Testing the entire application
Testing database connections
Testing UI elements
Q115
Q115 What is the role of mock objects in VB.NET testing?
To replace actual objects in tests
To increase test performance
To reduce code execution time
To debug faster
Q116
Q116 What does the following code output?
Debug.WriteLine("Debugging Message")
Debugging Message
Error
No output
Compiles only
Q117
Q117 How do you assert a condition in a test case using VB.NET?
If condition Then Assert.Fail()
Assert.That(condition)
Assert.IsTrue(condition)
Assert.Equals(condition)
Q118
Q118 What is the output of the following code?
Try Throw New Exception("Error") Catch ex As Exception Debug.WriteLine(ex.Message) End Try
No output
Error
Throws Exception
Error printed to debug window
Q119
Q119 What happens if you set a breakpoint in a VB.NET program?
Pauses execution at the breakpoint
Displays runtime errors
Terminates the application
Skips the line
Q120
Q120 How can you inspect the value of a variable during debugging in Visual Studio?
Use the Debug.WriteLine method
Hover over the variable
Use the Immediate Window
Both hover and Immediate Window