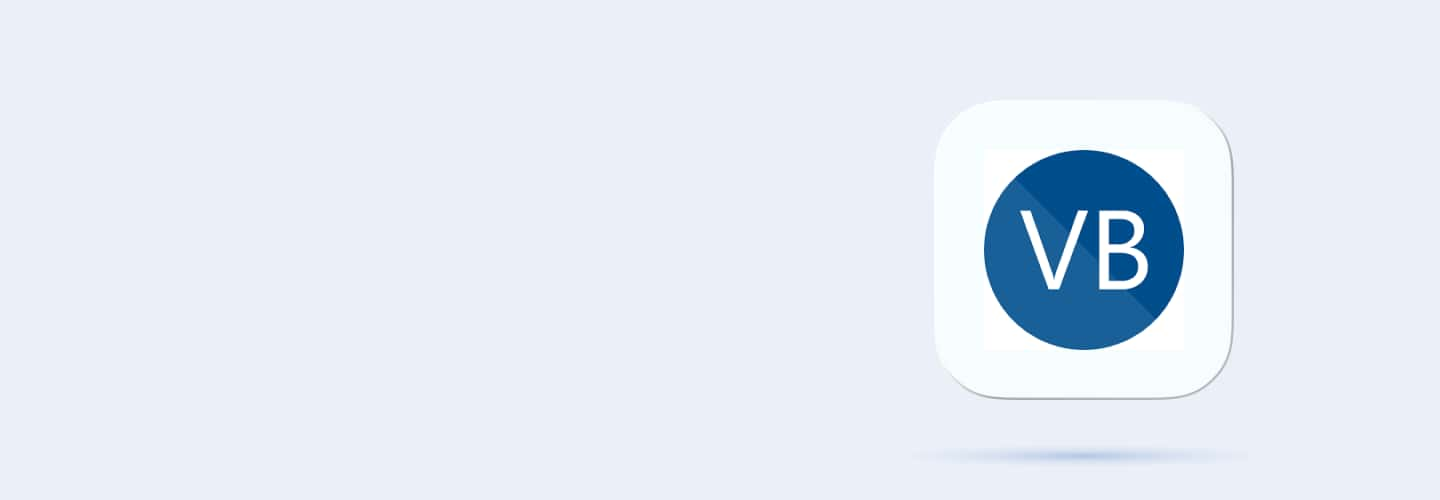
Q61
Q61 Identify the error in the following code:
Try Dim x As Integer = CInt("abc") Catch ex As FormatException Console.WriteLine("Invalid format") End Try
Invalid format
abc
0
Exception
Q62
Q62 What is the output of the following code?
Try Throw New Exception("Test Exception") Catch ex As Exception Console.WriteLine(ex.Message) End Try
Test Exception
Exception
Error
No Output
Q63
Q63 What happens if there is no Catch block for an exception in VB.NET?
The program crashes
The program continues
The program ignores the exception
The program stops execution gracefully
Q64
Q64 How can you identify the type of exception during debugging in VB.NET?
Check the exception message
Use a breakpoint
Hover over the exception object
Use the Watch window
Q65
Q65 While debugging, an exception is not being caught. What could be the reason?
The exception type is not specified in the Catch block
The exception occurs in a Finally block
The exception is not thrown
The exception is of type DivideByZeroException
Q66
Q66 Which namespace is used for file handling in VB.NET?
System.File
System.IO
System.Data
System.Text
Q67
Q67 Which class is used to create a new file in VB.NET?
FileCreate
FileMaker
FileStream
File
Q68
Q68 What is the purpose of the StreamReader class in VB.NET?
To write data to a file
To delete a file
To read characters from a file
To execute file operations
Q69
Q69 Which method of the File class is used to check if a file exists?
FileExists
CheckFile
Exists
IsFile
Q70
Q70 What is the purpose of the FileMode enumeration in VB.NET?
To set file access permissions
To specify how a file should be opened or created
To handle file exceptions
To manage file paths
Q71
Q71 What will the following code do?
Dim writer As New StreamWriter("test.txt") writer.WriteLine("Hello") writer.Close()
Create a file and write "Hello" to it
Delete a file
Throw an exception
Do nothing
Q72
Q72 How do you read all text from a file in VB.NET?
File.ReadAllText("file.txt")
StreamReader.ReadFile("file.txt")
File.ReadFile("file.txt")
File.GetText("file.txt")
Q73
Q73 What will the following code output?
If File.Exists("test.txt") Then Console.WriteLine("File exists") Else Console.WriteLine("File does not exist")
File exists
File does not exist
Error
No output
Q74
Q74 Identify the error in the following code:
Dim reader As New StreamReader("nonexistent.txt") Console.WriteLine(reader.ReadToEnd()) reader.Close()
File not found
Error opening file
Stream is null
No error
Q75
Q75 What happens if a file is locked by another process while being accessed in VB.NET?
Throws an IOException
Skips the file
Accesses the file
Deletes the file
Q76
Q76 How can you ensure a file stream is closed properly, even if an exception occurs?
Use Try-Catch
Use the Finally block
Use Using statement
Use File.Close() explicitly
Q77
Q77 A StreamWriter is writing data to a file but fails intermittently. What could be the issue?
File permissions are insufficient
File is read-only
Disk space is low
Buffer is not flushed
Q78
Q78 What is a collection in VB.NET?
A type of array
A class used to store and manage multiple items
A data type
A control
Q79
Q79 Which class in VB.NET is used for a dynamic array?
ArrayList
List
Dictionary
HashSet
Q80
Q80 What is the primary advantage of using generics in VB.NET?
Better performance
Type safety
Simplified code
Memory management
Q81
Q81 Which namespace provides classes for collections in VB.NET?
System.Collections
System.Collections.Generic
System.Collections.Concurrent
All of these
Q82
Q82 What is the difference between List and ArrayList in VB.NET?
List is fixed size
ArrayList is type-safe
List is generic and type-safe
ArrayList does not exist
Q83
Q83 Which of the following is the correct way to declare a generic List in VB.NET?
Dim numbers As New List(Of Integer)
Dim numbers As New ArrayList(Of Integer)
Dim numbers As List(Integer)
Dim numbers As New HashSet(Of Integer)
Q84
Q84 What will the following code output?
Dim names As New List(Of String) names.Add("Alice") names.Add("Bob") Console.WriteLine(names.Count)
1
2
0
Error
Q85
Q85 What is the correct way to iterate through a Dictionary in VB.NET?
For Each key As String In dict.Keys Console.WriteLine(key) Next
For i As Integer = 0 To dict.Count
For Each item As String In dict
None of these
Q86
Q86 What is the output of the following code?
Dim numbers As New HashSet(Of Integer) numbers.Add(1) numbers.Add(1) Console.WriteLine(numbers.Count)
1
2
Error
0
Q87
Q87 What happens if you try to add a duplicate key to a Dictionary in VB.NET?
Throws an ArgumentException
Replaces the existing key
Throws a runtime error
Allows duplicate keys
Q88
Q88 How can you handle a situation where a key is missing in a Dictionary?
Check using the ContainsKey method
Try-Catch block
Direct access
Using a default key
Q89
Q89 Why might a ConcurrentDictionary be used instead of a regular Dictionary?
To handle type mismatches
To handle concurrency issues in multithreaded environments
To add duplicate keys
To improve performance
Q90
Q90 What is ADO.NET primarily used for in VB.NET?
Reading and writing files
Accessing and managing databases
Handling exceptions
Creating user interfaces