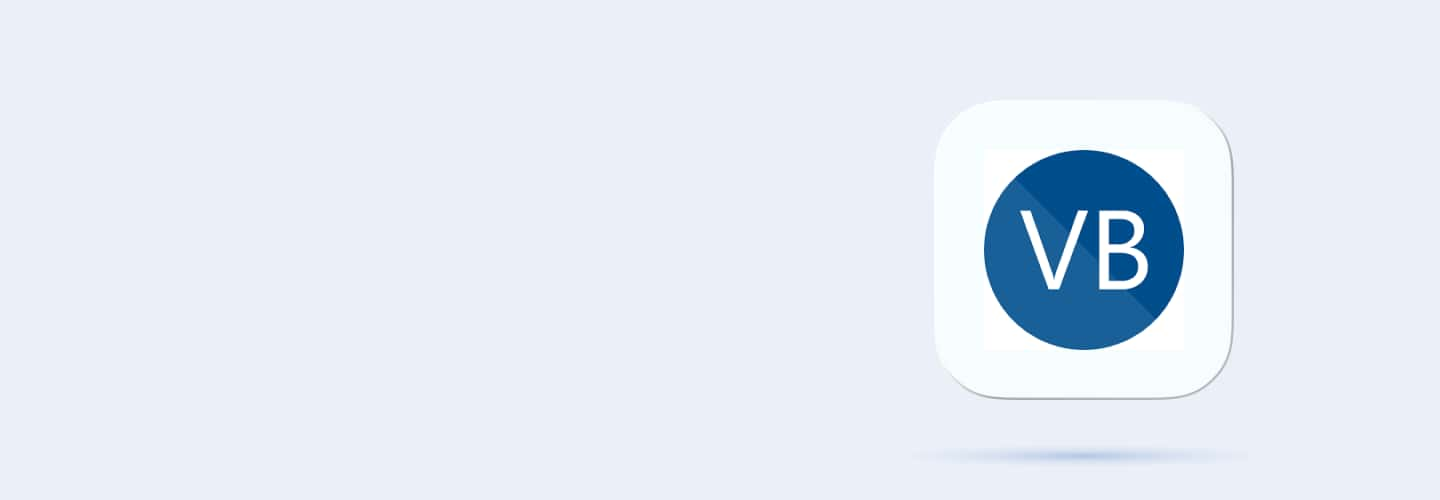
Q31
Q31 What is the purpose of the If statement in VB.NET?
Iteration
Decision-making
Variable declaration
Error handling
Q32
Q32 Which control structure in VB.NET is used for repetitive tasks?
If
For
Select Case
Try-Catch
Q33
Q33 What is the default keyword in the Select Case statement used for?
To handle errors
To define the loop limit
To specify the default case
To initialize variables
Q34
Q34 What is the purpose of the Exit For statement?
To exit a For loop immediately
To pause a For loop
To restart a For loop
To ignore errors
Q35
Q35 Which loop in VB.NET is guaranteed to execute at least once?
For
While
Do-While
Select Case
Q36
Q36 How do you exit a While loop in VB.NET?
Use Continue While
Use Exit While
Use End While
Use Stop
Q37
Q37 What will the following code output?
Dim x As Integer = 5 If x > 3 Then Console.WriteLine("Greater") Else Console.WriteLine("Smaller")
Greater
Smaller
Error
No output
Q38
Q38 What will the following For loop output?
For i As Integer = 1 To 3 Console.WriteLine(i) Next
123
321
12
Error
Q39
Q39 What will the following code output?
Select Case 5 Case 1 Console.WriteLine("One") Case 5 Console.WriteLine("Five") Case Else Console.WriteLine("Other") End Select
One
Five
Other
Error
Q40
Q40 A For loop in VB.NET runs infinitely. What could be the issue?
Condition never becomes False
Improper initialization
Incorrect step value
Incorrect loop declaration
Q41
Q41 How can you break out of a nested loop in VB.NET?
Use Continue
Use Exit For
Use End
Restart the loop
Q42
Q42 In debugging, how can you identify which iteration of a loop caused an error?
By using the debugger
By printing the iteration value
By adding a breakpoint
By checking iteration logs
Q43
Q43 What is a class in VB.NET?
A data structure
A blueprint for creating objects
A loop construct
A method
Q44
Q44 What does the keyword "Inherits" do in VB.NET?
Creates a new class
Extends a base class
Implements an interface
Declares an abstract class
Q45
Q45 Which feature of OOP allows the same method name to behave differently based on the input?
Encapsulation
Inheritance
Polymorphism
Abstraction
Q46
Q46 What is encapsulation in VB.NET?
Hiding implementation details and exposing functionality
Creating multiple classes
Reusing code
None of these
Q47
Q47 What is an abstract class in VB.NET?
A class with only static methods
A class that must be inherited
A class without methods
A sealed class
Q48
Q48 What is the correct way to define a property in a VB.NET class?
Property MyProperty As Integer
Dim MyProperty As Property
Public Property MyProperty As Integer
Declare Property MyProperty As Integer
Q49
Q49 What is the output of the following code?
Public Class MyClass Public Function GetValue() As Integer Return 10 End Function End Class Dim obj As New MyClass Console.WriteLine(obj.GetValue())
10
0
Error
Null
Q50
Q50 How do you call the base class constructor from a derived class in VB.NET?
MyBase.New
Base
Super
Inherited
Q51
Q51 What is the correct way to implement an interface in VB.NET?
Class MyClass Implements IInterface
Class MyClass Inherits IInterface
Class MyClass Extends IInterface
Class MyClass Includes IInterface
Q52
Q52 What happens if you try to instantiate an abstract class in VB.NET?
Syntax Error
Runtime Error
No Error
The program crashes
Q53
Q53 How can you determine if a method is overriding a base class method in VB.NET?
Check for the "Overridable" keyword
Check for the "Overrides" keyword
Check for the "Inherits" keyword
Check for the "MyBase" keyword
Q54
Q54 While debugging, you notice a derived class method is not called. What could be the reason?
The method is not marked as Overridable in the base class
The method is not marked as Overloads in the derived class
The method is Private in the base class
The derived class does not inherit the base class
Q55
Q55 What is the primary purpose of exception handling in VB.NET?
To prevent runtime errors
To debug code
To gracefully handle runtime errors
To compile the program
Q56
Q56 Which VB.NET keyword is used to throw an exception?
Raise
Throw
Catch
Try
Q57
Q57 What is the purpose of the Finally block in a Try-Catch statement?
To execute code only if no exception occurs
To execute code only if an exception occurs
To execute code regardless of exceptions
To handle specific exceptions
Q58
Q58 Which of the following is a common exception in VB.NET?
FormatException
NullPointerException
SegmentationFault
DivideByZeroException
Q59
Q59 How can you create a custom exception in VB.NET?
By inheriting from the System.Exception class
By overriding the base exception class
By implementing a Try-Catch
By using the ExceptionHandler class
Q60
Q60 What will the following code output?
Try Console.WriteLine(10 / 0) Catch ex As DivideByZeroException Console.WriteLine("Error") End Try
Error
0
Exception
No Output