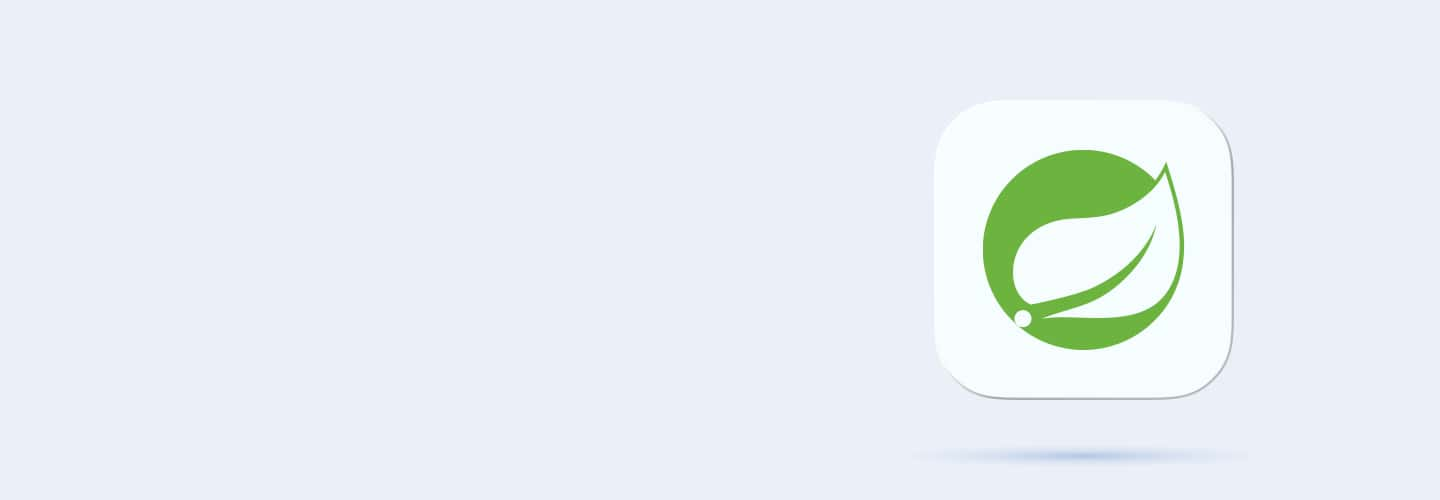
Q91
Q91 An application throws "NoClassDefFoundError" during startup. What is the likely cause?
Missing dependency
Incorrect classpath configuration
Corrupted JAR file
All of the above
Q92
Q92 What is the primary goal of Spring Security?
To provide authentication and authorization
To handle database transactions
To secure network communication
To provide MVC support
Q93
Q93 Which annotation is used to enable Spring Security configuration?
@EnableWebSecurity
@EnableSecurity
@EnableAuthorization
@EnableConfiguration
Q94
Q94 What is the default password storage mechanism in Spring Security?
MD5
SHA-256
BCrypt
Plain Text
Q95
Q95 How can you secure a REST API endpoint in Spring Security?
Use @Secure annotation
Use @PreAuthorize annotation
Use URL-based security
All of the above
Q96
Q96 How does Spring Security handle CSRF (Cross-Site Request Forgery)?
Enables by default
Disables by default
Requires custom filter
Not supported
Q97
Q97 How can you define a custom UserDetailsService in Spring Security?
Implement the UserDetailsService interface
Use the @Service annotation
Extend the UserDetails class
Use the AuthenticationManager interface
Q98
Q98 How can you configure HTTP security in Spring Security?
Use HttpSecurity in a configuration class
Use XML configuration
Use the @Secure annotation
Use the @Configuration annotation
Q99
Q99 An application throws "AccessDeniedException" in Spring Security. What is the likely cause?
Incorrect role configuration
Authentication failure
Improper password hashing
Invalid session configuration
Q100
Q100 Why does a "BadCredentialsException" occur in Spring Security?
Invalid username
Invalid password
Incorrect authentication provider configuration
All of the above
Q101
Q101 An application fails with "AuthenticationCredentialsNotFoundException". What could be the issue?
Missing authentication filter
Improper authentication manager configuration
No security context set
Any of the above
Q102
Q102 Which annotation is used to define a RESTful web service in Spring?
@RestController
@Controller
@RequestMapping
@Service
Q103
Q103 How does Spring handle serialization for REST responses?
Using ObjectMapper
Using SerializationUtils
Using custom serializers
Using built-in converters
Q104
Q104 What is the role of @ResponseBody in Spring REST?
Converts Java object to HTTP response body
Defines REST endpoints
Maps URLs to controllers
Handles dependency injection
Q105
Q105 How does Spring support content negotiation in REST APIs?
Using @RestController
Using MediaType class
Using @ResponseBody
Using Accept header
Q106
Q106 How can you define a GET endpoint in Spring REST?
Use @PostMapping
Use @PutMapping
Use @GetMapping
Use @DeleteMapping
Q107
Q107 How do you return a custom HTTP status in a Spring REST controller?
Use ResponseEntity
Use HttpHeaders
Use @ResponseBody
Use RestTemplate
Q108
Q108 How can you add query parameters to a REST endpoint in Spring?
Use @PathVariable
Use @RequestParam
Use @ModelAttribute
Use @RequestBody
Q109
Q109 Why does a "HttpMessageNotReadableException" occur in Spring REST?
Missing @RequestBody
Unsupported media type
Malformed JSON request
Improper dependency injection
Q110
Q110 An application fails with "HttpMediaTypeNotAcceptableException". What could be the issue?
Unsupported response media type
Invalid Accept header
Missing content negotiation strategy
All of the above
Q111
Q111 Which Spring module is typically used for unit testing?
Spring Test module
Spring Core module
Spring ORM module
Spring MVC module
Q112
Q112 How does Spring enable integration testing?
By providing in-memory database support
By offering testing annotations
By enabling mock injection
By using all these methods
Q113
Q113 What is the role of @ContextConfiguration in Spring tests?
Configures application context
Defines transactional boundaries
Sets up mock Beans
Enables AOP
Q114
Q114 Which annotation is used for transactional tests in Spring?
@Transactional
@TransactionTest
@IntegrationTest
@ContextConfiguration
Q115
Q115 How can you mock a Bean in Spring integration tests?
Use @MockBean annotation
Use @Mock annotation
Use @MockConfiguration
Use @BeanDefinition
Q116
Q116 How can you load a specific application context configuration in a test?
Use @ContextConfiguration annotation
Use @SpringConfiguration annotation
Use @Configuration annotation
Use @SpringTest annotation
Q117
Q117 How do you assert that a REST endpoint returns the correct status code?
Use MockMvc.perform()
Use ResponseEntity assertions
Use HttpClient assertions
Use RestTemplate
Q118
Q118 Why does a "BeanCreationException" occur in Spring tests?
Circular dependency
Missing Bean definition
Improper test configuration
All of the above
Q119
Q119 An integration test fails with "TransactionRollbackException". What is the likely cause?
Improper transactional boundary
Database constraint violation
Missing test configuration
Invalid mock data
Q120
Q120 What is the purpose of Spring Cloud?
To manage microservices
To secure web applications
To simplify testing
To enhance MVC features