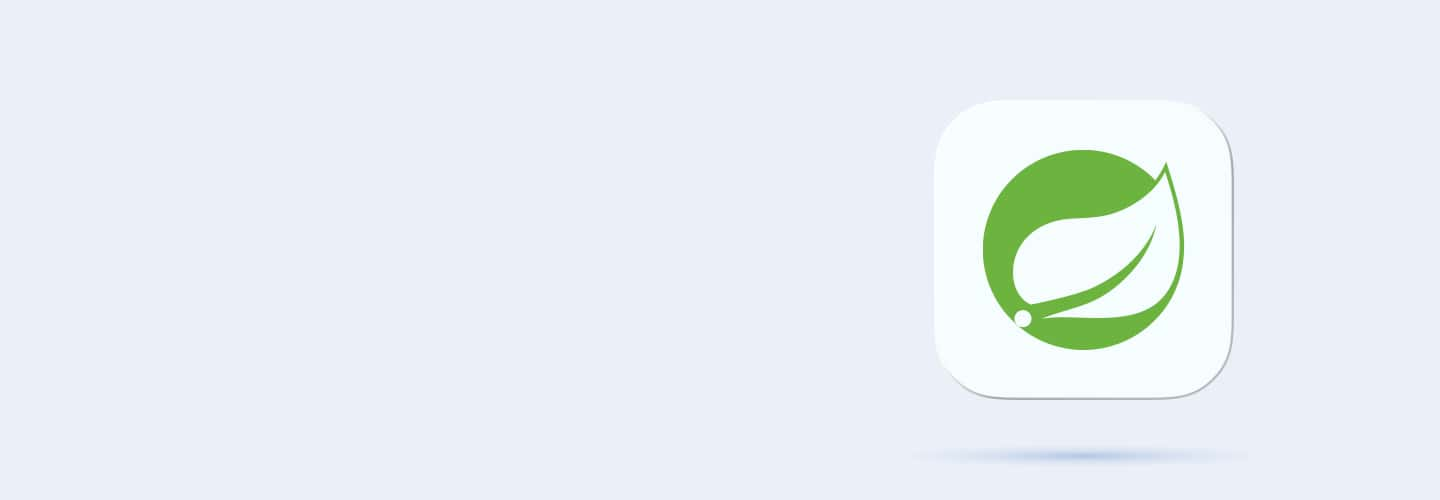
Q61
Q61 How can you configure a DataSource Bean in Spring?
Using @DataSource annotation
Using @Bean annotation in a configuration class
Using XML configuration
Using @Repository annotation
Q62
Q62 How do you define a repository interface in Spring Data JPA?
Extend the JpaRepository interface
Implement the JpaRepository interface
Extend the Repository class
Annotate it with @Transactional
Q63
Q63 How can you execute a named query in Spring Data JPA?
Use @Query annotation
Use EntityManager's createQuery method
Define a query in XML
Use @Transaction
Q64
Q64 How can you implement batch processing in Spring JDBC?
Use JdbcTemplate's batchUpdate method
Use @Batch annotation
Use @Transaction annotation
Use SQL scripts
Q65
Q65 An application fails with "DataIntegrityViolationException". What could be the cause?
Circular dependency
Database constraint violation
Incorrect Bean definition
Improper transaction management
Q66
Q66 Why does a "LazyInitializationException" occur in Spring Data JPA?
EntityManager closed prematurely
Incorrect database configuration
Improper dependency injection
Incorrect query syntax
Q67
Q67 An application throws "TransactionSystemException". What should you check?
Transaction timeout settings
Incorrect repository configuration
Database connection pool size
All of the above
Q68
Q68 What does MVC stand for in Spring?
Model View Controller
Model View Configuration
Model Value Controller
Mapping View Configuration
Q69
Q69 Which component of Spring MVC handles incoming HTTP requests?
DispatcherServlet
HandlerMapping
ViewResolver
ModelAndView
Q70
Q70 What is the role of ViewResolver in Spring MVC?
Resolves HTTP requests
Handles dependency injection
Maps views to names
Manages session state
Q71
Q71 How does Spring MVC handle form submissions?
By using @Controller
By mapping @RequestMapping
By using @ModelAttribute
By using all three
Q72
Q72 Which annotation is used to define a RESTful controller in Spring MVC?
@RestController
@Controller
@RequestMapping
@Service
Q73
Q73 How can you map a URL to a method in Spring MVC?
Use @Controller
Use @RestController
Use @RequestMapping
Use @Service
Q74
Q74 How do you pass data from a controller to a view in Spring MVC?
Use ModelAndView object
Use HttpServletResponse
Use DispatcherServlet
Use RestTemplate
Q75
Q75 How can you enable Spring MVC configuration in a Java-based configuration?
Use @EnableWebMvc annotation
Use @Configuration annotation
Use @Controller annotation
Use @RequestMapping annotation
Q76
Q76 How do you handle exceptions globally in Spring MVC?
Use @ExceptionHandler annotation
Use @ControllerAdvice annotation
Use global exception configuration
Use any one of the above
Q77
Q77 An application throws "404 Not Found" in Spring MVC. What is the likely cause?
Incorrect view name
Handler method not mapped
Invalid controller configuration
Missing @Controller annotation
Q78
Q78 Why does a "HttpMediaTypeNotSupportedException" occur in Spring MVC?
Unsupported content type
Missing view configuration
Invalid HTTP method
Incorrect controller mapping
Q79
Q79 An application fails with "NullPointerException" in a controller method. What could be the issue?
Missing @RequestParam annotation
Improper dependency injection
Invalid Bean configuration
All of the above
Q80
Q80 What is the purpose of Spring Boot?
To replace Spring Framework
To simplify Spring configuration
To handle database transactions
To build mobile apps
Q81
Q81 Which annotation is used to specify a Spring Boot application entry point?
@Configuration
@SpringBootApplication
@Component
@RestController
Q82
Q82 How does Spring Boot manage dependencies?
Using a custom Maven plugin
Using a built-in dependency manager
Using Maven's dependency scope
Using Spring Framework
Q83
Q83 What is the purpose of the application.properties file in Spring Boot?
To store application configuration
To store Bean definitions
To handle HTTP requests
To define security rules
Q84
Q84 What is the purpose of the @EnableAutoConfiguration annotation?
To enable custom configurations
To disable default configurations
To allow Spring Boot to guess configurations
To set application properties
Q85
Q85 How can you run a Spring Boot application from the command line?
Use the java command
Use mvn spring-boot:run
Use Gradle bootRun
Use mvn spring-run
Q86
Q86 How can you specify a custom port for a Spring Boot application?
Use server.port in application.properties
Use server.port in application.yml
Pass as a command-line argument
All of the above
Q87
Q87 How can you include additional libraries in a Spring Boot project?
Add to the classpath
Add to application.properties
Include in Maven's dependencies
Include in src/main/resources
Q88
Q88 How can you create a REST endpoint in Spring Boot?
Use @RestController annotation
Use @Component annotation
Use @RequestMapping annotation
Use @Service annotation
Q89
Q89 Why does a Spring Boot application fail to start with "Port already in use"?
Duplicate Bean definition
Invalid application.properties file
Another process is using the port
Dependency injection issue
Q90
Q90 Why does a "BeanCreationException" occur in Spring Boot?
Missing Bean configuration
Invalid dependency injection
Circular dependencies
All of the above