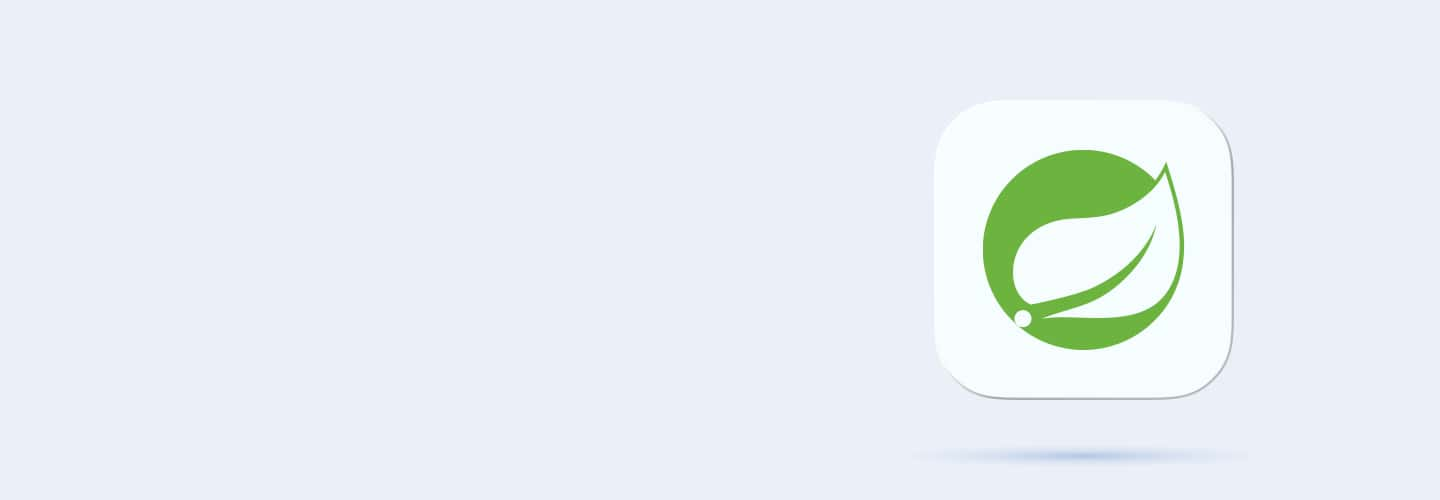
Q31
Q31 An application fails with "UnsatisfiedDependencyException". What should you check first?
Missing Bean definitions
Misconfigured dependencies
Presence of circular dependencies
Incorrect property file configuration
Q32
Q32 What is the purpose of a Spring Bean?
To represent a data entity
To define the scope of an object
To create reusable Java components
To initialize an application context
Q33
Q33 Which scope is used for a Bean that needs to be shared across all HTTP requests?
Singleton
Prototype
Request
Session
Q34
Q34 Which method is called when a Bean is initialized in Spring?
afterPropertiesSet
postConstruct
init
initialize
Q35
Q35 How can you define a Bean’s lifecycle in Spring?
Using init-method and destroy-method
Using annotations like @PostConstruct and @PreDestroy
Using XML configuration
Using a BeanPostProcessor
Q36
Q36 What is the default scope of a Bean in Spring?
Singleton
Prototype
Request
GlobalSession
Q37
Q37 What is the purpose of the BeanPostProcessor interface in Spring?
To provide callbacks before and after Bean initialization
To configure dependency injection
To manage application events
To handle exceptions during Bean creation
Q38
Q38 How can you explicitly declare a Bean in Spring using Java configuration?
Use @Bean annotation
Use @Configuration annotation
Use @Component annotation
Use @Service annotation
Q39
Q39 How do you define a prototype-scoped Bean in XML configuration?
<bean scope="singleton">
<bean scope="prototype">
<bean scope="request">
<bean scope="session">
Q40
Q40 How can you customize the initialization behavior of a Spring Bean?
Implement the InitializingBean interface
Annotate methods with @PostConstruct
Specify an init-method in XML
All of the above
Q41
Q41 An application fails with "BeanDefinitionStoreException". What is the likely cause?
Circular dependencies
Improper Bean definition in XML
Incorrect dependency injection
Improper use of annotations
Q42
Q42 Why does a "BeanInitializationException" occur during application startup?
Bean definition not found
Error in Bean initialization callback
Dependency injection failure
All of the above
Q43
Q43 An application throws "NoUniqueBeanDefinitionException". What is the best way to resolve this issue?
Use @Primary annotation
Use @Qualifier annotation
Rename conflicting Beans
Remove duplicate Beans
Q44
Q44 What does AOP stand for in Spring?
Application Oriented Programming
Aspect-Oriented Programming
Advanced Object Programming
Asynchronous Oriented Programming
Q45
Q45 Which Spring module supports AOP?
Spring Core
Spring AOP
Spring ORM
Spring MVC
Q46
Q46 What is a "pointcut" in Spring AOP?
A specific advice
A collection of advices
A predicate that matches join points
A method in a Bean
Q47
Q47 What is the purpose of "advice" in Spring AOP?
To encapsulate cross-cutting concerns
To replace dependency injection
To define Bean lifecycle
To create Bean definitions
Q48
Q48 Which advice type runs after a join point completes?
Before advice
After returning advice
Around advice
After throwing advice
Q49
Q49 Which annotation is used to declare a method as advice in Spring AOP?
@Advice
@Pointcut
@Aspect
@Around
Q50
Q50 How do you define a pointcut in Spring using annotations?
Use @Aspect
Use @Pointcut
Use @Around
Use @Advice
Q51
Q51 How can you enable AOP in Spring configuration?
Add @EnableAspectJAutoProxy annotation
Use @Aspect annotation
Use @Around annotation
Declare a pointcut in XML
Q52
Q52 How can you apply advice only to specific methods in a class?
Use method-level annotations
Define specific pointcut expressions
Apply Bean-level configuration
Use XML-based configuration
Q53
Q53 An application throws "NoSuchMethodException" during AOP execution. What could be the cause?
Incorrect pointcut expression
Missing Bean definition
Improper dependency injection
Circular dependency
Q54
Q54 Why does a "ProxyCreationException" occur in Spring AOP?
Incorrect AOP proxy configuration
Missing @Aspect annotation
Improper dependency injection
Invalid Bean scope
Q55
Q55 An application fails with "IllegalArgumentException" during advice execution. What should you check?
Pointcut expressions
Advice method parameters
Dependency injection
All of the above
Q56
Q56 What is the primary goal of Spring JDBC?
To provide low-level JDBC APIs
To simplify database interaction
To create database schemas
To manage transactions
Q57
Q57 What is an ORM tool commonly used with Spring?
Hibernate
JDBC
MySQL
PostgreSQL
Q58
Q58 Which annotation is used to declare a transactional method in Spring?
@Transaction
@Transactional
@Repository
@Service
Q59
Q59 What is the role of JPA in Spring Data Access?
To manage database transactions
To simplify object-relational mapping
To perform dependency injection
To manage MVC components
Q60
Q60 How does Spring manage database transactions?
Using AOP-based proxies
Using custom SQL queries
Using Bean lifecycle methods
Using JPA annotations