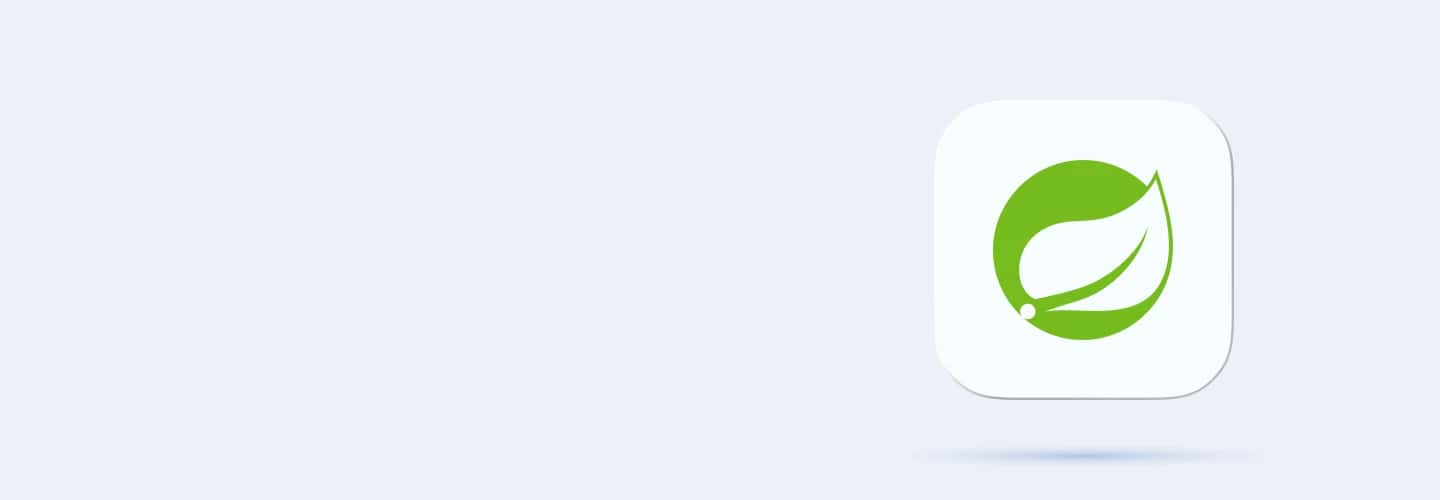
Q121
Q121 What is the primary purpose of Aspect-Oriented Programming (AOP) in Spring?
To manage transactions
To modularize cross-cutting concerns
To handle dependency injection
To improve database performance
Q122
Q122 Which annotation in Spring is used to define an aspect?
@Aspect
@Transactional
@Pointcut
@Around
Q123
Q123 Which Spring AOP advice type is used to execute logic before a method runs?
@After
@Before
@Around
@Pointcut
Q124
Q124 What is a Pointcut in Spring AOP?
A method that handles exceptions
A way to specify where an advice should apply
A method for configuring beans
A feature for database transactions
Q125
Q125 Which annotation is used in Spring to define a transaction boundary?
@Transactional
@TransactionManager
@Transaction
@Commit
Q126
Q126 How can you define a custom Pointcut expression in Spring AOP?
@Pointcut("execution(* com.example.service..(..))")
@Before("com.example.service.")
@Around("execution(...*(..))")
@Aspect("execution(..)")
Q127
Q127 How do you apply a transaction to a specific method in Spring?
Annotate the method with @Transactional
Call the method inside a try-catch block
Define the method in application.properties
Use @Commit annotation
Q128
Q128 A transactional method is not rolling back after an exception. What could be the issue?
The exception is not a RuntimeException
@Transactional is not applied
The database connection is closed
The method is private
Q129
Q129 A Spring AOP advice is not executing as expected. What could be the issue?
The aspect class is not annotated with @Aspect
The advice method is private
The pointcut expression is incorrect
All of the above
Q130
Q130 Which caching mechanism is commonly used in Spring MVC to improve performance?
Session caching
Request caching
EhCache
SSL caching
Q131
Q131 What is the primary benefit of using lazy loading in Spring MVC applications?
Reduces memory consumption
Improves database security
Increases processing time
Enhances UI rendering
Q132
Q132 Which of the following is NOT a recommended approach for optimizing database performance in Spring MVC?
Using connection pooling
Using indexes on frequently queried columns
Executing queries within loops
Implementing caching
Q133
Q133 Which annotation enables caching in a Spring MVC application?
@EnableCaching
@Cacheable
@CacheConfig
@EnableTransactionManagement
Q134
Q134 How can you limit the number of records fetched from the database in a Spring JPA query?
Using @Query with LIMIT
Using @Size annotation
Using @Restrict
Configuring application.properties
Q135
Q135 Which Spring Boot property helps reduce application startup time?
spring.main.lazy-initialization=true
spring.optimize.startup=true
spring.lazy.init.enabled
spring.performance.boost=true
Q136
Q136 A Spring MVC application is experiencing slow response times. What could be a potential cause?
Excessive database queries
Using an optimized connection pool
Properly configured caching
Minimized JavaScript and CSS
Q137
Q137 A Spring application is consuming excessive memory. What could be the cause?
Inefficient garbage collection
Using lazy initialization
Proper connection pooling
Minimized API calls
Q138
Q138 Which testing framework is commonly used for unit testing in Spring MVC?
JUnit
Mockito
TestNG
JUnit and Mockito
Q139
Q139 What is the primary purpose of mocking in unit tests?
To create real database connections
To simulate dependencies and isolate tests
To execute performance tests
To integrate external services
Q140
Q140 Which of the following is NOT a benefit of unit testing in Spring MVC?
Improves code maintainability
Reduces debugging time
Eliminates the need for integration testing
Ensures correctness of individual components
Q141
Q141 Which annotation is used to enable Spring context for unit tests?
@SpringBootTest
@MockBean
@TestConfiguration
@ContextConfiguration
Q142
Q142 How do you mock a Spring Bean in a unit test?
Use @Mock
Use @InjectMocks
Use @MockBean
Use @Test
Q143
Q143 A unit test fails because the application context is not loading. What could be the issue?
Missing @SpringBootTest annotation
Incorrect dependency injection
Incorrect test data
Missing dependencies
Q144
Q144 A mocked dependency is returning null in a unit test. What could be the cause?
The mock is not properly initialized
The real implementation is being used instead of the mock
@MockBean is missing
The test method is private
Q145
Q145 What is a common challenge when developing large-scale Spring MVC applications?
Managing dependencies
Configuring security
Handling performance bottlenecks
Implementing logging
Q146
Q146 Which design pattern is commonly used in Spring MVC applications to handle service layer logic?
Singleton
Factory
Service Layer
Decorator
Q147
Q147 What is a key consideration when implementing RESTful APIs in a Spring MVC application?
Ensuring proper exception handling
Using efficient query strategies
Optimizing response serialization
Managing concurrency
Q148
Q148 Which method in Spring MVC is used for handling file uploads in a web application?
uploadFile()
handleMultipartFile()
processFile()
storeFile()
Q149
Q149 A Spring MVC application is experiencing slow response times due to excessive database calls. What is the recommended solution?
Implement caching
Increase the server RAM
Reduce the number of controllers
Disable security
Q150
Q150 A Spring MVC application is facing memory leaks. What could be the cause?
Excessive use of Singleton beans
Unclosed database connections
Circular dependencies in beans
Misconfigured caching