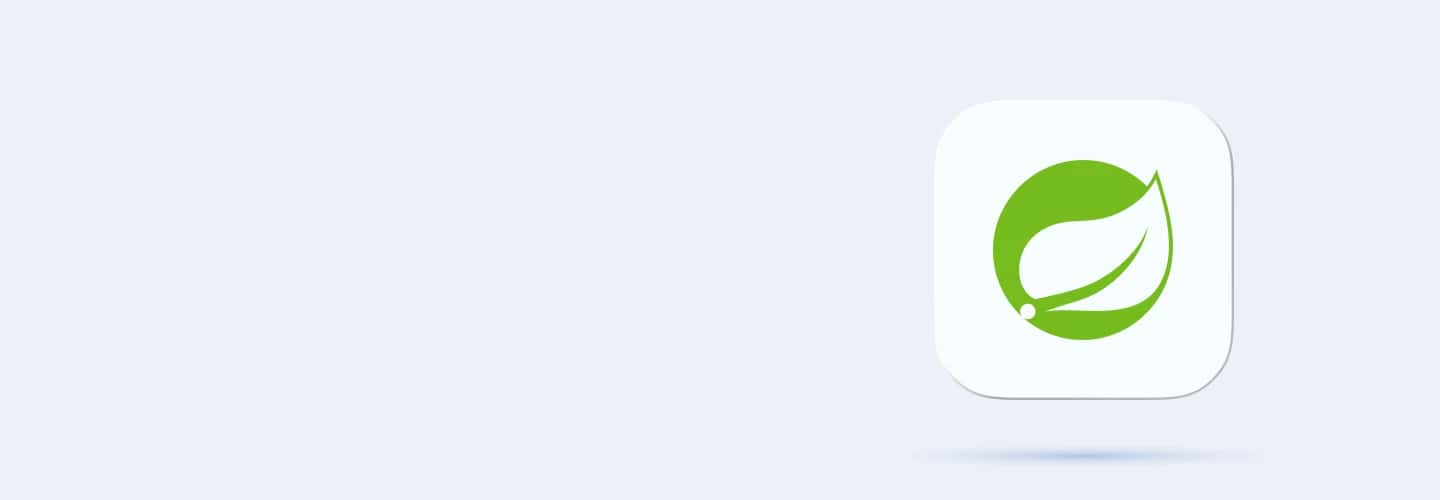
Q61
Q61 Which configuration is required for enabling Spring MVC's form validation?
Enable component scanning
Add a Validator bean and use @Valid
Use @Autowired in the Controller
Define a custom ViewResolver
Q62
Q62 Which method is used to bind form attributes in Spring MVC?
model.addObject()
model.addAttribute()
request.getAttribute()
response.setAttribute()
Q63
Q63 How do you specify a default value for a request parameter in @RequestParam?
@RequestParam("id", defaultValue="1")
@RequestParam(value="id", default="1")
@RequestParam(value="id", required=false)
@RequestParam("id")
Q64
Q64 How can you handle multiple form submissions in Spring MVC?
Using multiple controller methods with @RequestMapping
Using @SessionAttributes
Using a single method with @ModelAttribute
Using @Component annotation
Q65
Q65 A form submission in Spring MVC is not binding data to the model. What is the likely reason?
The controller method does not have @ModelAttribute
The form action URL is incorrect
The view is missing a submit button
The model object is not annotated with @Entity
Q66
Q66 A required request parameter is missing, causing an error. How can this be handled?
Use a try-catch block
Use @RequestParam with required=false
Redirect to an error page
Use @RequestBody instead
Q67
Q67 A developer is facing an issue where form validation is not working in Spring MVC. What could be the reason?
The controller method is missing @Valid
The form has incorrect input types
The form is missing a submit button
The application is using an outdated Spring version
Q68
Q68 Which of the following is a view technology supported by Spring MVC?
JSP
Thymeleaf
Freemarker
All of these
Q69
Q69 What is the role of a ViewResolver in Spring MVC?
To map logical view names to actual view templates
To handle HTTP requests
To validate user input
To manage session attributes
Q70
Q70 Which statement about JSP and Thymeleaf is correct?
JSP is an HTML-based template engine
Thymeleaf is a Spring-integrated template engine
JSP requires a servlet container to run
Both B and C
Q71
Q71 Which is a major advantage of using Thymeleaf over JSP in Spring MVC?
Thymeleaf can be used as a standalone template engine
Thymeleaf does not require a servlet container
JSP has better integration with Spring MVC
JSP has more security features
Q72
Q72 Which of the following is NOT a valid way to pass data from a Spring MVC controller to a JSP view?
Using Model object
Using HttpSession
Using JavaScript
Using ModelAndView
Q73
Q73 Which expression is used in JSP to display the value of a request attribute?
${requestScope.value}
${sessionScope.value}
${value}
${param.value}
Q74
Q74 How do you reference a model attribute in a Thymeleaf template?
${model.attribute}
#{attribute}
${attribute}
@{attribute}
Q75
Q75 How can you create a hyperlink in a Thymeleaf template that dynamically maps to a Spring MVC controller?
<a href='/home'>Home</a>
<a th:href='@{/home}'>Home</a>
<a th:ref='/home'>Home</a>
<a link='home'>Home</a>
Q76
Q76 Which tag is used in JSP to include another JSP file dynamically?
jsp:import
jsp:include
jsp:forward
jsp:invoke
Q77
Q77 A JSP page is not rendering correctly, showing raw EL expressions like ${attribute}. What could be the issue?
The EL syntax is incorrect
The view resolver is misconfigured
Expression Language (EL) is disabled
The controller is missing @RequestMapping
Q78
Q78 A Thymeleaf template is not displaying dynamic data. What could be the reason?
The controller is not returning a Model attribute
The view resolver is not configured
The template file is missing
The application is not deployed correctly
Q79
Q79 A developer is getting a 'Template not found' error in Thymeleaf. What is the most likely cause?
The template path is incorrect
The model attribute is missing
Thymeleaf dependency is missing
The database connection is incorrect
Q80
Q80 Which annotation is used in Spring MVC to handle exceptions at the controller level?
@RequestMapping
@ExceptionHandler
@ControllerAdvice
@RestController
Q81
Q81 What is the purpose of @ControllerAdvice in Spring MVC?
To provide global exception handling for controllers
To handle security authentication
To resolve views
To inject dependencies
Q82
Q82 Which interface in Spring MVC can be used for customized exception handling?
HandlerInterceptor
HandlerExceptionResolver
ControllerAdvice
DispatcherServlet
Q83
Q83 What happens when an exception occurs in a Spring MVC controller that is not explicitly handled?
The request is forwarded to the default error page
Spring MVC returns a 500 error response
The request is silently ignored
The request is retried automatically
Q84
Q84 Which of the following is NOT a recommended approach for handling exceptions in Spring MVC?
Using @ExceptionHandler methods
Defining a global exception handler with @ControllerAdvice
Throwing unchecked exceptions without handling
Implementing HandlerExceptionResolver
Q85
Q85 How do you define an exception handler for NullPointerException in a Spring MVC controller?
@ExceptionHandler(NullPointerException.class)
@ControllerAdvice(NullPointerException.class)
@ErrorHandler(NullPointerException.class)
@HandleException(NullPointerException.class)
Q86
Q86 How can you return a custom error response from an exception handler in Spring MVC?
Returning a String as a view name
Returning a ResponseEntity object
Throwing the exception again
Returning null
Q87
Q87 Which method in HandlerExceptionResolver is used to resolve exceptions?
resolveException()
handleException()
processException()
mapException
Q88
Q88 How can you configure a custom error page in Spring MVC?
Using @ExceptionHandler
Using @ErrorPage
Defining an entry in web.xml
Creating a Controller with an error endpoint
Q89
Q89 A Spring MVC application is showing a generic 500 error page instead of a custom error message. What could be the issue?
The controller does not have @ExceptionHandler
@ControllerAdvice is missing
The custom error page is not configured properly
The database is down
Q90
Q90 A controller-specific exception handler is not catching exceptions. What could be the issue?
The method is missing @ExceptionHandler
The exception type does not match
The method is private
All of the above