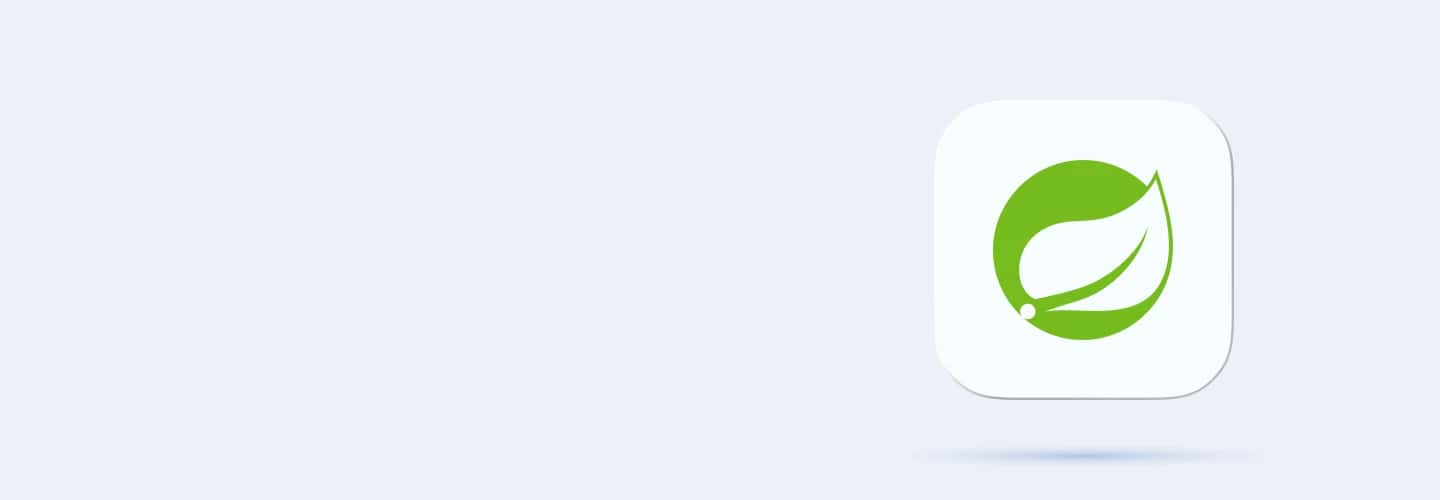
Q31
Q31 A controller method is not being called even though the URL mapping is correct. What could be the issue?
DispatcherServlet is missing
Incorrect HTTP method used in the request
Database connection issue
Application is not deployed properly
Q32
Q32 What is the purpose of the @RequestMapping annotation in Spring MVC?
To map HTTP requests to controller methods
To define beans in the Spring container
To handle database transactions
To manage view resolution
Q33
Q33 Which attribute of @RequestMapping is used to specify the HTTP method?
value
method
path
produces
Q34
Q34 What happens if a request does not match any @RequestMapping method?
It is ignored
Spring MVC returns a 404 error
Spring MVC redirects to the default controller
Spring MVC executes the first controller method
Q35
Q35 How do you define multiple URL mappings for a single controller method?
Use multiple @RequestMapping annotations
Provide multiple values inside a single @RequestMapping annotation
Define separate controller methods
Use a wildcard (*) in URL mapping
Q36
Q36 What is the difference between @RequestMapping and @GetMapping?
@GetMapping is specific to GET requests, while @RequestMapping supports multiple HTTP methods
Both are identical
@RequestMapping is specific to GET requests, while @GetMapping supports multiple HTTP methods
@GetMapping is used for form handling only
Q37
Q37 Which annotation is used to map dynamic path variables in Spring MVC?
@RequestParam
@PathVariable
@ModelAttribute
@RequestBody
Q38
Q38 How do you define a method that handles HTTP POST requests?
@PostMapping("/submit")
@RequestMapping(value="/submit", method=RequestMethod.POST)
Both A and B
@GetMapping("/submit")
Q39
Q39 How do you pass a query parameter in a GET request?
/users?id=1
/users/1
/users?id==1
/users?id-1
Q40
Q40 What is the correct way to extract query parameters in Spring MVC?
@PathVariable("id") int id
@RequestParam("id") int id
@ModelAttribute("id") int id
@RequestBody int id
Q41
Q41 A request is returning a 405 (Method Not Allowed) error. What is the likely cause?
The URL mapping is incorrect
The request is using an unsupported HTTP method
The database is down
The controller is missing
Q42
Q42 A controller method using @RequestMapping is not being invoked. What could be the issue?
The method is private
The URL pattern does not match
The controller is not annotated with @RestController
All of the above
Q43
Q43 A developer needs to handle both GET and POST requests in the same method. How can this be achieved?
Use two different methods
Use multiple @RequestMapping annotations with different methods
Use @RequestMapping with method={RequestMethod.GET, RequestMethod.POST}
It is not possible
Q44
Q44 What is the primary role of the Model in the MVC pattern?
To handle user interactions
To store and manage data
To control the view rendering
To map HTTP requests to methods
Q45
Q45 Which component in MVC is responsible for displaying data to the user?
Model
View
Controller
DispatcherServlet
Q46
Q46 Which component in MVC handles user inputs and updates the Model?
Model
View
Controller
Service
Q47
Q47 Which of the following best describes the MVC pattern?
A software pattern that separates concerns
A database management technique
A cloud computing model
A UI rendering framework
Q48
Q48 What is the main benefit of using the MVC pattern in Spring applications?
It allows for better scalability and maintenance
It improves database performance
It makes the application load faster
It eliminates the need for front-end code
Q49
Q49 In Spring MVC, how does the Controller communicate with the View?
By modifying the database
By returning a View object
By using Model and ViewResolver
By calling JavaScript functions
Q50
Q50 Which method is commonly used in a controller to add data to the Model?
setModel()
addAttribute()
setAttribute()
setView()
Q51
Q51 How do you return a View name from a Spring MVC controller method?
return "index.jsp";
return new ModelAndView("index");
return "index";
return View("index");
Q52
Q52 Which class in Spring MVC is responsible for resolving the logical view name to an actual view?
ModelAndView
ViewResolver
DispatcherServlet
RequestMapping
Q53
Q53 A developer is facing an issue where the model attributes are not available in the View. What could be the reason?
The controller is missing @RequestMapping
The model attributes were not added using addAttribute()
The database is not connected
The view file is missing
Q54
Q54 A Spring MVC application is rendering a blank page instead of the expected View. What could be the issue?
The ViewResolver is not configured correctly
The Model is empty
The Controller does not return a View name
The View file is missing
Q55
Q55 A developer is receiving a '404 Not Found' error when trying to access a View in Spring MVC. What could be the cause?
Incorrect View name returned from Controller
ViewResolver is not configured properly
The requested View file does not exist
Incorrect request mapping
Q56
Q56 Which annotation in Spring MVC is used to bind form data to a model object?
@RequestParam
@ModelAttribute
@RequestBody
@PathVariable
Q57
Q57 What is the primary advantage of using @ModelAttribute in Spring MVC?
It simplifies form data binding
It improves database transactions
It enhances security
It manages session attributes
Q58
Q58 Which Spring MVC annotation is used to retrieve query parameters from a request?
@PathVariable
@RequestParam
@ModelAttribute
@RequestMapping
Q59
Q59 How does Spring MVC handle form validation?
Using custom JavaScript functions
Using @Valid and BindingResult
Using @Autowired
Using @PathVariable
Q60
Q60 Which object in Spring MVC is used to store validation errors from form submissions?
Model
BindingResult
Session
ResponseEntity