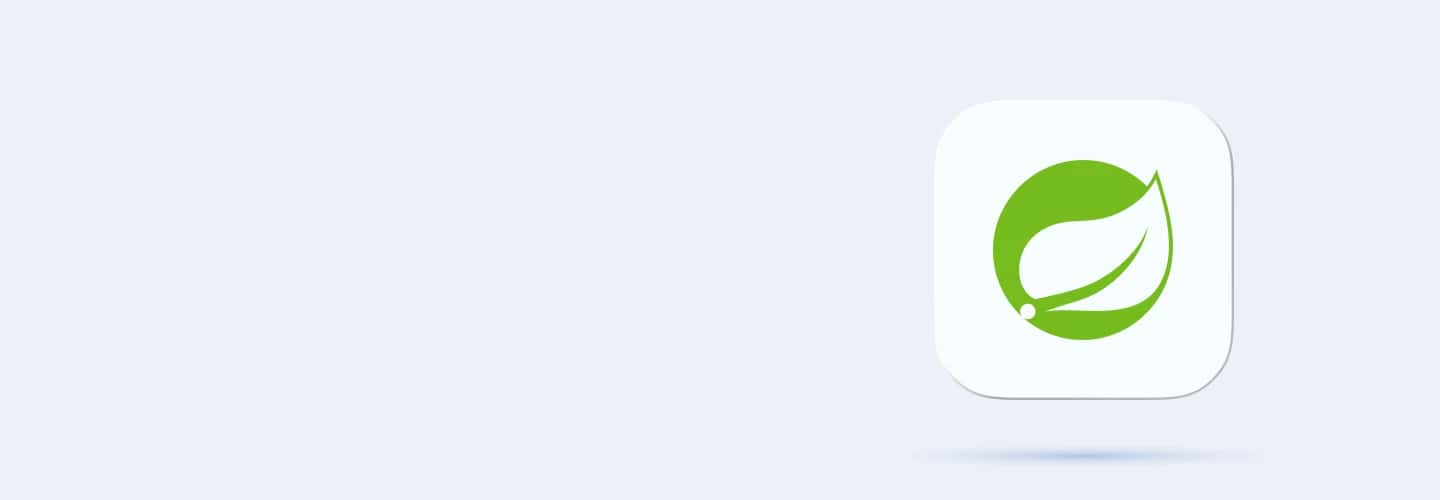
Q1
Q1 What is the primary purpose of Spring MVC in a web application?
To manage database transactions
To handle client-side JavaScript code
To implement the Model-View-Controller pattern for web applications
To manage dependency injection in an application
Q2
Q2 Which module of the Spring Framework provides support for Spring MVC?
Spring JDBC
Spring ORM
Spring Web
Spring Security
Q3
Q3 Which design pattern does Spring MVC follow?
Singleton
Factory
Model-View-Controller
Decorator
Q4
Q4 Which of the following is NOT a key component of Spring MVC?
DispatcherServlet
ModelAndView
SessionFactory
ViewResolver
Q5
Q5 What is the role of DispatcherServlet in Spring MVC?
It handles database transactions
It dispatches requests to appropriate controllers
It is responsible for managing sessions
It compiles Java code into bytecode
Q6
Q6 Which interface does DispatcherServlet implement?
Servlet
HttpServlet
GenericServlet
ServletContextListener
Q7
Q7 In Spring MVC, what is the default URL mapping of DispatcherServlet?
/
/dispatcher/
/.mvc
/*
Q8
Q8 Which annotation in Spring MVC is used to define a controller class?
@Service
@RestController
@Controller
@Component
Q9
Q9 A Spring MVC application is returning a '404 Not Found' error when accessing a URL. What is the most likely cause?
The controller method handling the request is missing the @RequestMapping annotation.
The database connection is misconfigured.
The @Service annotation is missing in the service layer.
The application is not running on the correct Java version.
Q10
Q10 What is the role of the DispatcherServlet in Spring MVC?
To manage the database connections
To act as the front controller
To perform dependency injection
To handle authentication
Q11
Q11 Which component in Spring MVC is responsible for resolving views?
ModelAndView
ViewResolver
ViewHandler
ResponseMapper
Q12
Q12 In Spring MVC, where is the request first received?
Model
ViewResolver
Controller
DispatcherServlet
Q13
Q13 Which component in Spring MVC handles request mapping?
DispatcherServlet
HandlerMapping
ViewResolver
Model
Q14
Q14 Which component in Spring MVC handles user inputs and binds them to objects?
HandlerMapping
Model
DataBinder
ViewResolver
Q15
Q15 What happens after DispatcherServlet receives a request in Spring MVC?
It directly renders the view
It routes the request to the appropriate controller using HandlerMapping
It executes the database query
It validates the request headers
Q16
Q16 What is the main function of HandlerAdapter in Spring MVC?
To handle HTTP requests
To execute the appropriate controller method
To perform security validation
To manage view resolution
Q17
Q17 Which of the following best describes the Model in Spring MVC?
A template engine
A way to pass data between the controller and the view
A database entity
A part of the front-end framework
Q18
Q18 Which method in Spring MVC is typically used to add model attributes?
setAttribute()
addObject()
addAttribute()
setModel()
Q19
Q19 A developer is facing an issue where the correct view is not being resolved in a Spring MVC application. What could be the most likely reason?
Incorrect HandlerMapping configuration
ViewResolver is not properly configured
DispatcherServlet is missing
Controller class is missing
Q20
Q20 What is the main responsibility of a controller in Spring MVC?
Handling business logic
Managing database transactions
Handling HTTP requests and returning responses
Rendering views
Q21
Q21 Which annotation is used to handle form submissions in Spring MVC?
@ModelAttribute
@RequestBody
@RequestParam
@ResponseBody
Q22
Q22 Which annotation is used to handle HTTP GET requests in a Spring MVC controller?
@RequestMapping
@GetMapping
@PostMapping
@PutMapping
Q23
Q23 Which return type is commonly used in a Spring MVC controller method to return a view?
void
ModelAndView
HttpServletResponse
String
Q24
Q24 What is the purpose of the @RequestParam annotation in Spring MVC?
To inject a service into the controller
To map request parameters to method arguments
To handle session management
To return a JSON response
Q25
Q25 Which annotation is used to handle exceptions in a Spring MVC controller?
@ErrorHandler
@ExceptionHandler
@ErrorController
@RestController
Q26
Q26 What is the default scope of a Spring MVC controller?
Prototype
Session
Singleton
Request
Q27
Q27 How do you define a request mapping for the URL '/home' in a Spring MVC controller?
@RequestMapping("/home")
@RequestMapping(path="/home")
@GetMapping("/home")
All of the above
Q28
Q28 How do you pass data from a Spring MVC controller to a view using the Model?
model.set("key", value)
model.add("key", value)
model.addAttribute("key", value)
model.put("key", value)
Q29
Q29 A developer is getting a 400 Bad Request error when submitting a form in Spring MVC. What is the most likely cause?
Incorrect database query
Missing @RequestParam annotation
Incorrect model binding
Application is not running
Q30
Q30 A Spring MVC application is returning a '500 Internal Server Error'. What could be the most probable cause?
A missing controller class
An exception occurring in the controller
A missing DispatcherServlet
A misconfigured web.xml