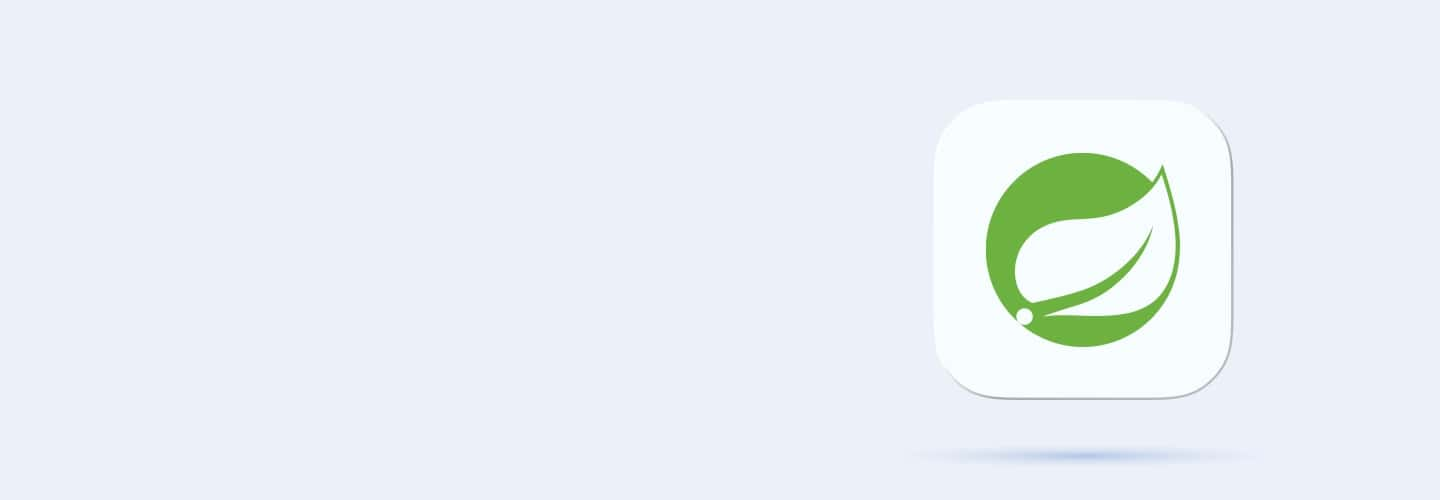
Q121
Q121 What command is used to build a Spring Boot application using Maven for deployment?
mvn package
mvn compile
mvn install
mvn deploy
Q122
Q122 How can you specify the active Spring profile in a deployed application?
Using an environment variable
Modifying the application.properties directly
Hard-coding in the application
Using the Spring dashboard
Q123
Q123 What is the purpose of using a CI/CD pipeline in the context of deploying Spring Boot applications?
To ensure that the application is free from bugs.
To automate the deployment process.
To manage the application's users.
To optimize the application's performance.
Q124
Q124 What common issue should be checked first if a Spring Boot application fails immediately after deployment?
Incorrect application properties
Server compatibility issues
Network failures
Insufficient server resources
Q125
Q125 How can you address a failure in a Spring Boot application when it starts but is inaccessible externally?
Check firewall settings and network configuration
Increase the logging level
Decrease application load
Replicate the environment locally
Q126
Q126 What is the primary role of Apache Kafka in a Spring Boot application?
To provide database capabilities
To serve as a messaging system
To handle external API calls
To manage application security
Q127
Q127 How does Kafka's partitioning feature enhance message processing in Spring Boot applications?
By allowing concurrent processing of messages
By encrypting messages
By storing messages permanently
By validating message integrity
Q128
Q128 What is the purpose of a Kafka Consumer Group in the context of Spring Boot integration?
To group similar consumers for load balancing
To provide fault tolerance
To prioritize message consumption
To segregate consumer data
Q129
Q129 How does Kafka ensure data durability and high availability in Spring Boot applications?
By replicating data across multiple nodes
By using a single active node for data storage
By encrypting all stored data
By using cloud storage exclusively
Q130
Q130 How can you configure a Kafka Producer in a Spring Boot application to send messages asynchronously?
By using the @Async annotation on the producer method
By setting enable.idempotence=true in the producer configuration
By specifying linger.ms greater than zero
By calling the send() method with a callback
Q131
Q131 What is the advantage of enabling idempotence in a Kafka Producer configuration within a Spring Boot application?
To ensure messages are only processed once, even if sent multiple times
To increase the speed of message delivery
To encrypt messages
To automatically compress messages
Q132
Q132 What should you check if messages are not being received by a Kafka Consumer in a Spring Boot application?
The consumer's group configuration
The network connectivity
The topic subscription details
Incorrect consumer settings
Q133
Q133 How can you troubleshoot issues related to message order in a Kafka stream consumed by a Spring Boot application?
By ensuring all messages are sent to the same partition
By increasing the number of consumers
By enabling message encryption
By using a different message key for each message
Q134
Q134 Which tool is commonly used in Spring Boot for real-time application monitoring?
Nagios
Prometheus
Splunk
New Relic
Q135
Q135 What is the significance of Micrometer in the context of Spring Boot applications?
It serves as an application optimizer.
It is a metrics integration tool.
It enhances code quality.
It manages application configurations.
Q136
Q136 How does Spring Boot utilize the Actuator /metrics endpoint?
To configure application properties
To monitor application health and performance metrics
To manage application security
To handle incoming requests
Q137
Q137 How do custom metrics enhance monitoring in Spring Boot?
By allowing personalized security protocols
By providing detailed user analytics
By tailoring monitoring to specific application needs
By reducing data redundancy
Q138
Q138 What is the purpose of using the @Timed annotation in Spring Boot applications?
To measure the response time of methods
To synchronize method executions
To delay method execution
To time database transactions
Q139
Q139 How can you implement custom metrics in a Spring Boot application using Micrometer?
By using the Counter and Gauge classes from Micrometer
By manually logging metrics data
By using the Spring Boot CLI
By using JMX tools
Q140
Q140 What initial step should be taken when a monitored metric shows unexpected spikes in a Spring Boot application?
Reviewing the application logs for errors
Increasing server resources
Changing the metric collection interval
Restarting the server
Q141
Q141 How can the issue of missing metrics data be resolved in a Spring Boot application?
Ensuring correct Micrometer setup
Expanding the metrics storage capacity
Reducing the metrics collection frequency
Upgrading the application framework
Q142
Q142 What is a recommended practice for managing application configurations in Spring Boot?
Using hard-coded values in the code
Using external configuration files
Storing configurations in the database
Embedding configurations in the application
Q143
Q143 Why is it important to keep your Spring Boot dependencies up-to-date?
To increase the application size
To improve security and performance
To make the application slower
To complicate dependency management
Q144
Q144 What is the benefit of using Spring Boot's devtools?
To speed up the development process by enabling automatic restart and live reload
To enhance application security
To compress the application code
To manage application dependencies
Q145
Q145 How can Spring Boot help in improving the security of an application?
By enforcing strong password policies
By providing ready-to-use security configurations
By encrypting all data
By tracking user behavior
Q146
Q146 What is the advantage of integrating Spring Boot with a CI/CD pipeline?
To decrease code quality
To automate the build and deployment processes
To reduce developer collaboration
To increase development costs
Q147
Q147 What annotation is used in Spring Boot to schedule a task to run at fixed intervals?
@Scheduled
@Interval
@FixedRate
@Timer
Q148
Q148 What is a best practice when creating RESTful APIs in Spring Boot?
Using only GET requests for all operations
Using meaningful HTTP verbs and URIs
Using non-standard HTTP status codes
Ignoring statelessness
Q149
Q149 How can you effectively manage exceptions in a Spring Boot application?
By ignoring all exceptions
By using a global exception handler
By only logging exceptions to the console
By sending all exceptions to the client
Q150
Q150 What approach should be taken to optimize the performance of a Spring Boot application experiencing slow load times?
Adding more hardware resources
Increasing the number of application instances
Profiling and identifying bottlenecks
Reducing the amount of logging