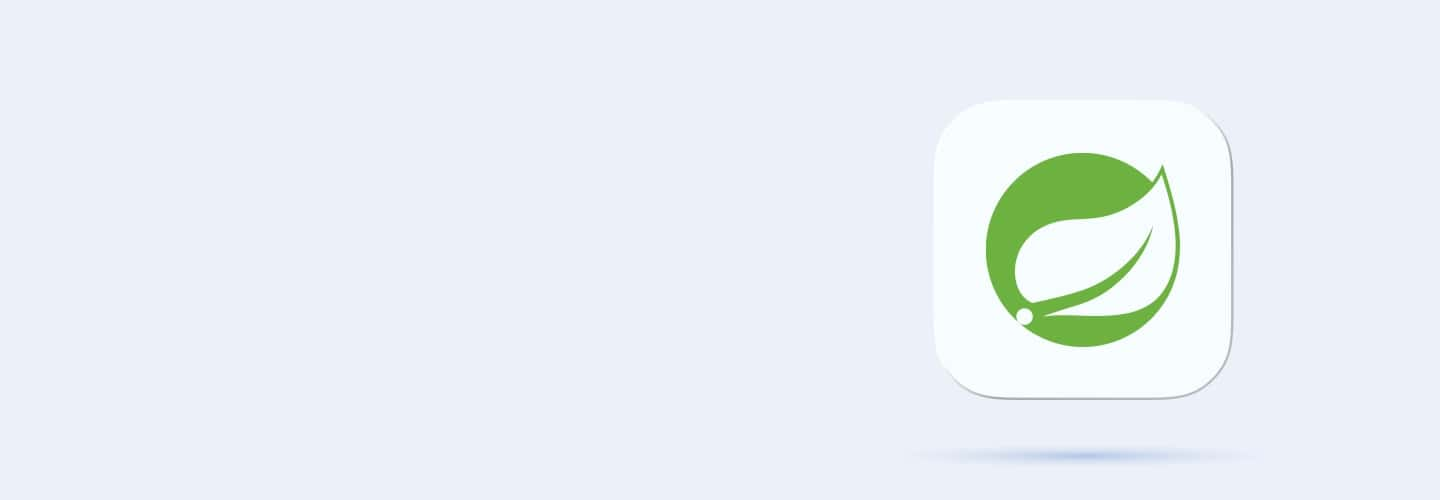
Q91
Q91 What challenge can arise when multiple Spring Boot applications are containerized and run on the same host?
Port conflicts
Memory leaks
Slow boot times
Version incompatibility
Q92
Q92 What is a best practice for managing application configurations in a containerized Spring Boot environment?
Storing configurations inside the container
Using external configuration files
Hard-coding configurations in the application
Using default configurations
Q93
Q93 How do you specify a base image in a Dockerfile for a Spring Boot application?
Using the FROM directive
Using the BASE directive
Using the IMAGE directive
Using the SOURCE directive
Q94
Q94 Which command in a Dockerfile is used to copy the Spring Boot application jar into the Docker image?
COPY
ADD
PASTE
INSERT
Q95
Q95 How can you optimize the build process of a Docker image for a Spring Boot application to reduce build time and size?
Using multi-stage builds
Using a single layer for all commands
Using uncompressed layers
Using larger base images
Q96
Q96 What is a common issue when a Spring Boot application in a Docker container fails to connect to external services?
Incorrect network settings
Incompatible software versions
Unsupported database drivers
Faulty application code
Q97
Q97 How can you troubleshoot a Spring Boot application that starts in a Docker container but is not accessible externally?
Checking the Docker container logs
Verifying the container's network settings
Ensuring the correct application port is exposed
All of the above
Q98
Q98 What does the Spring WebFlux framework in Spring Boot facilitate?
Building synchronous, blocking web apps
Building reactive, non-blocking web apps
Automated testing of web apps
Building microservices
Q99
Q99 In reactive programming with Spring Boot, what role does the Project Reactor play?
It provides a reactive library for implementing reactive APIs
It is a build tool
It is a dependency management system
It handles only synchronous operations
Q100
Q100 How does backpressure control the flow of data in reactive programming?
By speeding up data flow
By limiting data flow based on consumer capacity
By encrypting data
By automatically storing excess data in the cloud
Q101
Q101 What is the main advantage of using reactive programming for data streams in Spring Boot?
Increased security of data transmissions
Simplification of code
Ability to handle large numbers of concurrent data streams efficiently
Reduced memory usage
Q102
Q102 How do you define a Mono object that returns a single value asynchronously in Spring Boot's WebFlux?
Using Mono.just()
Using Mono.create()
Using Mono.single()
Using Mono.async()
Q103
Q103 What method can be used to combine multiple reactive streams in Spring Boot?
Flux.merge()
Flux.combine()
Flux.join()
Flux.zip()
Q104
Q104 Which operator in Project Reactor is used to handle errors in a reactive stream?
onErrorReturn()
onErrorContinue()
onErrorStop()
onErrorHandle()
Q105
Q105 When debugging a non-responsive reactive API in Spring Boot,
what is an initial step to identify the issue?
Checking the network connections
Verifying the reactive chain for blocking calls
Increasing server resources
Reducing the number of API calls
Q106
Q106 How can you trace and diagnose issues in a Spring Boot application using reactive programming?
Utilizing traditional debugging techniques
Incorporating logging and tracing frameworks like Sleuth
Using system-level monitoring tools
All of the above
Q107
Q107 What is the purpose of using @Profile annotation in Spring Boot?
To inject dependencies conditionally
To integrate third-party services
To enable conditional configuration based on the environment
To create asynchronous methods
Q108
Q108 How does the @Conditional annotation enhance Spring Boot's configuration capabilities?
By allowing beans to be loaded based on specific conditions
By automatically configuring beans
By enhancing the security of the application
By optimizing performance
Q109
Q109 In what way does externalized configuration benefit a Spring Boot application?
It allows configurations to be changed at runtime without redeployment
It simplifies the codebase
It speeds up the boot process
It reduces memory usage
Q110
Q110 What role does Spring Cloud Config play in managing Spring Boot application configurations?
It serves as a central server for managing external properties across all environments
It locally caches application properties
It encrypts application configurations
It manages version control for properties
Q111
Q111 How do you specify a property source in a Spring Boot application if the properties file is not named application.properties?
By using the @PropertySource annotation
By modifying the spring.config.name property
By using the @Value annotation
By renaming the properties file
Q112
Q112 How can you dynamically switch between different data sources in a Spring Boot application based on a specific condition?
By using @Primary annotation on data source beans
By using @Conditional on data source configurations
By creating multiple application.properties files
By manually switching the bean in the context
Q113
Q113 What is a method for applying fine-grained traffic routing rules in a Spring Boot application when using microservices?
Using Spring MVC controllers
Using Netflix Zuul as an API gateway
Using Spring Data REST
Using the @RequestMapping annotation
Q114
Q114 What should you check first if your @Conditional bean does not load in a Spring Boot application?
Bean definition conflicts
Environmental properties
The condition's logic
Classpath issues
Q115
Q115 How can you resolve issues related to external configuration properties not being recognized by a Spring Boot application?
Check the property file's location and format
Increase logging level to debug
Rebuild the application
Change the Spring Boot version
Q116
Q116 Which deployment option is natively supported by Spring Boot for web applications?
Deploying as a WAR to an external Tomcat server
Deploying as a standalone JAR
Deploying using FTP
Deploying via SSH
Q117
Q117 What is a benefit of using containerization with Docker for deploying Spring Boot applications?
Enhanced security features
Scalability and easy version control
Reduced memory usage
Automated data backups
Q118
Q118 What does the Spring Boot Maven plugin provide for application deployment?
It provides version management.
It automates the application deployment process.
It manages database migrations.
It optimizes performance.
Q119
Q119 Which feature does Spring Boot provide to simplify the deployment in cloud environments?
Automatic scaling
Built-in cloud-native features
Automatic resource allocation
All of the above
Q120
Q120 How does the Actuator 'health' endpoint assist in the deployment of Spring Boot applications?
It provides continuous integration support.
It monitors the health and performance of the application post-deployment.
It manages application scaling.
It enhances security protocols.