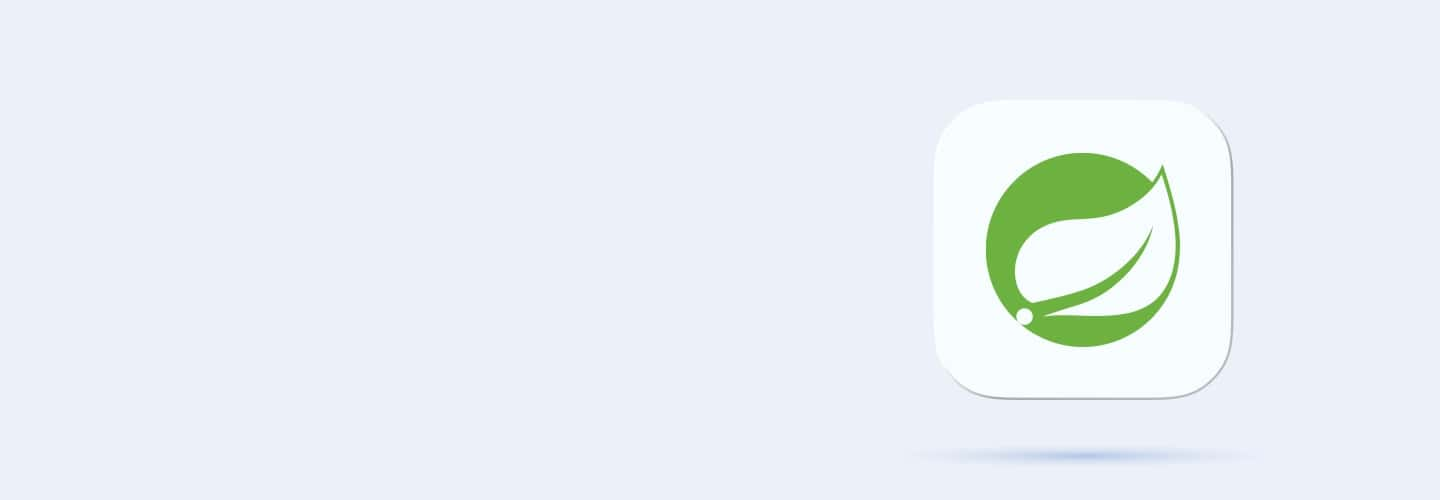
Q31
Q31 How can you handle exceptions in a Spring Boot REST API to return a custom JSON error response?
Using @ControllerAdvice
Using @ExceptionHandler
Using @ErrorController
Using all of the above
Q32
Q32 What is the best practice to secure a REST API in Spring Boot?
Using HTTPS
Using Basic Auth
Using OAuth2
Using API keys
Q33
Q33 A developer is encountering a 404 Not Found error when accessing a REST endpoint in Spring Boot.
What is the likely cause?
Incorrect URL in the request
Server not running
Endpoint not defined
Firewall blocking access
Q34
Q34 If a REST API method in Spring Boot is unexpectedly returning 200 OK with an empty body,
what might be the issue?
The method is not annotated correctly
The data source is returning null
The method's return type is void
All of the above
Q35
Q35 How can you trace a request through a Spring Boot application to debug issues with REST API calls?
Using Spring Boot Actuator
Using application logs
Using @Trace
Using both Spring Boot Actuator and application logs
Q36
Q36 Which Spring Boot starter is used for integrating Spring Data JPA into an application?
spring-boot-starter-jdbc
spring-boot-starter-data-jpa
spring-boot-starter-data-mongodb
spring-boot-starter-data-rest
Q37
Q37 What does the @Transactional annotation do in a Spring Boot application?
Manages the scope of a single database transaction
Applies a filter to a query
Optimizes query performance
Provides security for database operations
Q38
Q38 How can you enable lazy loading of associations in Spring Data JPA?
By using the @Lazy annotation
By setting fetch = FetchType.LAZY on associations
By using the @Basic(fetch = FetchType.LAZY) annotation
By configuring it in the application.properties file
Q39
Q39 What role does the JdbcTemplate play in Spring Boot?
It provides a way to access NoSQL databases
It simplifies JDBC operations by handling boilerplate code
It manages JPA entities
It serves as a template for RESTful services
Q40
Q40 How does Spring Boot support database migrations?
Through JDBC template
Through Spring Data REST
Through the use of JPA entities
Through integration with tools like Flyway or Liquibase
Q41
Q41 Which annotation would you use to execute a custom query with Spring Data JPA repository?
@Query
@Select
@SQL
@DatabaseQuery
Q42
Q42 In Spring Data, how can you dynamically construct queries using method names in your repository interface?
By naming methods according to the properties of the entity classes
By using the @NamedMethod annotation
By using special keywords in method names
By annotating methods with @GenerateQuery
Q43
Q43 What is the purpose of the @EnableJpaRepositories annotation in a Spring Boot application?
To enable support for JPA repositories
To configure JPA entities
To activate JPA query logging
To initialize the JPA EntityManager factory
Q44
Q44 What common issue might cause a DataIntegrityViolationException in a Spring Boot application using JPA?
Incorrect data types
Missing required fields
Constraint violations
All of the above
Q45
Q45 If a Spring Boot application fails to connect to the database,
what could be potential issues to check?
Incorrect database URL or credentials in properties file
Network issues
Incorrect driver configuration
All of the above
Q46
Q46 How can you resolve a LazyInitializationException in a Spring Boot application using Hibernate?
By ensuring the session remains open during the access of lazily loaded properties
By marking all associations as EAGER
By using the @Transactional annotation during data access
All of the above
Q47
Q47 Which module in Spring Boot provides support for securing web applications?
Spring Security
Spring Web
Spring MVC
Spring Data
Q48
Q48 What is the primary use of the @PreAuthorize annotation in Spring Security?
To define SQL queries
To restrict access to methods based on authority
To validate user inputs
To configure method-level security settings
Q49
Q49 How does Spring Boot automatically secure web applications?
By using basic authentication by default
By enforcing HTTPS
By integrating with OAuth2
By applying role-based access control
Q50
Q50 In Spring Security, what is the function of the WebSecurityConfigurerAdapter?
It is used for SQL database integration
It customizes web-based security
It manages REST API authentication
It handles form-based security
Q51
Q51 Which annotation is used to enable Spring Security’s web security support in a Spring Boot application?
@EnableWebSecurity
@EnableWebMvc
@EnableTransactionManagement
@EnableSecurity
Q52
Q52 How can you configure a custom login page in Spring Boot using Spring Security?
By overriding the default security configuration in WebSecurityConfigurerAdapter
By using the @AuthenticationPrincipal annotation
By using a custom UserDetailsService implementation
By annotating a controller with @LoginController
Q53
Q53 What is the role of the UserDetailsService interface in Spring Security?
Managing user permissions
Retrieving user-specific data
Managing user sessions
Customizing user authentication
Q54
Q54 If Spring Security is blocking access to a resource that should be public,
what is a common solution?
Adjusting the order of security rules in the configuration
Disabling Spring Security
Removing the security dependency
Rewriting the application logic
Q55
Q55 How can you debug issues with role-based access controls not working as expected in Spring Boot?
Reviewing the roles assigned in the database
Checking the security configuration in WebSecurityConfigurerAdapter
Ensuring the @PreAuthorize annotations are set correctly
Reviewing user session settings
Q56
Q56 What should you check if a user's authentication is failing in a Spring Boot application secured with Spring Security?
The user's credentials in the data source
The encryption algorithm used
The security configuration in WebSecurityConfigurerAdapter
The network settings
Q57
Q57 What annotation is primarily used to indicate a class is a Spring Boot test class?
@SpringBootTest
@Test
@WebMvcTest
@DataJpaTest
Q58
Q58 Which annotation is used to mock a specific bean in the Spring application context during testing?
@MockBean
@Mock
@Autowired
@InjectMock
Q59
Q59 What is the purpose of the @WebMvcTest annotation in Spring Boot testing?
It tests the entire application
It tests individual web layers only
It tests data layers only
It tests security layers only
Q60
Q60 How does Spring Boot simplify integration testing with databases?
By using the @DatabaseTest annotation
By automatically configuring in-memory databases
By isolating the web layer during tests
By using JDBC templates