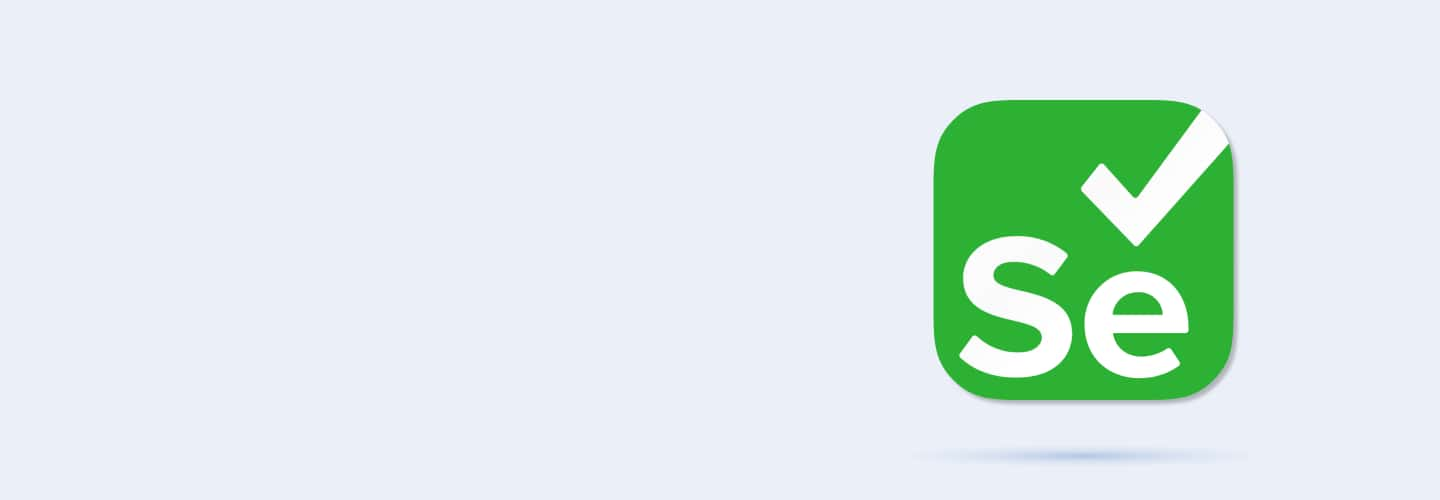
Q61
Q61 Which method in Selenium is used to switch to a new browser window?
driver.switchTo().window()
driver.switchTo().tab()
driver.getWindowHandle()
driver.selectWindow()
Q62
Q62 How can you check if a checkbox is selected in Selenium WebDriver?
isChecked()
isSelected()
isClicked()
getValue()
Q63
Q63 Which Selenium command is used to retrieve the attribute value of a web element?
element.getText()
element.getValue()
element.getAttribute()
element.getProperties()
Q64
Q64 What is the purpose of the driver.switchTo().frame() method?
To close the frame
To move focus to a specific frame
To maximize the frame
To reload the frame
Q65
Q65 How can you interact with hidden elements in Selenium?
Use JavaScript Executor
Direct click
Clear text
Use getText()
Q66
Q66 How do you handle browser alerts in Selenium WebDriver?
driver.alert().accept()
driver.switchTo().alert().accept()
driver.alertAccept()
driver.confirmAlert()
Q67
Q67 Which method is used to select an option by visible text in a dropdown list?
selectByValue()
selectByIndex()
selectByVisibleText()
selectText()
Q68
Q68 In Selenium WebDriver, how do you simulate pressing the Enter key?
sendKeys(Keys.ENTER)
driver.click(Keys.Enter)
driver.type(Keys.Enter)
pressEnter()
Q69
Q69 How can you perform a double-click action on an element in Selenium WebDriver?
action.doubleClick(element)
element.doubleClick()
element.click(2)
driver.doubleClick()
Q70
Q70 A test fails with a "NoAlertPresentException" error. What could be the cause?
Alert is not displayed
Network connection issue
Invalid URL
Alert is maximized
Q71
Q71 A script fails when trying to select an option from a dropdown. What could be a likely solution?
Use click() method instead
Verify dropdown is loaded
Restart the browser
Update browser version
Q72
Q72 A Selenium test encounters an "ElementNotInteractableException." What is a common cause?
Element is hidden or off-screen
Test case has a typo
Incorrect Selenium version
Network speed is high
Q73
Q73 What is the main purpose of waits in Selenium?
To speed up script execution
To handle page load times
To reduce code lines
To automatically close the browser
Q74
Q74 Which type of wait applies to all elements and is set once in Selenium WebDriver?
Explicit Wait
Thread.sleep
Implicit Wait
Fluent Wait
Q75
Q75 What is the advantage of using Explicit Wait over Implicit Wait?
It’s faster
It allows waiting for specific conditions
It applies to all elements
It doesn’t require imports
Q76
Q76 Which of the following conditions can be used with WebDriverWait?
Element to be editable
Element to load
Browser to close
Page title to update
Q77
Q77 In which scenario would Fluent Wait be preferred over Explicit Wait?
When wait conditions are static
For short load times
When polling is needed
For global application
Q78
Q78 How do you set an Implicit Wait of 10 seconds in Selenium?
driver.manage().timeouts().implicitWait(10)
driver.wait(10)
driver.setWait(10)
driver.setImplicitWait(10)
Q79
Q79 How would you apply an Explicit Wait until an element is clickable in Selenium?
WebDriverWait(driver,10).until(element.isClickable())
WebDriverWait(driver,10).until(EC.element_to_be_clickable(locator))
driver.waitClickable(10)
EC.clickable(driver,10)
Q80
Q80 Which method in Fluent Wait allows setting an exception to ignore during polling?
withIgnoreException()
ignoring()
pollException()
waitWithException()
Q81
Q81 A script fails due to a "TimeoutException." What is a likely solution?
Increase the wait time
Reduce screen size
Run on a different browser
Ignore the error
Q82
Q82 A script with Implicit Wait works on some elements but not others. What could be a better approach?
Add Explicit Waits for specific elements
Increase screen brightness
Run on headless mode
Decrease browser resolution
Q83
Q83 A test intermittently fails when waiting for a dynamic element. What might improve its reliability?
Increase Implicit Wait
Switch to Fluent Wait with polling
Reduce screen size
Change element locator
Q84
Q84 What is TestNG primarily used for in Selenium?
Browser automation
Test case management
Java development
Data analysis
Q85
Q85 Which annotation in TestNG is used to define a method that runs before each test?
@AfterTest
@BeforeMethod
@BeforeClass
@Test
Q86
Q86 What does the @Test annotation indicate in TestNG?
Setup method
Test method
Ignore method
Exit method
Q87
Q87 Which method in TestNG allows executing tests in a specified order?
@OrderOfExecution
@Test(priority=...)
@RunOrder
@SequentialExecution
Q88
Q88 How does TestNG manage data-driven testing?
@DataProvider
@Test
@Parameters
@Provider
Q89
Q89 How do you set up a group of tests in TestNG?
@Test(groups="groupName")
@Group(group="groupName")
@RunGroup("groupName")
@TestGroup("groupName")
Q90
Q90 How would you use a @DataProvider in TestNG to pass data to a test?
@DataProvider("name")
@Test(data="providerName")
@Test(dataProvider="name")
@Provider(name="data")