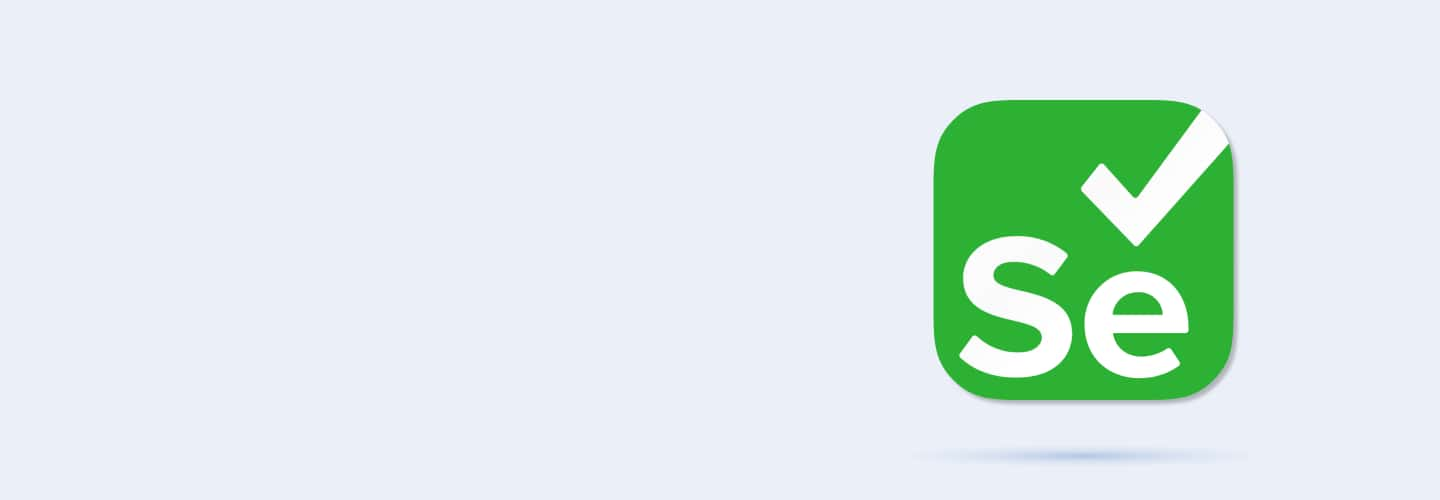
Q31
Q31 In a Maven project, where do you add Selenium dependencies to integrate Selenium WebDriver?
In pom.xml under
In test.java file
In environment variables
In an external file
Q32
Q32 A Selenium test fails to run due to missing ChromeDriver. What is a likely solution?
Reinstall Chrome
Add ChromeDriver to PATH
Ignore the error
Run on a different browser
Q33
Q33 The Chrome browser does not open when running a Selenium script. What should you check first?
Check if ChromeDriver is installed
Check if Firefox is installed
Verify Selenium IDE is working
Check if URL is valid
Q34
Q34 A test fails after updating ChromeDriver, citing version mismatch. What is the solution?
Ignore the error
Rollback ChromeDriver
Update the Chrome browser to match the driver version
Run on Firefox
Q35
Q35 Which Selenium method is used to find an element by its ID?
findElement(By.tag)
findElement(By.name)
findElement(By.id)
findElement(By.cssSelector)
Q36
Q36 What is the purpose of locators in Selenium?
To define test data
To find and interact with web elements
To increase execution speed
To manage test cases
Q37
Q37 Which locator is recommended for selecting elements with unique identifiers?
XPath
CSS Selector
Class Name
ID
Q38
Q38 What does the findElements method in Selenium WebDriver return?
A single WebElement
A list of WebElements
A string
A boolean
Q39
Q39 Which locator should you use when elements have no unique attributes, but specific text?
Name
Tag Name
Link Text
ID
Q40
Q40 When should XPath be used as a locator in Selenium?
When elements have unique IDs
When CSS selectors are not suitable
When using Selenium IDE
For faster execution
Q41
Q41 How do you find an element by class name in Selenium WebDriver?
driver.findElement(By.cssSelector())
driver.findElement(By.className())
driver.findElement(By.tagName())
driver.findByClass()
Q42
Q42 How would you locate an element with the XPath //button[text()='Submit']?
findElement(By.id("Submit"))
findElement(By.xpath("//button[text()='Submit']"))
findElement(By.tag("Submit"))
findByText("Submit")
Q43
Q43 Which Selenium method would you use to select an element using its CSS selector?
findElement(By.cssSelector())
findElement(By.name())
findElement(By.linkText())
findElement(By.tagName())
Q44
Q44 How would you locate the third <div> element using XPath?
//div[3]
//div[@id='3']
findElement(div, 3)
//div(3)
Q45
Q45 A Selenium script fails to locate an element by ID. What is the first check to perform?
Check if the ID is unique
Increase wait time
Ignore the failure
Change the locator to XPath
Q46
Q46 The script fails intermittently when locating elements with dynamic IDs. What could be a solution?
Use findElement(By.id())
Switch to CSS selectors
Ignore the test
Use dynamic locators like XPath with conditions
Q47
Q47 An element is not found during testing because it loads slowly. What is a potential fix in Selenium?
Restart the test
Use explicit wait until the element is visible
Add the element in HTML
Ignore the error
Q48
Q48 What is Selenium WebDriver primarily used for?
To automate desktop applications
To automate web applications
To generate test data
To interact with APIs
Q49
Q49 Which method is used to close the current browser window in Selenium WebDriver?
driver.quit()
driver.stop()
driver.close()
driver.exit()
Q50
Q50 What does the driver.quit() command do in Selenium WebDriver?
Closes all browser windows and ends the WebDriver session
Only closes the current window
Maximizes the browser
Navigates to a URL
Q51
Q51 Which Selenium method is used to retrieve the title of the current webpage?
driver.getText()
driver.getTitle()
driver.getHeading()
driver.getPageTitle()
Q52
Q52 What is the purpose of the manage().window().maximize() method in Selenium WebDriver?
To close the window
To switch tabs
To maximize the browser window
To take a screenshot
Q53
Q53 Which method would you use to navigate back to the previous page in Selenium WebDriver?
driver.forward()
driver.navigate().back()
driver.previousPage()
driver.back()
Q54
Q54 How do you retrieve the current URL of a page in Selenium WebDriver?
driver.getPageURL()
driver.getUrl()
driver.getCurrentUrl()
driver.get()
Q55
Q55 Which method would you use to clear text from an input field in Selenium WebDriver?
element.clearText()
element.delete()
element.clear()
element.removeText()
Q56
Q56 How do you take a screenshot in Selenium WebDriver?
driver.captureScreenshot()
driver.takeScreenshot()
driver.getScreenshotAs()
driver.saveScreen()
Q57
Q57 In Selenium WebDriver, how do you handle a checkbox?
element.select()
element.toggle()
element.click()
element.activate()
Q58
Q58 A Selenium test script fails with a "NoSuchElementException" error. What could be the cause?
Incorrect element locator
Network connection issues
Outdated ChromeDriver
Unsupported programming language
Q59
Q59 The browser does not navigate to a URL provided in driver.get(). What could be the reason?
URL is incorrect
Driver version mismatch
Selenium IDE is not installed
The element is not visible
Q60
Q60 A script encounters a "StaleElementReferenceException." What does this imply in Selenium WebDriver?
The element is missing attributes
The page has been refreshed or updated
The browser is closed
The element has duplicate IDs