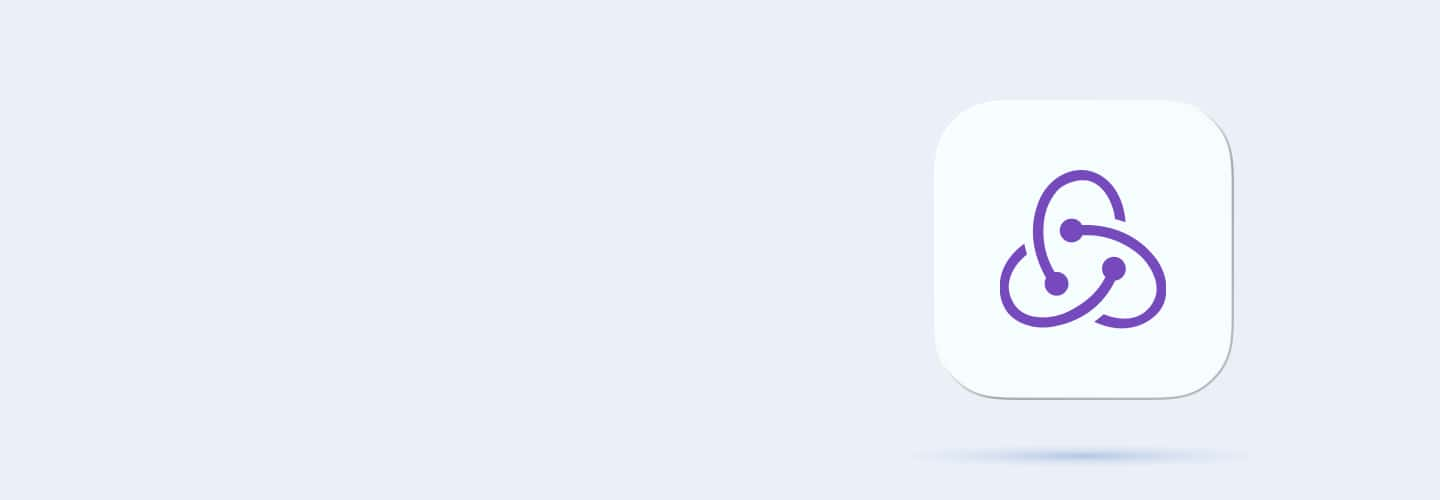
Q121
Q121 A developer sees that Redux actions are being dispatched multiple times unexpectedly. What could be the cause?
A component is dispatching an action in useEffect without a dependency array
The reducer is mutating state directly
Middleware is missing
The Redux store is corrupted
Q122
Q122 What is the main reason for performance issues in a Redux application?
Too many action types
Excessive re-renders due to unnecessary state updates
Using Redux with React
Not using Redux middleware
Q123
Q123 How can useSelector() be optimized to prevent unnecessary re-renders?
Use multiple useSelector() calls in a component
Use useMemo() inside the selector
Always select the entire Redux state
Use shallowEqual as a second argument in useSelector()
Q124
Q124 Why is it important to normalize state structure in Redux?
It increases the number of re-renders
It makes it easier to modify state directly
It prevents deep nested objects that cause inefficient state updates
It removes the need for reducers
Q125
Q125 What is the benefit of using reselect in Redux?
It allows modifying the Redux store directly
It enables memoization to optimize state-derived values
It reduces the number of Redux actions needed
It removes the need for reducers
Q126
Q126 What is the correct way to memoize derived state using reselect?
const getFilteredItems = createSelector([state => state.items], items => items.filter(item => item.active));
const getFilteredItems = state => state.items.filter(item => item.active);
const getFilteredItems = () => state.items.filter(item => item.active);
const getFilteredItems = new Selector(state.items, item => item.active);
Q127
Q127 Identify the issue in the following Redux store setup: const store = createStore(rootReducer, applyMiddleware(thunk, logger, logger));
The applyMiddleware function is incorrect
The logger middleware is applied twice, leading to redundant logs
Redux does not allow multiple middlewares
The reducer is missing
Q128
Q128 A Redux application is experiencing performance issues when updating the state. What is the most likely cause?
The reducer is modifying the state directly
The Redux state tree is deeply nested and complex
Middleware is missing
Redux is running in development mode
Q129
Q129 A developer notices that a component is re-rendering frequently even when the relevant Redux state has not changed. What could be the cause?
The component is missing useEffect
The component is using useSelector() without a memoized selector
Redux does not support React hooks
The store is not updating
Q130
Q130 What is the main advantage of using Redux Toolkit (RTK) over traditional Redux?
It allows direct state mutation in reducers
It simplifies state management by reducing boilerplate
It removes the need for actions
It eliminates the need for reducers
Q131
Q131 Which function in Redux Toolkit replaces createStore() from traditional Redux?
configureStore()
createSlice()
createReducer()
createStore()
Q132
Q132 What is a key benefit of using createSlice() in Redux Toolkit?
It automatically generates action creators and reducers together
It replaces Redux middleware
It eliminates the need for dispatching actions
It removes the need for React components
Q133
Q133 How do you correctly dispatch an action from a slice in Redux Toolkit?
dispatch(counterSlice.increment())
dispatch({ type: 'increment' })
dispatch({ increment: true })
dispatch(increment())
Q134
Q134 Identify the issue in the following RTK store configuration: const store = configureStore({ reducer: counterReducer, middleware: [thunk] });
Middleware should not be an array
Thunk should be imported separately
The middleware property should be a function returning an array
The reducer must be wrapped in combineReducers
Q135
Q135 A Redux Toolkit application is not logging actions in the console. What could be the cause?
Redux Toolkit does not support logging
Middleware like redux-logger is missing
The reducer is incorrect
Actions are not being dispatched
Q136
Q136 A developer is using createAsyncThunk(), but actions are not updating the store. What could be the issue?
The extraReducers field is missing in createSlice()
Async actions cannot modify Redux state
The store is not configured correctly
Thunk middleware is missing
Q137
Q137 What is the purpose of Redux Persist?
To automatically refresh the Redux store on every page load
To persist Redux state across page reloads or browser restarts
To store Redux state in a global variable
To replace Redux reducers
Q138
Q138 Where does Redux Persist store the persisted state by default?
Session storage
Local storage
Redux store
Global state object
Q139
Q139 What is a potential drawback of using Redux Persist?
It slows down Redux actions
It prevents state updates
It increases app startup time if the persisted state is large
It removes the need for Redux middleware
Q140
Q140 Which configuration option is required to ensure that Redux Persist only persists a specific part of the Redux state?
whitelist in persistConfig
persistAll function
store.persist()
autoPersist: true
Q141
Q141 Identify the issue in the following Redux Persist configuration: const persistConfig = { key: 'root', storage: sessionStorage }; const persistedReducer = persistReducer(persistConfig, rootReducer); const store = configureStore({ reducer: persistedReducer });
The persistStore() function is missing
sessionStorage is not a valid Redux Persist storage
The reducer is missing middleware
The Redux store should not use persisted reducers
Q142
Q142 A Redux Persist setup is not restoring the persisted state after a page reload. What could be the cause?
The persisted reducer is missing
Middleware is not configured correctly
The key used in persistConfig does not match the store key
The app does not have access to Redux DevTools
Q143
Q143 A Redux Persist setup is working but is causing performance issues. What could be the reason?
The persisted state is too large
Redux Persist does not work well with React
Persisting middleware is missing
The app is using too many reducers
Q144
Q144 What is one key advantage of using Redux in large-scale applications?
It eliminates the need for React state
It provides a predictable state management pattern
It improves performance by reducing re-renders
It removes the need for API calls
Q145
Q145 How does Redux help in managing state in an e-commerce application?
By keeping UI components in sync with the global store
By replacing backend API calls
By eliminating the need for local component state
By improving the rendering speed of React components
Q146
Q146 What is a common challenge when using Redux in real-world applications?
Managing asynchronous state updates
Directly modifying the Redux store
Handling local component state
Writing reducers
Q147
Q147 How can Redux handle theme selection (light/dark mode) in an application?
Store theme in local state
Use Redux state to track theme
Use Redux middleware
Use React Context instead of Redux
Q148
Q148 Identify the issue in the following Redux setup for user profiles: const userReducer = (state = {}, action) => { if (action.type === 'UPDATE_PROFILE') { state.user = action.payload; return state; } return state; };
The reducer is missing a return statement
The reducer is modifying the existing state directly instead of returning a new object
The action type is incorrect
The reducer is not connected to the store
Q149
Q149 A Redux-based project management application has sluggish performance when updating tasks. What could be a potential cause?
Redux does not support large applications
State updates are not being handled properly
Too many actions are dispatched unnecessarily
Middleware is missing in the Redux store
Q150
Q150 A social media application built with Redux is experiencing delays when fetching posts. What could be a reason for this issue?
The Redux store is too large
Middleware like Thunk is not properly handling async actions
The Redux DevTools are causing issues
Redux cannot handle real-time data