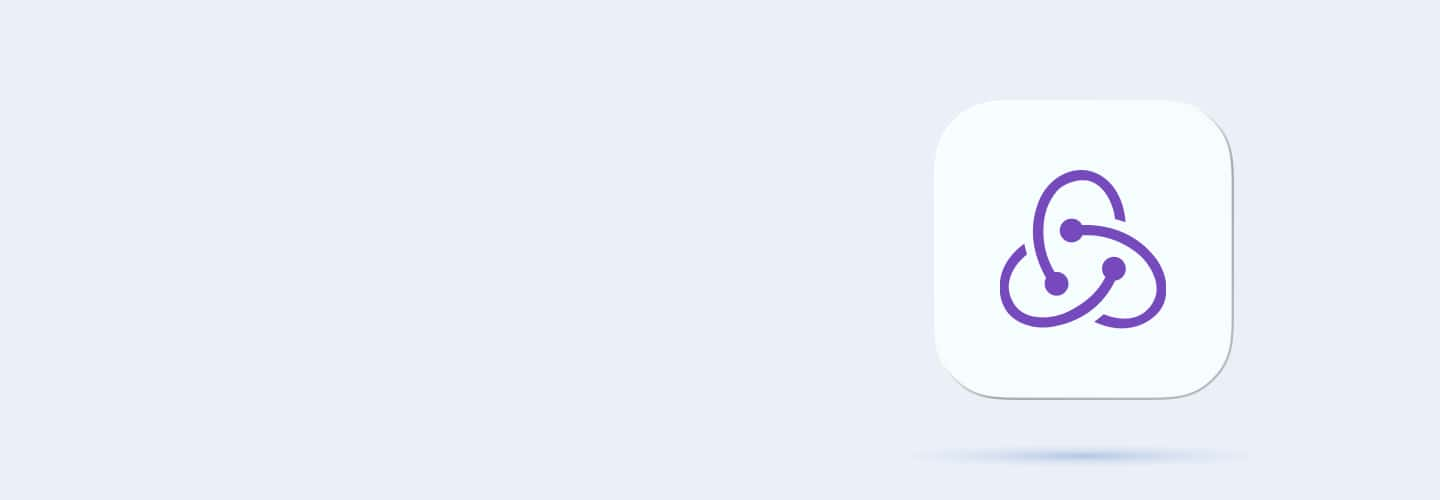
Q91
Q91 A developer notices that actions are dispatched correctly, but the UI does not update. What could be the issue?
The Redux state is being mutated in the reducer.
Middleware is missing.
The dispatch function is not working.
The store is not updating the UI.
Q92
Q92 What are side effects in Redux?
Any synchronous state update inside reducers
Actions that modify the Redux store directly
Operations like API calls, timers, or logging that happen outside Redux
Pure functions that return a new state
Q93
Q93 Which of the following is NOT a recommended way to handle side effects in Redux?
Using Redux Thunk for async actions
Using Redux Saga for complex side effects
Directly modifying the Redux state inside reducers
Using middleware like Redux Observable
Q94
Q94 How does Redux middleware help manage side effects?
It allows async operations to be handled outside reducers
It removes the need for action creators
It replaces reducers
It modifies the store directly
Q95
Q95 Why should API calls not be made inside Redux reducers?
API calls are synchronous and block UI updates
Reducers must be pure functions without side effects
Reducers cannot access external APIs
Reducers should return promises instead of state objects
Q96
Q96 What is the primary difference between Redux Thunk and Redux Saga?
Thunk is synchronous while Saga is asynchronous
Thunk uses functions inside actions, while Saga uses generators to manage side effects
Thunk modifies reducers, while Saga modifies actions
Saga requires no additional setup while Thunk does
Q97
Q97 How do you use Redux Thunk to fetch data in an action creator?
const fetchData = () => dispatch => { dispatch({ type: 'FETCH_START' }); fetch('/api/data').then(res => res.json()).then(data => dispatch({ type: 'FETCH_SUCCESS', payload: data })); };
const fetchData = () => { return { type: 'FETCH_START' }; }
const fetchData = async () => { const data = await fetch('/api/data'); return { type: 'FETCH_SUCCESS', payload: data }; }
const fetchData = () => store.getState();
Q98
Q98 What will happen in the following code? const fetchData = () => async dispatch => { dispatch({ type: 'FETCH_START' }); const data = await fetch('/api/data').then(res => res.json()); dispatch({ type: 'FETCH_SUCCESS', payload: data }); };
The Redux action will fail because async is not supported
The API call will execute and update the store correctly
The function must return a promise instead of dispatching actions
The await keyword should not be used in Redux
Q99
Q99 Given the following Redux Saga effect, what will happen? function* fetchDataSaga() { yield takeEvery('FETCH_DATA', function* () { const data = yield call(fetch, '/api/data'); yield put({ type: 'FETCH_SUCCESS', payload: data }); }); }
The saga listens for FETCH_DATA actions and makes an API call when triggered
The saga replaces the Redux store
The reducer modifies the store directly
The saga runs once on app load and never again
Q100
Q100 Identify the issue in the following Redux Saga function: function* fetchDataSaga() { const response = yield call(fetch, '/api/data'); const data = yield response.json(); yield put({ type: 'FETCH_SUCCESS', payload: data }); }
The call effect does not return a promise
The response needs to be converted using yield call([response, 'json'])
The fetch call should be placed inside a reducer
The saga should return an action object instead of dispatching it
Q101
Q101 A Redux Thunk async action is not dispatching the success action after an API call. What could be the issue?
The API response is incorrect
The function does not return a promise
The success action is missing in the dispatch chain
Redux Thunk does not support API calls
Q102
Q102 A Redux Saga function is stuck and not dispatching actions. What could be the issue?
The Saga function is missing a yield statement
The reducer is not handling the actions
The dispatch function is not working
Saga cannot handle side effects
Q103
Q103 A developer notices that API requests are being made multiple times unexpectedly in Redux Thunk. What could be the cause?
The action is dispatched multiple times without condition checks
Redux does not support async actions
Reducers modify the Redux store directly
Middleware is missing in the Redux setup
Q104
Q104 Why is immutability important in Redux?
It improves the performance of React components
It ensures the Redux store can modify state directly
It prevents unnecessary re-renders in React and ensures predictable state updates
It allows direct changes to be made inside reducers
Q105
Q105 How should state updates be handled in Redux reducers?
By modifying the existing state object
By using the setState function
By returning a new object with updated properties
By using this.state directly
Q106
Q106 What happens if a reducer mutates the state directly?
Redux throws an error
React may not detect changes, leading to incorrect UI updates
The Redux store automatically corrects the mutation
The action gets dispatched twice
Q107
Q107 Which of the following is the best way to ensure immutability when updating an array in Redux?
Using the spread operator (...state, newItem)
Using state.push(newItem)
Modifying state directly
Using a global variable
Q108
Q108 How do you correctly update an object property in a Redux reducer while ensuring immutability?
return { ...state, count: state.count + 1 };
state.count++; return state;
return Object.assign(state, { count: state.count + 1 });
state = { count: state.count + 1 }; return state;
Q109
Q109 What is the correct way to add an item to an array stored in Redux state?
return { ...state, items: state.items.push(newItem) };
return { ...state, items: [...state.items, newItem] };
state.items.push(newItem); return state;
return { state.items.concat(newItem) };
Q110
Q110 Identify the issue in the following reducer: function reducer(state = { items: [] }, action) { if (action.type === 'ADD_ITEM') { state.items.push(action.payload); return state; } return state; }
The reducer does not handle actions correctly
The reducer mutates state directly instead of returning a new object
The reducer is missing an initial state
The reducer should use useState instead of Redux
Q111
Q111 A Redux state update is not triggering a UI re-render. What could be the issue?
The reducer is modifying the existing state instead of returning a new one
The component is not connected to Redux
The action type is missing
The Redux store is missing middleware
Q112
Q112 A developer sees the following warning in their Redux application: "Do not mutate state directly inside reducers." What is the likely cause?
The reducer is returning a new state object instead of modifying it
The reducer is modifying the state object directly
Middleware is missing
Actions are dispatched incorrectly
Q113
Q113 What is the best way to debug Redux state changes?
Using console.log inside reducers
Using the Redux DevTools extension
Manually checking the store.getState() function
Observing UI changes
Q114
Q114 What is a common symptom of a reducer mutating the state instead of returning a new object?
The Redux store crashes immediately
Actions stop being dispatched
State updates do not trigger re-renders in React components
The store automatically resets to the initial state
Q115
Q115 A Redux action is being dispatched, but the state is not updating. What could be the possible cause?
The action type is not handled in the reducer
The Redux store is missing middleware
The reducer is modifying state directly
The dispatch function is incorrect
Q116
Q116 A Redux application crashes with the error: "Reducers may not dispatch actions." What is the likely cause?
An action is missing a type property
A reducer is calling dispatch inside itself
The Redux store has no initial state
Middleware is incorrectly applied
Q117
Q117 What is the issue in the following reducer? function reducer(state = { count: 0 }, action) { switch (action.type) { case 'INCREMENT': state.count += 1; return state; default: return state; } }
The reducer should return null instead of state
The reducer is mutating the state directly
The reducer should call dispatch instead
State should be reset inside the reducer
Q118
Q118 What is the correct way to update state in the following reducer? function reducer(state = { todos: [] }, action) { if (action.type === 'ADD_TODO') { state.todos.push(action.payload); return state; } return state; }
return { ...state, todos: [...state.todos, action.payload] };
state.todos.push(action.payload); return state;
state = { todos: action.payload }; return state;
return Object.assign(state, { todos: action.payload });
Q119
Q119 Identify the issue in the following Redux Thunk action: const fetchData = () => async dispatch => { const data = await fetch('/api/data').then(res => res.json()); dispatch({ type: 'FETCH_SUCCESS', payload: data }); dispatch({ type: 'FETCH_SUCCESS', payload: data }); };
The API call is missing
The function is dispatching the same action twice
The reducer is incorrect
Middleware is missing
Q120
Q120 A Redux application is not persisting state between page reloads. What is a possible solution?
Use Redux middleware
Use localStorage to save and rehydrate the state
Add more reducers to the store
Use React Context instead of Redux