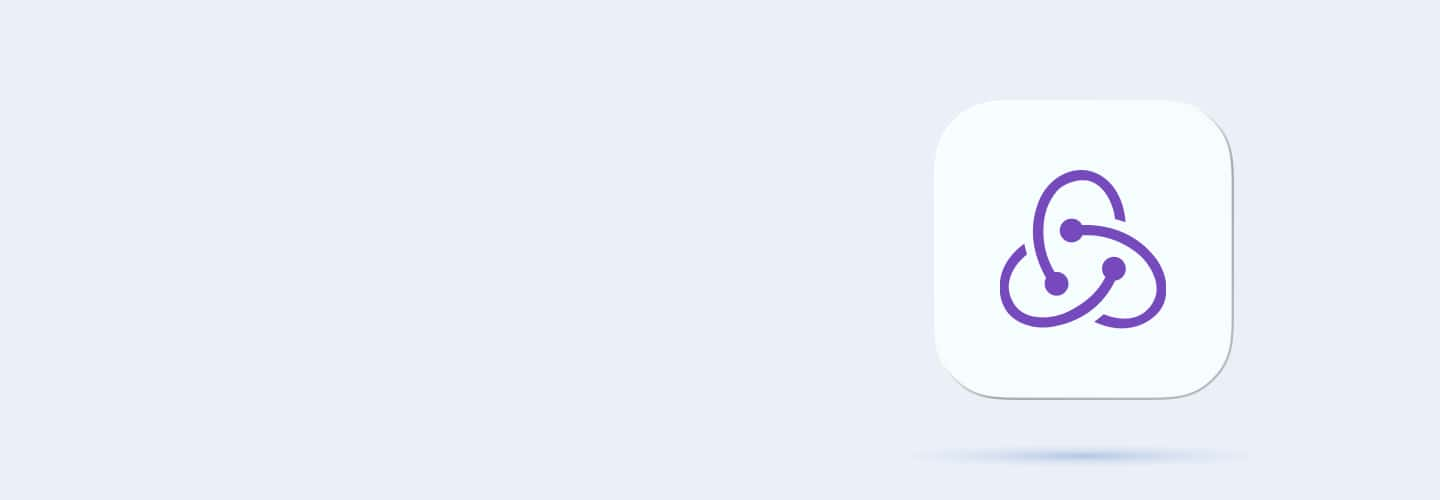
Q61
Q61 How do you apply middleware in a Redux store?
const store = createStore(rootReducer, applyMiddleware(logger));
const store = new Store(rootReducer, middleware);
const store = configureStore(middleware);
const store = setMiddleware(rootReducer, logger);
Q62
Q62 Given the middleware function below, what will it log? const logger = store => next => action => { console.log('Dispatching:', action.type); return next(action); };
Only the action type before it reaches the reducer.
The new state after an action is processed.
The entire Redux store state.
Nothing, as middleware does not log actions.
Q63
Q63 What will happen if next(action) is omitted in middleware?
The middleware will log the action twice.
The reducer will not receive the action.
The Redux store will crash.
Middleware will continue execution as normal.
Q64
Q64 Identify the issue in the following middleware: const customMiddleware = store => next => action => { console.log('Action:', action.type); next(action); store.dispatch({ type: 'LOGGED_ACTION' }); };
Middleware should not dispatch actions after calling next(action).
Middleware must always modify the state directly.
Middleware should return undefined.
Middleware should not log actions.
Q65
Q65 A Redux application with middleware does not process an action. What is the most likely cause?
Middleware is not applied using applyMiddleware.
Middleware is modifying the Redux store.
The reducer is missing an initial state.
Middleware is using store.getState().
Q66
Q66 A developer notices that actions are not reaching the reducer when using middleware. What could be the issue?
The middleware does not call next(action).
Middleware is logging actions incorrectly.
Reducers are mutating the state directly.
The Redux store is missing an initial state.
Q67
Q67 A Redux application with async middleware is dispatching multiple actions, but only the first one updates the state. What could be the cause?
The middleware is dispatching actions incorrectly.
Actions are modifying the state directly.
Middleware is calling dispatch without ensuring state updates complete.
Reducers are mutating the state.
Q68
Q68 What is Redux Thunk used for?
Handling synchronous state updates
Executing async logic inside reducers
Allowing action creators to return functions instead of action objects
Rewriting reducers dynamically
Q69
Q69 Why is Redux Thunk needed in Redux applications?
Redux only supports synchronous updates by default.
Reducers cannot handle action types.
Middleware is required for Redux to work.
Redux uses Thunk for debugging.
Q70
Q70 How does a Redux Thunk function typically dispatch actions?
It dispatches actions only when the component re-renders.
It allows an action creator to return a function that receives dispatch.
It replaces reducers with middleware.
It prevents the store from updating state.
Q71
Q71 What parameter does Redux Thunk provide to the function returned by an action creator?
getState
dispatch
store
Both dispatch and getState
Q72
Q72 What happens if you dispatch a plain object instead of a function while using Redux Thunk?
The object is ignored by the store.
The action is dispatched normally.
Redux throws an error.
The reducer modifies the store directly.
Q73
Q73 How do you correctly define an async action using Redux Thunk?
const fetchData = () => { return dispatch => { dispatch({ type: 'LOADING' }); fetchData().then(data => dispatch({ type: 'SUCCESS', payload: data })); } };
const fetchData = dispatch => { dispatch({ type: 'LOADING' }); fetchData().then(data => dispatch({ type: 'SUCCESS', payload: data })); }
const fetchData = () => ({ type: 'FETCH_DATA' });
const fetchData = dispatch => dispatch({ type: 'FETCH_DATA' });
Q74
Q74 Given the following code, what is missing? const fetchData = () => { return dispatch => { dispatch({ type: 'REQUEST_STARTED' }); fetch('https://api.example.com/data') .then(response => response.json()) .then(data => dispatch({ type: 'REQUEST_SUCCESS', payload: data })); }; };
The initial state is missing.
There is no error handling.
The reducer is incorrect.
Redux Thunk is not installed.
Q75
Q75 What is the purpose of calling dispatch({ type: 'LOADING' }) before making an async API request?
To reset the Redux store
To indicate that an API request has started
To fetch data from the local store
To replace the reducer
Q76
Q76 Identify the issue in the following Redux Thunk action: const fetchData = () => dispatch => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => dispatch({ type: 'FETCH_SUCCESS', payload: data })); };
The dispatch function is missing in the returned function.
The reducer cannot handle async actions.
The function should return a promise.
The dispatch function should be passed as an argument.
Q77
Q77 A Redux Thunk action is not updating the state. What could be a possible reason?
The reducer is modifying the state directly.
The Thunk function is not dispatching an action.
Middleware is missing from Redux.
Actions cannot modify state.
Q78
Q78 A developer notices that async actions work in development but fail in production. What could be the cause?
Redux Thunk is not installed in production.
The reducer is not updating the state.
Actions are missing a payload.
Async actions are only allowed in development.
Q79
Q79 A Redux Thunk action is dispatching multiple times, causing unexpected state changes. What could be the issue?
The API response is incorrect.
The reducer is mutating state directly.
The action creator is dispatching inside a loop without checking conditions.
The store is missing middleware.
Q80
Q80 What is the primary purpose of React-Redux?
To manage component state
To connect Redux state and actions to React components
To replace Redux reducers
To store React component props globally
Q81
Q81 Which component is used to make the Redux store available to all React components?
<StoreProvider>
<Provider>
<ReduxContainer>
<Context>
Q82
Q82 How does a React component access the Redux state?
By using useState()
By calling store.getState() inside the component
By using useSelector() from React-Redux
By dispatching an action
Q83
Q83 What does useDispatch() do in a React-Redux component?
Connects the component to the Redux state
Dispatches actions to the Redux store
Replaces the Redux store
Fetches data from an API
Q84
Q84 Why is it recommended to use useSelector() instead of accessing store.getState() directly?
useSelector() only returns the entire state.
Directly calling store.getState() causes unnecessary re-renders.
useSelector() memoizes state selections and prevents unnecessary re-renders.
store.getState() modifies the Redux store.
Q85
Q85 How do you correctly use useSelector() to get the Redux state in a component?
const count = useSelector(state => state.count);
const count = store.getState().count;
const count = state.useSelector(count);
const count = store.count;
Q86
Q86 What is the correct way to connect a class component to Redux using connect()?
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
export default MyComponent(connect(mapStateToProps, mapDispatchToProps));
connect(MyComponent)(mapStateToProps, mapDispatchToProps);
export default mapStateToProps(connect(MyComponent, mapDispatchToProps));
Q87
Q87 Given the following component, what will happen? const Counter = () => { const count = useSelector(state => state.count); const dispatch = useDispatch(); return (<button onClick={() => dispatch({ type: 'INCREMENT' })}>{count}); };
The count will update correctly when clicked.
The button will not display the count.
The reducer will not update the state.
The button click will cause an infinite loop.
Q88
Q88 Identify the issue in the following component: const Counter = () => { const count = useSelector(state => state.count); const dispatch = useDispatch(); return (<button onClick={() => dispatch(increment())}>{count}); };
increment() should be defined inside the component.
The increment() function should be imported as an action creator.
The useDispatch() hook should be inside a useEffect().
useSelector() should return a function.
Q89
Q89 A React component does not update when the Redux state changes. What could be a possible reason?
The component is missing useEffect().
The Redux state is being mutated inside the reducer.
The component is missing useState().
The Redux store has no actions.
Q90
Q90 A Redux-connected component throws an error saying store is undefined. What could be the issue?
The Redux store is not properly provided using
The connect() function is used incorrectly.
The useSelector() hook is missing.
The component is not using useState().