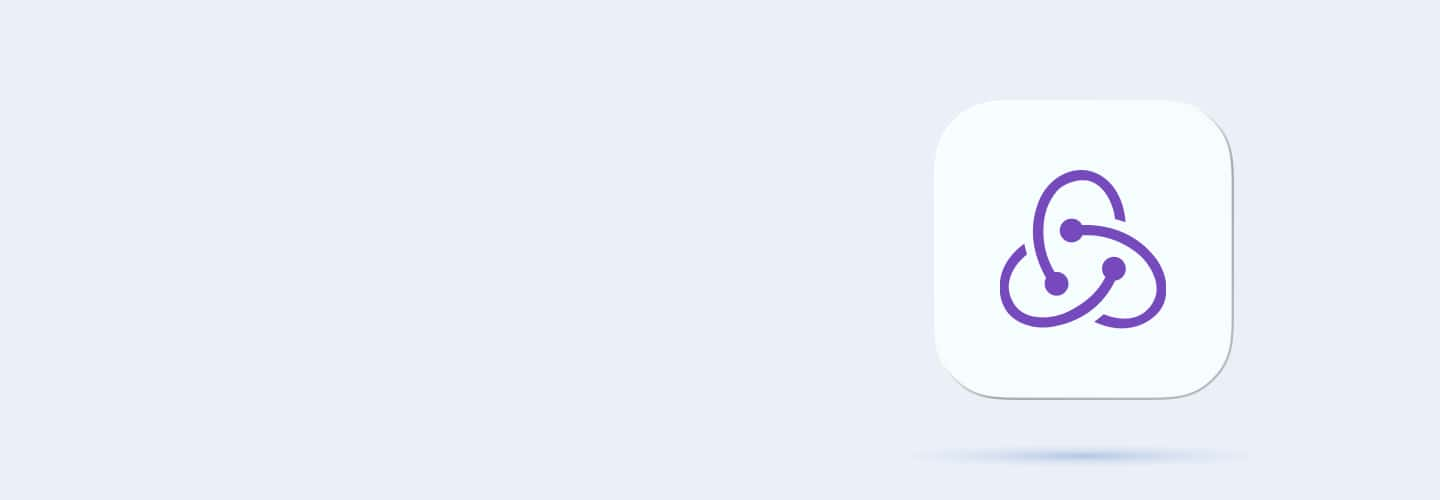
Q31
Q31 A developer notices that the Redux state resets on every page refresh. What could be a possible solution?
Use localStorage to save the state
Dispatch an action on page load
Use a global variable instead of Redux
Call store.subscribe() in every component
Q32
Q32 What is an action in Redux?
A function that modifies the store directly.
An object that describes a state change.
A method that fetches data from an API.
A middleware that connects reducers to the store.
Q33
Q33 What is the minimum required property in a Redux action object?
type
payload
dispatch
store
Q34
Q34 What does an action creator do in Redux?
Directly modifies the store
Returns an action object
Handles API calls
Creates a new reducer
Q35
Q35 How is an action dispatched in Redux?
store.dispatch(action)
store.setState(action)
dispatch(action)
action.dispatch()
Q36
Q36 Why should action creators be used instead of directly dispatching action objects?
To improve performance
To avoid hardcoded action objects in components
To make Redux asynchronous
To allow reducers to modify actions
Q37
Q37 What is the purpose of a payload in a Redux action?
It identifies the type of action.
It holds additional data needed for the state change.
It modifies the state directly.
It acts as middleware.
Q38
Q38 What will be the output of the following action creator? const increment = () => ({ type: 'INCREMENT' });
{ type: 'INCREMENT' }
{ type: INCREMENT }
{ INCREMENT: true }
An error occurs
Q39
Q39 How would you define an action creator that takes a payload?
const addItem = (item) => ({ type: 'ADD_ITEM', payload: item });
const addItem = (item) => ( type: 'ADD_ITEM', item );
const addItem = (item) => { return 'ADD_ITEM', item; }
const addItem = (item) => ({ ADD_ITEM, payload: item });
Q40
Q40 What will the following code output? const loginUser = (username) => ({ type: 'LOGIN', user: username }); console.log(loginUser('john_doe'));
{ type: 'LOGIN', user: 'john_doe' }
{ LOGIN: true, user: 'john_doe' }
{ type: 'LOGIN' }
An error occurs
Q41
Q41 An action is dispatched, but the state does not update. What could be the reason?
The action object is missing a type property.
The action is dispatched incorrectly.
The action payload is undefined.
The reducer is missing an initial state.
Q42
Q42 A Redux action is dispatched, but no changes occur in the store. What is a possible reason?
The action creator is incorrect.
The reducer does not handle the action type.
The store is missing middleware.
The action is asynchronous.
Q43
Q43 A developer notices that dispatched actions are being logged but not affecting the state. What could be the problem?
The reducer is returning a modified state object.
The Redux store is not configured correctly.
The action is dispatched in the wrong component.
The action type does not match any case in the reducer.
Q44
Q44 What is a reducer in Redux?
A function that modifies the store directly.
A function that returns a new state based on an action.
A function that dispatches an action.
A function that fetches data from an API.
Q45
Q45 Why should reducers be pure functions?
To prevent infinite loops in React components.
To allow asynchronous operations in Redux.
To ensure predictability and avoid side effects.
To improve Redux performance.
Q46
Q46 What should a reducer return if no matching action type is found?
A modified version of the previous state.
A new state object.
The previous state unchanged.
An error.
Q47
Q47 What is the default value of state in a Redux reducer?
null
undefined
An empty object
An empty array
Q48
Q48 How should state updates be handled in a reducer?
By mutating the existing state directly.
By creating and returning a new state object.
By updating state asynchronously.
By modifying the Redux store directly.
Q49
Q49 What happens if a reducer mutates the state instead of returning a new state?
Redux throws an error.
React still updates the UI normally.
Redux considers no state change has occurred.
The action gets dispatched again.
Q50
Q50 What will be the output of the following reducer? function reducer(state = { count: 0 }, action) { if (action.type === 'INCREMENT') { return { count: state.count + 1 }; } return state; } console.log(reducer(undefined, { type: 'INCREMENT' }));
{ count: 1 }
{ count: 0 }
undefined
An error occurs
Q51
Q51 Given the following reducer, what will console.log(reducer({ count: 5 }, { type: 'RESET' })) output? function reducer(state = { count: 0 }, action) { switch (action.type) { case 'INCREMENT': return { count: state.count + 1 }; case 'RESET': return { count: 0 }; default: return state; } }
{ count: 0 }
{ count: 5 }
undefined
An error occurs
Q52
Q52 Identify the issue in the following reducer: function reducer(state = { count: 0 }, action) { if (action.type === 'INCREMENT') { state.count++; return state; } return state; }
The reducer should mutate state directly.
The reducer is missing an initial state.
The reducer is mutating state directly instead of returning a new object.
The reducer does not return a default state.
Q53
Q53 A Redux state change is not reflecting in the UI. What is the most likely cause?
The reducer is modifying the existing state instead of returning a new one.
The Redux store is missing middleware.
The action type is incorrect.
The component is not wrapped with Provider.
Q54
Q54 A Redux application is throwing an undefined state error in the reducer. What is a possible cause?
The reducer is missing a default parameter for the initial state.
The action type is incorrect.
The store is missing middleware.
The action creator is incorrect.
Q55
Q55 A developer notices that the Redux state resets unexpectedly when dispatching an action. What could be the cause?
The reducer is missing a return statement for the default case.
The action type is incorrect.
The store is missing middleware.
The state is too large.
Q56
Q56 What is the primary purpose of middleware in Redux?
To modify the store directly.
To intercept actions and execute side effects.
To replace reducers.
To store actions for debugging.
Q57
Q57 Which of the following is an example of a common use case for middleware?
Directly modifying Redux state
Performing async API calls before an action reaches the reducer
Replacing the Redux store
Defining new action types dynamically
Q58
Q58 What function does middleware wrap in Redux?
store.dispatch()
store.getState()
store.subscribe()
store.replaceReducer()
Q59
Q59 What argument does Redux middleware receive first?
Dispatch function
State object
Action object
Next function
Q60
Q60 Why must middleware call next(action) instead of dispatching an action directly?
To ensure actions continue through the middleware chain.
To modify the Redux store directly.
To prevent state updates.
To bypass reducers.