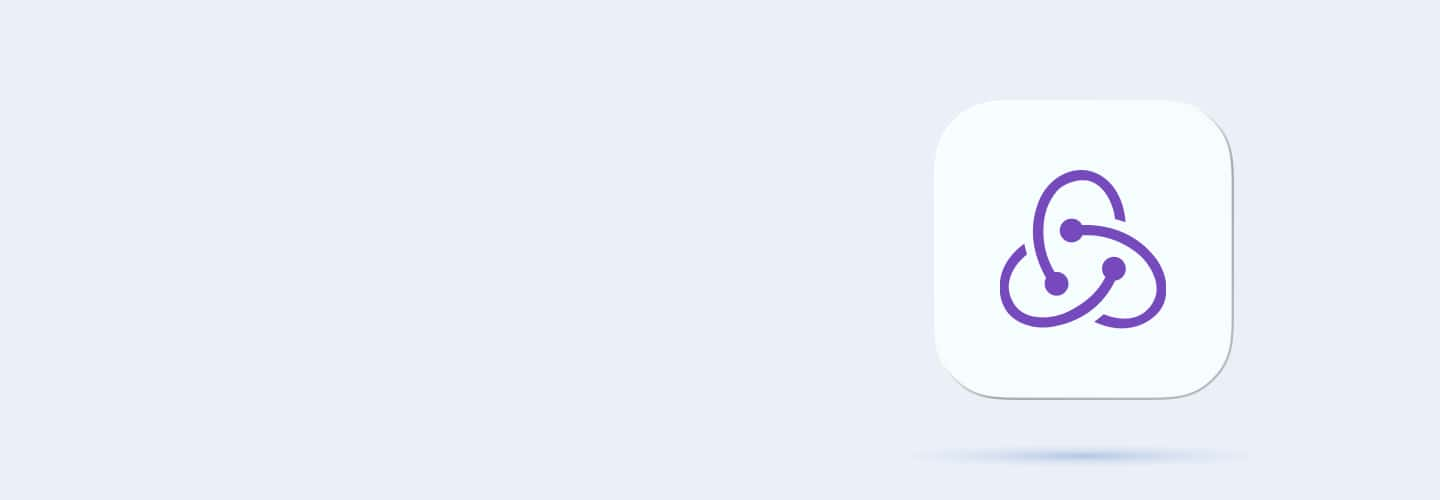
Q1
Q1 What is the primary purpose of Redux in a React application?
To manage and centralize the application state.
To improve the performance of React components.
To create UI components dynamically.
To handle routing in a React application.
Q2
Q2 Redux follows which type of data flow?
Bidirectional
Cyclic
Unidirectional
Random
Q3
Q3 What is the single source of truth in Redux?
Actions
Reducers
Store
Middleware
Q4
Q4 What is the main advantage of using Redux?
It allows multiple sources of truth.
It enables asynchronous state updates.
It helps manage application state predictably.
It eliminates the need for components.
Q5
Q5 Redux is most commonly used with which JavaScript library/framework?
Vue.js
Angular
React
Svelte
Q6
Q6 What are the three core principles of Redux?
Single source of truth, state is read-only, changes are made with pure functions.
Single store, multiple reducers, mutable state.
Actions, selectors, and reducers.
Middleware, store, and dispatcher.
Q7
Q7 Why should reducers be pure functions in Redux?
To modify the global state directly.
To ensure predictable state updates without side effects.
To improve performance of React components.
To make Redux asynchronous.
Q8
Q8 Which function is used to create a Redux store?
configureStore()
createReducer()
createStore()
combineReducers()
Q9
Q9 A Redux application does not update the UI after dispatching an action. What could be the most likely cause?
The reducer is returning the same state object without modification.
The store is missing an initial state.
The application is using an outdated version of React.
The component does not import CSS styles properly.
Q10
Q10 Which of the following is NOT a core principle of Redux?
Single source of truth
State is read-only
Changes are made with pure functions
Direct state mutation
Q11
Q11 Why does Redux follow a single source of truth principle?
To allow direct access to multiple states
To improve UI rendering speed
To maintain a centralized state for predictable updates
To reduce API calls
Q12
Q12 What is the primary role of actions in Redux?
To directly modify the store
To describe an event that causes a state change
To update the component's local state
To fetch data from an API
Q13
Q13 What function is responsible for determining how the state changes in Redux?
Actions
Reducers
Dispatch
Middleware
Q14
Q14 In Redux, what is the purpose of the dispatch function?
To trigger an action that updates the state
To access the current state
To create a reducer
To replace the store
Q15
Q15 Why is the Redux state immutable?
To prevent re-renders in React components
To avoid conflicts when multiple components access the same state
To improve JavaScript performance
To allow direct object mutations
Q16
Q16 What should a Redux reducer always return?
A new state object
A modified version of the existing state
A function
An action object
Q17
Q17 Why does Redux use pure functions for reducers?
To allow async operations
To maintain predictability and avoid side effects
To improve UI rendering performance
To enable direct state modifications
Q18
Q18 How do you define a simple reducer in Redux?
function reducer(state, action) { state.count += 1; return state; }
function reducer(state = { count: 0 }, action) { return { ...state, count: state.count + 1 }; }
function reducer() { return null; }
function reducer(state, action) { return state.count; }
Q19
Q19 A Redux reducer is not updating the state correctly. What is the most likely issue?
The reducer is modifying the existing state directly.
The action type is undefined.
The store is missing middleware.
The reducer does not have access to dispatch.
Q20
Q20 What is the purpose of the Redux store?
To manage and hold the global application state
To create UI components dynamically
To handle user authentication
To fetch data from APIs
Q21
Q21 How many stores should a Redux application ideally have?
One
Multiple
One per component
One per reducer
Q22
Q22 Which method is used to retrieve the current state from the Redux store?
store.dispatch()
store.getState()
store.updateState()
store.subscribe()
Q23
Q23 How does the Redux store update its state?
By directly modifying the state object
By dispatching an action
By calling store.updateState()
By using setState()
Q24
Q24 What is the role of store.subscribe() in Redux?
It modifies the Redux store.
It listens for state changes and triggers a callback.
It resets the store state.
It dispatches an action automatically.
Q25
Q25 What happens if a Redux reducer does not return a new state?
The state remains unchanged.
Redux automatically creates a new state.
An error occurs in Redux.
The component re-renders with the old state.
Q26
Q26 What should be passed as an argument when creating a Redux store?
An array of actions
A single reducer or root reducer
A Redux component
An API URL
Q27
Q27 How do you create a Redux store using createStore?
const store = createStore(rootReducer);
const store = new Store(rootReducer);
const store = ReduxProvider(rootReducer);
const store = setState(rootReducer);
Q28
Q28 Given the following reducer, what will be the initial state if none is provided? function reducer(state, action) { if (action.type === 'INCREMENT') { return { count: state.count + 1 }; } return state; }
{ count: 1 }
{ count: 0 }
undefined
An error occurs
Q29
Q29 A Redux application does not reflect state updates in the UI. What could be a possible reason?
The store is not created using createStore()
Reducers are returning the same state object
Actions are not dispatched
Middleware is missing
Q30
Q30 A Redux store is initialized but does not trigger updates. What could be the issue?
No initial state is defined
Reducers are not pure functions
Actions are not dispatched
Reducers are directly modifying the state