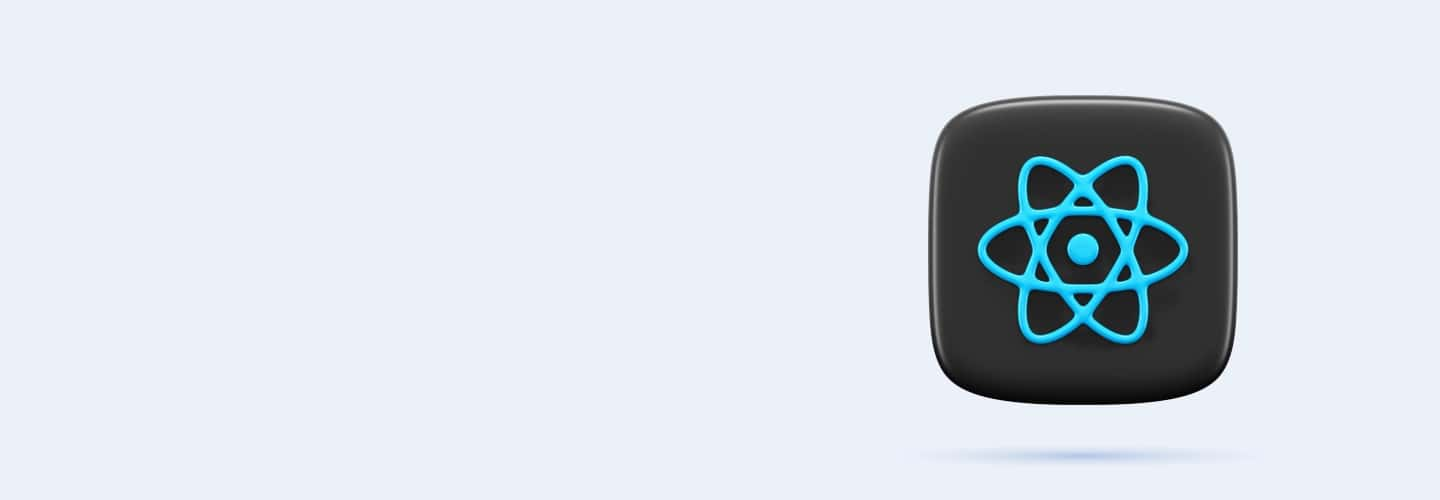
Q121
Q121 What could cause a React component wrapped in an HOC not to update as expected?
The HOC does not pass down props to the wrapped component
The wrapped component is not exporting correctly
The HOC is not connected to the Redux store
None of the above
Q122
Q122 How do you avoid namespace clashes in props when using multiple Higher-Order Components?
By using unique prop names across all HOCs
By nesting HOCs instead of composing them
By using React's built-in prop namespace feature
None of the above
Q123
Q123 What is the main purpose of custom hooks in React?
To interact with the DOM directly
To create more complex components
To reuse stateful logic between different components
To enhance performance
Q124
Q124 What are the benefits of using advanced hooks like useReducer and useContext together?
They allow for global state management without third-party libraries
They simplify the process of prop drilling
They provide a more performant solution to state management
Both A and B
Q125
Q125 What should you consider when creating a custom hook?
Its impact on the component's lifecycle
The types of components it will be used in
How it manages state and side effects
All of the above
Q126
Q126 How do you create a custom hook in React?
By adding a new method to the React object
By creating a function that starts with use and uses other hooks
By extending the React.Component class
None of the above
Q127
Q127 How can you optimize performance in a component that uses many custom hooks?
By using the useCallback and useMemo hooks
By using React.PureComponent
By minimizing the number of state updates
Both A and C
Q128
Q128 What is a common mistake to avoid when using custom hooks?
Using them inside conditional statements
Defining them inside components
Calling them outside of functional components
All of the above
Q129
Q129 How do you troubleshoot a memory leak in a component caused by a custom hook?
By ensuring cleanup functions are defined in effects
By reducing the number of state variables
By avoiding the use of state and effects altogether
None of the above
Q130
Q130 What is the purpose of using React.memo in a functional component?
To memorize the component's state
To prevent a component from re-rendering unless its props have changed
To cache the component's output
None of the above
Q131
Q131 How does the useCallback hook contribute to performance optimization in React components?
By caching functions to prevent unnecessary re-creations
By reducing the bundle size
By accelerating the rendering process
None of the above
Q132
Q132 What is the main benefit of code splitting in React?
To reduce the initial loading time of the application
To simplify the development process
To improve the runtime performance
None of the above
Q133
Q133 How can you implement lazy loading of components in React?
Using React.lazy and Suspense
Using the lazy function in JavaScript
Deferring the component rendering with setTimeout
None of the above
Q134
Q134 In what scenario is using shouldComponentUpdate or React.memo not effective for preventing unnecessary re-renders?
When the component always receives new object references as props
When the component is stateless
When the component has no children
None of the above
Q135
Q135 How do you identify and address unnecessary re-renders in a React application?
Using the React DevTools Profiler
Using console logs in the render method
Reviewing the code for inefficient patterns
All of the above
Q136
Q136 What can cause a memory leak in a React component, and how do you fix it?
Not cleaning up event listeners or subscriptions in useEffect
Using outdated libraries
Incorrect use of state
Both A and B
Q137
Q137 What is the primary benefit of using TypeScript with React?
Improved runtime performance
Automatic type conversion
Static type checking and easier error detection
None of the above
Q138
Q138 How does TypeScript enhance the development of large-scale React applications?
By reducing the size of the final bundle
By providing better integration with third-party libraries
By offering enhanced readability and maintainability through type annotations and interfaces
By increasing rendering speed
Q139
Q139 In TypeScript, how can you define the types of props in a React functional component?
Using type assertions
Using interfaces or types to define the shape of props
Using generic types
Both B and C
Q140
Q140 How do you define state in a TypeScript class component?
state: StateType = initialState; inside the class
this.state = { ... } inside the constructor
Both A and B
None of the above
Q141
Q141 How do you type a React component with generic props in TypeScript?
By using the React.ComponentType with generics
By defining a generic interface for props and using it in the component
By using the React.FC type with a generic parameter
Both A and C
Q142
Q142 What is a common issue when using TypeScript with React and how can it be resolved?
Type errors due to incorrect prop types, resolved by ensuring prop types match the expected interface or type
Difficulty in setting up the TypeScript environment, resolved by configuring the TypeScript compiler
Both A and B
None of the above
Q143
Q143 How can you troubleshoot TypeScript errors related to the context API in a React application?
By ensuring the context value matches the type defined in the Context creation
By using any type for all context values
By avoiding the use of context in TypeScript applications
None of the above
Q144
Q144 What is the main purpose of using Jest in React applications?
For end-to-end testing
For performance testing
For unit and integration testing
For style testing
Q145
Q145 How does React Testing Library differ from enzyme in testing React components?
React Testing Library focuses on internal component state and methods, while enzyme focuses on rendering components
React Testing Library focuses on rendering components and user interactions, while enzyme focuses on internal component state and methods
React Testing Library is only for functional components, while enzyme is for both functional and class components
None of the above
Q146
Q146 What is a best practice when writing tests for React components using React Testing Library?
To frequently use snapshot testing for component output
To test internal component states and methods
To focus on testing the component as users would interact with it
To mock all component props and external modules
Q147
Q147 How do you mock a module or function in Jest?
Using jest.fn() to create a mock function
Using jest.mock() to mock an entire module
Both A and B
None of the above
Q148
Q148 How do you test a component that fetches data from an API using React Testing Library?
By making real API calls during the test
By mocking the fetch function and simulating a response
By testing only the loading state
None of the above
Q149
Q149 What common issue should you be aware of when testing asynchronous behavior in React components?
Tests completing before asynchronous operations
Ignoring error handling in asynchronous operations
Both A and B
None of the above
Q150
Q150 How do you identify and resolve issues related to event handling in tests using React Testing Library?
By ensuring the correct event types and payloads are used
By manually triggering events in the component
Both A and B
None of the above