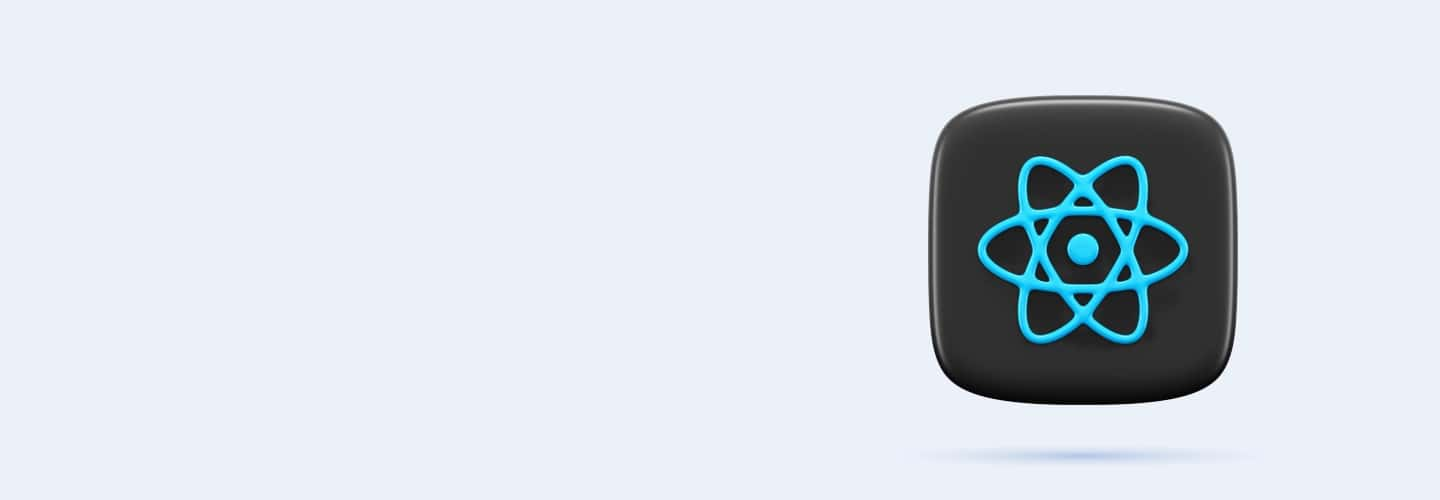
Q91
Q91 What is the difference between the and
There is no difference
None of the above
Q92
Q92 How do you define a route parameter in React Router?
Q93
Q93 How can you programmatically navigate to a new route in React Router?
Using this.props.history.push('/new-route')
Using navigate('/new-route')
Using this.context.router.push('/new-route')
None of the above
Q94
Q94 What is the use of the exact prop in a
To exactly match the component's CSS class
To ensure that the route only matches the exact path without any sub-routes
To make the component render only once
None of the above
Q95
Q95 Identify the issue with this route definition:
in a React Router application.
No issue
The route should have an exact prop
The component should be defined inline
The path should be more specific
Q96
Q96 What might cause a not to render the expected component in React Router?
The is outside a
The corresponding
Both of the above
None of the above
Q97
Q97 What is the purpose of the useState hook in React?
To connect a React component to an external state management library
To manage state in a class component
To manage local state in a functional component
To store global state across the application
Q98
Q98 When is the useEffect hook run in a React component?
After every render of the component, including the first render
Only when the component mounts
Only when the component updates
None of the above
Q99
Q99 What does the useEffect hook replace in class components?
componentDidMount and componentDidUpdate methods
componentDidMount, componentDidUpdate, and componentWillUnmount methods
componentWillUnmount method
None of the above
Q100
Q100 What is the correct way to conditionally run an effect with the useEffect hook?
By using an if statement inside the useEffect hook
By setting a specific condition in the dependency array
By using a ternary operator inside the useEffect hook
None of the above
Q101
Q101 What does the useCallback hook do?
Memoizes a callback function to prevent unnecessary re-renders
Creates a new function every time the component renders
Runs a function in the background
None of the above
Q102
Q102 How do custom hooks enhance the functionality of React components?
By adding new features to React that are not available in built-in hooks
By allowing state and lifecycle features to be reused across multiple components
By replacing the need for class components
None of the above
Q103
Q103 How do you initialize state with the useState hook?
useState(initialState)
useState()
useState({})
useState(() => initialState)
Q104
Q104 What is the correct way to update state based on the previous state using useState?
setState(prevState => prevState + 1)
setState(state + 1)
setState(prevState => { return prevState + 1; })
setState({ ...state, count: state.count + 1 })
Q105
Q105 How can you implement a fetch request with useEffect that updates the state when the data is retrieved?
By putting the fetch request inside the useEffect hook and updating the state in the .then() callback
By using async/await inside the useEffect hook
By creating a separate async function outside of useEffect and calling it inside useEffect
All of the above
Q106
Q106 Identify the issue in this useState usage:
const [count, setCount] = useState(0); setCount(count + 1);
The state update should be inside a component or effect
The initial state is incorrectly set
The setCount function is used incorrectly
No issue
Q107
Q107 What could cause an infinite loop in a component using useEffect?
Omitting the dependency array
Including a state variable that constantly changes within the dependency array
Both of the above
None of the above
Q108
Q108 How do you prevent a memory leak in a component that subscribes to an external data source using useEffect?
By unsubscribing from the data source in the return function of useEffect
By using the useMemo hook
By reducing the number of re-renders
None of the above
Q109
Q109 What is the primary use of the Context API in React?
To manage global state across the entire application
To enhance performance
To handle form validations
To create high-order components
Q110
Q110 How does the Context API help in avoiding "prop drilling"?
By using global variables accessible by all components
By allowing components to subscribe to context changes directly
By automatically passing props to all child components
None of the above
Q111
Q111 What is a potential drawback of using the Context API excessively?
Increased memory usage
Difficulty in managing and tracking state changes
Slower component re-renders
All of the above
Q112
Q112 How do you consume a context value in a functional component?
Using Context.Consumer
Using the useContext hook
Using this.context
None of the above
Q113
Q113 How can you dynamically change the value of a context in a component tree?
By modifying the context object directly
By updating the state that's passed to the context provider's value prop
By using a reducer with the context
None of the above
Q114
Q114 What might be the issue if a component is not receiving the expected context value?
The component is not wrapped with the Context.Provider
The context value is not updated correctly
Both of the above
None of the above
Q115
Q115 How do you address a performance issue caused by unnecessary re-renders in components consuming a frequently updated context?
Using the shouldComponentUpdate lifecycle method
Using the React.memo higher-order component
Splitting the context into multiple smaller contexts
All of the above
Q116
Q116 What is a Higher-Order Component (HOC) in React?
A component that renders other components
A component that is rendered by other components
A function that takes a component and returns a new component
None of the above
Q117
Q117 Why are Higher-Order Components useful in React?
They make components more reusable
They allow components to inherit from multiple sources
They increase the performance of components
None of the above
Q118
Q118 What is a key consideration when using Higher-Order Components?
They should be used for managing state
They should not alter the component's signature
They are the only way to share logic between components
None of the above
Q119
Q119 How do you apply a Higher-Order Component to a React component?
Q120
Q120 What is the correct way to pass additional arguments to a Higher-Order Component in React?