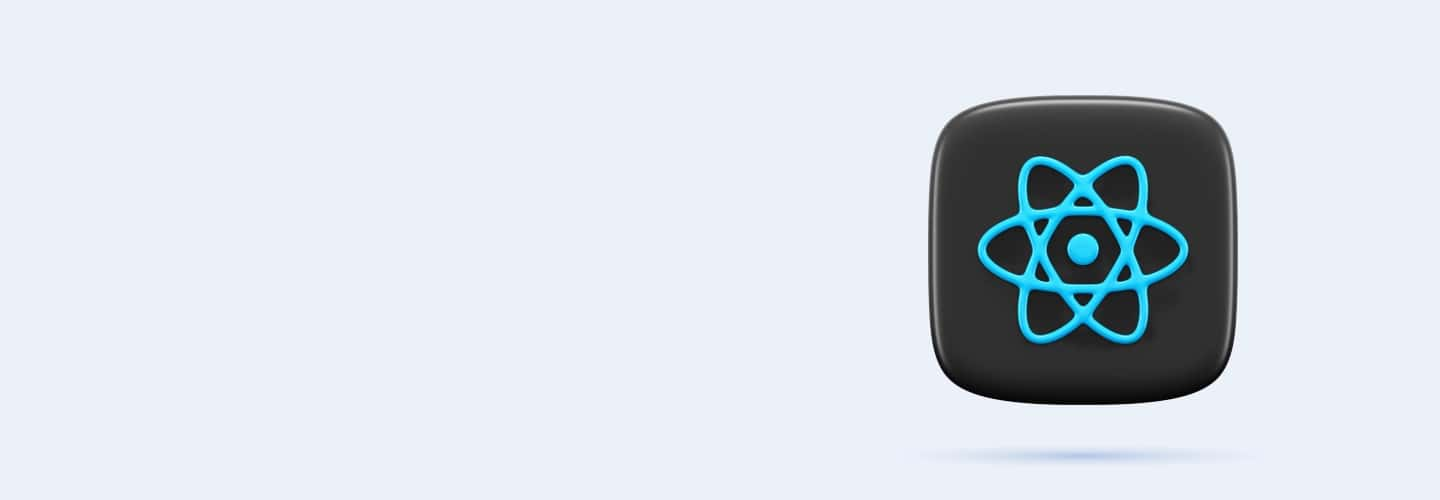
Q61
Q61 What is a controlled component in React?
A component that controls all the forms in the app
A component whose state is controlled by React
A component that cannot be modified
None of the above
Q62
Q62 In React, how do you handle form submission?
Using a submit button outside the form
By manually updating the DOM
Using an onSubmit event handler in the form element
None of the above
Q63
Q63 How do you collect form input values in React?
Using refs to directly access the DOM element
By storing the values in the state on every change
Using document.getElementById()
None of the above
Q64
Q64 What is the main difference between controlled and uncontrolled components in React?
Controlled components do not use state, while uncontrolled components do
Controlled components use refs, while uncontrolled components do not
Controlled components manage their own state, while uncontrolled components store their state in the DOM
None of the above
Q65
Q65 How would you create a basic text input field in a React component?
Q66
Q66 What is the correct way to handle a form input change in React?
onChange={(event) => this.setState({value: event.target.value})}
onInput={(event) => this.setState({value: event.target.value})}
onChange={this.updateValue}
onInputChange={(event) => this.setState({value: event})}
Q67
Q67 Identify the mistake in this form input:
The value attribute is incorrect
The type attribute is incorrect
The onchange event handler should be onChange
No mistake
Q68
Q68 What's wrong with this form submission handling?
onSubmit should be onsubmit
The form should have an action attribute
The form is missing an onChange handler for the input
No issue
Q69
Q69 What does "lifting state up" mean in React?
Moving state to a child component for shared access
Moving state to a parent component for shared access
Deleting state
None of the above
Q70
Q70 Why is lifting state up beneficial in React applications?
It enhances the performance of the application
It allows child components to modify each other's state directly
It enables multiple components to share and synchronize state changes
It simplifies the debugging process
Q71
Q71 In the context of lifting state up, what is a common pattern for two-way data binding between parent and child components?
Using refs to update parent state from the child
Using a callback function passed from parent to child
Relying on global variables
None of the above
Q72
Q72 What is a potential downside of lifting state up in a large React application?
Increased rendering time for all components
Difficulty in managing state across distant components
Increased risk of security vulnerabilities
None of the above
Q73
Q73 How do you lift state up from a child component to a parent component?
By using global state management libraries like Redux
By passing a state setter function from parent to child
By using React context
None of the above
Q74
Q74 What is the correct way to update the parent's state from a child component?
Directly modify the parent's state
Use a callback function provided by the parent
Send a request to the parent via an event bus
None of the above
Q75
Q75 Identify the problem in this code:
class Parent extends React.Component { updateState() { /.../ } render() { return
updateState should be bound to this in the constructor
updateState is missing parameters
Child component should not receive onChange prop
No issue
Q76
Q76 What's the issue with this lifted state approach?
class Parent extends React.Component {
constructor(props) {
super(props);
this.state = { value: '' };
} render() {
return <Child value={this.state.value} onChange={value => this.setState({value})} />; } }
onChange handler in Child is not implemented correctly
The value prop in Child is redundant
setState should not be used in the render method
No issue
Q77
Q77 In React, what is favored for reusing component logic?
Inheritance
Composition
Both equally
Neither
Q78
Q78 What is a key advantage of composition over inheritance in React?
Composition allows you to use multiple inheritances
Composition provides a clearer component hierarchy
Composition is easier to debug
Inheritance is more performant
Q79
Q79 How does React achieve code reuse with composition?
By inheriting methods from parent components
By rendering props children
By using higher-order components
By using React hooks
Q80
Q80 What is an example of composition in React?
Extending one component from another
Using state and lifecycle methods in class components
Passing components as props to other components
Using context API
Q81
Q81 Why might you choose composition over inheritance when designing React components?
Inheritance is not supported in React
Composition allows for easier code refactoring and maintenance
Composition makes components more complex
None of the above
Q82
Q82 Which React code snippet demonstrates composition?
Q83
Q83 How can you use composition to pass content into a component in React?
By using a prop to pass a function
By passing content as a child between component tags
By extending the component class
By using React context
Q84
Q84 What is a higher-order component (HOC) in React, and how does it relate to composition?
A component that inherits from another component
A function that takes a component and returns a new component
A class that renders multiple components
None of the above
Q85
Q85 Identify the error in this composition approach:
The
The
There is no error in this approach
The components should be connected using inheritance
Q86
Q86 What's wrong with using inheritance to extend a React component like this:
class EnhancedComponent extends BaseComponent { /.../ }?
React does not support class inheritance
Inheritance can lead to a more complex and less predictable component structure
BaseComponent methods can't be overridden
There is no issue
Q87
Q87 What is React Router used for in a React application?
Managing state within components
Routing between different components based on URL
Styling components
None of the above
Q88
Q88 Which component in React Router is used to configure a route?
Q89
Q89 What does the
It switches the application's theme
It provides conditional logic for rendering components
It toggles between two routes
None of the above
Q90
Q90 How do you navigate to a different URL using React Router?
Using the navigate() function
Using the
Using the component
All of the above