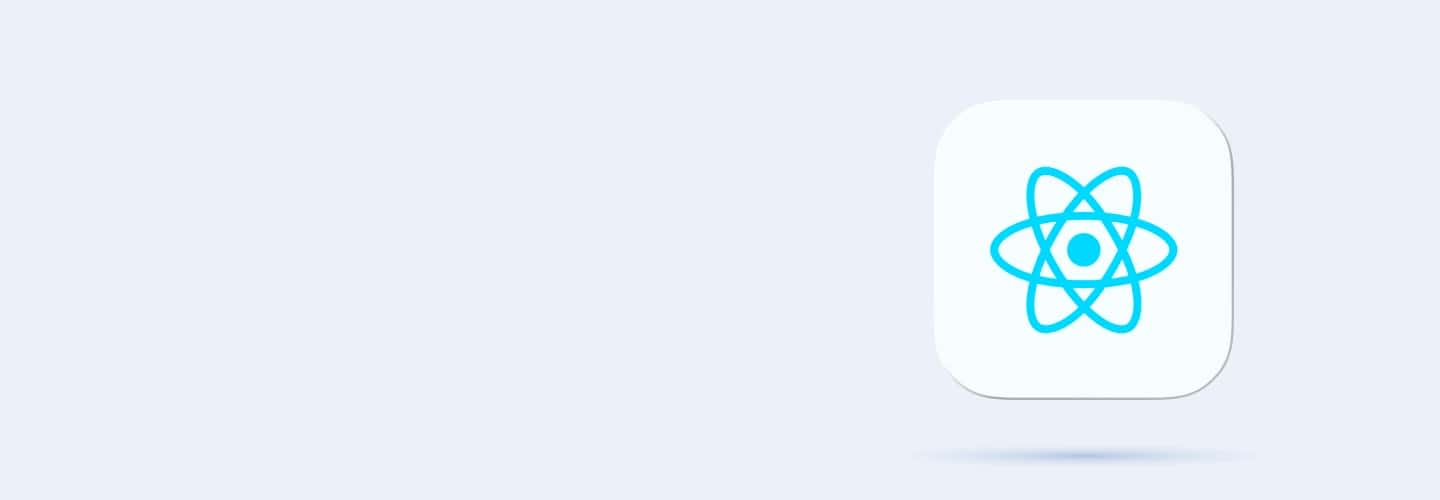
Q121
Q121 What could cause an “Out of Memory” error in React Native?
Too many images in memory
Excessive event listeners
Rendering too many components at once
Large data arrays in state
Q122
Q122 What is the purpose of native modules in React Native?
To access device-specific functionality
To improve JavaScript performance
To replace state management
To handle navigation
Q123
Q123 In which programming languages can you write native modules for React Native?
Only Java
Java and Swift
Java, Kotlin, Objective-C, Swift
Only JavaScript
Q124
Q124 What is the role of the React Native bridge?
It connects JavaScript code to native modules
It speeds up rendering
It prevents memory leaks
It enables Redux
Q125
Q125 How do you export a native module in Android?
@ReactModule
@ReactNativeModule
@ReactMethod
@NativeModule
Q126
Q126 How do you create a native module in iOS for React Native?
Extend RCTBridgeModule and use RCT_EXPORT_METHOD
Use NativeModule.create()
Extend ReactModuleBridge
Write a SwiftModule class
Q127
Q127 What is the correct way to call a native module method in React Native?
NativeModules.MyModule.myMethod()
NativeModules.callMethod('MyModule')
import { MyModule } from 'react-native'
useNativeBridge('MyModule')
Q128
Q128 Why might a native module not be found in React Native?
The module was not linked properly
The module is only available on iOS
The JavaScript code is incorrect
React Native does not support native modules
Q129
Q129 Why might an Android native module fail to load in React Native?
The package is not registered in MainApplication.java
React Native does not support Android
The app requires Expo
The bridge is disabled
Q130
Q130 What is the primary purpose of Jest in React Native?
To test UI components
To handle API requests
To manage state
To optimize animations
Q131
Q131 How does Detox differ from Jest in React Native testing?
Detox is used for end-to-end testing, while Jest is for unit testing
Detox is used only for Android apps
Jest cannot test UI components
Detox is faster than Jest
Q132
Q132 What is the main advantage of using Detox for testing?
It can simulate real user interactions
It is built into React Native
It does not require a test runner
It can test JavaScript code directly
Q133
Q133 How do you mock an API request in Jest?
jest.mock('axios')
jest.apiMock('fetch')
mockAPI('axios')
jest.spyOnFetch()
Q134
Q134 What is the correct way to write a snapshot test in Jest?
expect(component).toMatchSnapshot()
testSnapshot(component)
expect(component).snapshotMatch()
jest.snapshot(component)
Q135
Q135 Why might a Jest test fail unexpectedly?
The test is not wrapped in act()
Jest is outdated
The app is not compiled
The component is missing prop validation
Q136
Q136 Why might Detox fail to launch the test app?
Emulator/simulator is not running
Jest is not installed
React Native does not support Detox
Detox does not work with functional components
Q137
Q137 What is the best way to store sensitive data in a React Native app?
AsyncStorage
Redux Store
SecureStore or Keychain
Local JSON file
Q138
Q138 Why should API keys not be stored in the source code of a React Native app?
API keys can be stolen if the app is decompiled
API keys are too large to store in the source code
API keys slow down performance
API keys require encryption before use
Q139
Q139 Which method helps prevent reverse engineering of a React Native app?
Obfuscating JavaScript code
Removing all third-party libraries
Blocking app updates
Using Redux for state management
Q140
Q140 How do you securely store authentication tokens in React Native?
AsyncStorage.setItem('token', value)
SecureStore.setItemAsync('token', value)
localStorage.setItem('token', value)
Redux.setToken('token', value)
Q141
Q141 How do you prevent network requests from being intercepted in React Native?
Use https connections
Disable JavaScript debugging
Use SSL pinning
Disable API logging
Q142
Q142 Why should JavaScript debugging be disabled in production apps?
Debugging slows down the app
Debugging exposes sensitive information
Debugging is not supported in production
Debugging breaks Redux state
Q143
Q143 Why might an app be vulnerable to code injection attacks?
Using eval() or setTimeout() with user input
Using Redux for state management
Using TypeScript instead of JavaScript
Using functional components
Q144
Q144 What is a major benefit of using React Native for a real-world application?
Faster development with a single codebase
Better performance than native apps
No need for testing
Automatic bug fixes
Q145
Q145 Why do some companies prefer native development over React Native?
React Native is difficult to learn
React Native does not support animations
Native development provides better performance for complex applications
React Native does not support APIs
Q146
Q146 What is a common challenge when scaling a React Native app?
Limited third-party libraries
Difficulty in managing state across multiple screens
Incompatibility with iOS devices
Slow compilation
Q147
Q147 What is an important consideration when handling large amounts of data in React Native?
Using FlatList for optimized rendering
Storing all data in Redux
Keeping data in component state
Using useEffect for UI updates
Q148
Q148 How do you improve app startup time in a large React Native application?
Lazy load non-essential components
Use Redux for all state management
Increase JavaScript bundle size
Disable native modules
Q149
Q149 What could cause performance issues in a production React Native app?
Excessive re-renders
Using useEffect too frequently
Unoptimized images
Large navigation stack
Q150
Q150 How can you detect memory leaks in a React Native app?
Using React Profiler
Manually checking state changes
Using Expo Debugger
Reducing state variables