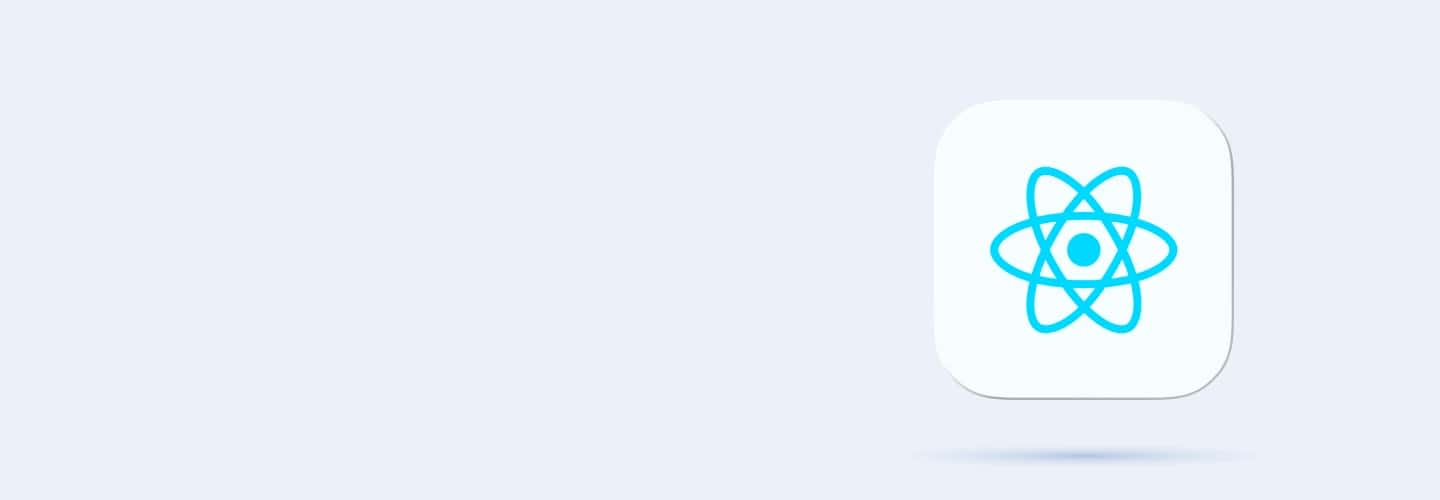
Q91
Q91 What could cause a CORS error when making an API request?
Missing headers in the request
The API server does not allow cross-origin requests
React Native does not support APIs
The request is too large
Q92
Q92 What is the primary purpose of Redux in React Native?
To manage global state
To handle navigation
To fetch API data
To style components
Q93
Q93 What are the main building blocks of Redux?
State, Actions, Reducers, Store
State, Props, Context, Store
Hooks, Reducers, Context, Dispatch
State, Functions, Components, API
Q94
Q94 What is the role of a reducer in Redux?
To handle API calls
To modify state based on actions
To fetch data from components
To render UI components
Q95
Q95 Which library is required to integrate Redux with React Native?
react-redux
redux-toolkit
redux-react-native
redux-state
Q96
Q96 How does the Context API differ from Redux?
Context API requires reducers, Redux does not
Redux provides a single store, while Context API provides multiple contexts
Redux is built into React Native, while Context API is not
There is no difference
Q97
Q97 What is the correct way to create a Redux store?
const store = createStore(reducer)
const store = createRedux(reducer)
const store = new ReduxStore(reducer)
const store = configureReduxStore(reducer)
Q98
Q98 How do you dispatch an action in Redux?
dispatch({ type: "ADD_ITEM", payload: item })
sendAction({ type: "ADD_ITEM", payload: item })
store.setState({ type: "ADD_ITEM" })
updateStore({ type: "ADD_ITEM" })
Q99
Q99 How do you access Redux state in a functional component?
useSelector(state => state.value)
useRedux(state => state.value)
store.getState().value
state.getRedux()
Q100
Q100 What is the purpose of Redux middleware?
To modify reducer functions
To handle async actions
To replace the Redux store
To automatically update UI
Q101
Q101 Why does a Redux reducer return undefined?
The initial state is missing
Reducers must return null
Redux does not allow reducers
Redux does not support state updates
Q102
Q102 What could cause a Redux action to not update state?
The action type is incorrect
Redux is not installed
Reducers cannot modify state
Actions cannot be dispatched in React Native
Q103
Q103 Why might useSelector not return updated state?
The reducer is not connected to the store
useSelector cannot read state
Redux does not work with functional components
Redux does not support hooks
Q104
Q104 What is the primary purpose of animations in React Native?
To improve user experience
To reduce memory usage
To prevent UI updates
To handle API calls
Q105
Q105 Which built-in API does React Native provide for animations?
ReactAnimation
Animated API
Lottie
React Motion
Q106
Q106 Which animation type provides the most natural movement?
timing
spring
decay
loop
Q107
Q107 What is the advantage of using the LayoutAnimation API?
Allows easy animations with minimal code
Improves network performance
Prevents UI re-renders
Requires manual frame updates
Q108
Q108 What is the correct way to create an animated value?
new Animated.Value(0)
Animated.createValue(0)
new Animation(0)
useAnimatedValue(0)
Q109
Q109 How do you animate a view's opacity using the Animated API?
Animated.timing(opacity, { toValue: 1, duration: 500 }).start()
Animated.fade(opacity, { duration: 500 })
opacity.animate({ to: 1, time: 500 })
setOpacity(1, { time: 500 })
Q110
Q110 How can you run multiple animations in parallel?
Animated.sequence([...])
Animated.parallel([...])
Animated.stagger([...])
Animated.together([...])
Q111
Q111 Why might an animation not work in React Native?
The animated value is not updated
Animations require Redux
Animations only work with state
React Native does not support animations
Q112
Q112 Why does LayoutAnimation not work properly on Android?
It requires enabling UI manager
It only works in React Native 0.70+
Animations are disabled in Android
React Native does not support animations on Android
Q113
Q113 What is the best tool to debug a React Native application?
React DevTools
Redux DevTools
Chrome Debugger
Flipper
Q114
Q114 What is a common cause of slow rendering in React Native?
Too many re-renders
Excessive use of setState
Inefficient component updates
Large images in memory
Q115
Q115 How can you prevent unnecessary re-renders in React Native?
Using React.memo
Using useEffect
Using console.log
Using setInterval
Q116
Q116 Which tool can be used to analyze React Native performance issues?
Flipper
Redux
Expo
Babel
Q117
Q117 How do you enable the performance monitor in React Native?
Debug > Performance Monitor in React DevTools
import Performance from 'react-native-performance'
Use useEffect
Modify App.js
Q118
Q118 What is the best way to optimize large lists in React Native?
Use ScrollView
Use FlatList with keyExtractor
Render all items at once
Use Redux
Q119
Q119 How do you prevent unnecessary renders in functional components?
Use React.memo
Use useState without updating state
Remove return statements
Use console.log
Q120
Q120 Why does a React Native app crash on startup without showing an error?
Corrupted node_modules folder
Incorrect import statements
Invalid Redux setup
Syntax errors in JavaScript code