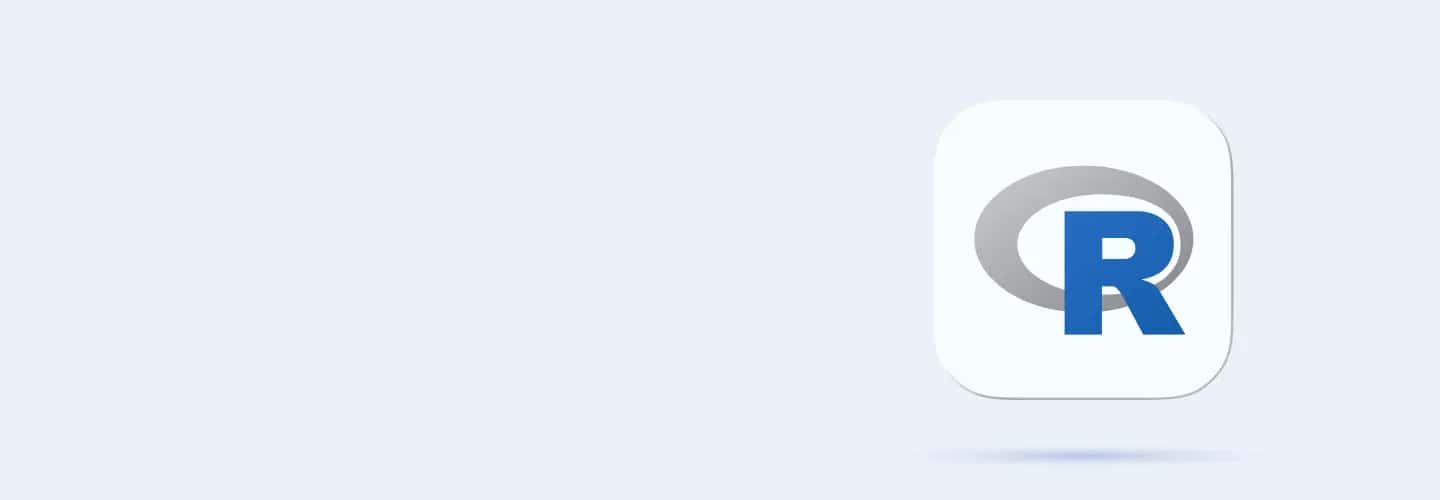
Q31
Q31 Which function is most appropriate for adding a new column to a data frame in R?
append()
insert()
$ operator
add()
Q32
Q32 If a vector operation returns unexpected 'NA' values, what is a possible cause?
Missing data in vector
Data type mismatch in operations
Incorrect function use
All elements are NA
Q33
Q33 What could be the issue if accessing an element beyond the length of a vector doesn't return an error but returns NA?
Vector is defined to have extra length
Vector indexing starts at 0
Vector contains explicit NA values
An error in R's internal handling
Q34
Q34 When merging two data frames, why might some entries appear as 'NA'?
Missing matches in key columns
Incorrect data types
Syntax errors in merge function
All columns are incompatible
Q35
Q35 Which control structure allows repeating a set of commands a fixed number of times in R?
while loop
for loop
if-else statement
repeat loop
Q36
Q36 In R, what does the if statement do?
Executes a loop
Tests a condition and executes an associated block of code
Defines a function
Imports a package
Q37
Q37 What is the use of the break statement in R?
Terminates a loop or switch statement
Starts a new iteration of a loop
Skips the current iteration of a loop
Exits the program
Q38
Q38 Which statement is used in R to skip the current iteration of a loop and begin the next one?
continue
next
skip
break
Q39
Q39 How can you execute a block of code multiple times conditionally in R?
Using the if loop
Using the for loop
Using the while loop
Using the do loop
Q40
Q40 What is the purpose of the else if statement in R?
To specify a new condition to test, if the first condition is false
To exit a loop
To define a function
To repeat a condition
Q41
Q41 Which syntax correctly starts a for loop in R that iterates from 1 to 10?
for (i = 1 to 10) { }
for i in 1:10 { }
for (i in 1:10) { }
for i from 1 to 10 { }
Q42
Q42 How do you write an infinite loop in R?
while (true) { }
while (1) { }
Both A and B are correct
Neither A nor B are correct
Q43
Q43 What does the following loop do?
for (i in 1:5) print(i^2)
Prints the squares of numbers 1 to 5
Prints numbers from 1 to 5
Counts from 1 to 5
None of the above
Q44
Q44 In a while loop, what happens if the condition never becomes false?
The loop terminates after a set number of iterations
The loop continues indefinitely
The loop skips iterations
The loop throws an error
Q45
Q45 What is a common mistake when using nested loops in R?
Mismanaging loop counters
Using the wrong loop type
Not nesting loops correctly
Forgetting to close brackets
Q46
Q46 If a loop is supposed to run but doesn't start, what could be a potential issue?
The loop condition is initially false
The loop is improperly nested
There are syntax errors in the loop code
All of the above
Q47
Q47 Which function in R is used to install packages from CRAN?
install.packages()
get.packages()
load.packages()
add.packages()
Q48
Q48 What is a primary use of the library() function in R?
Create new libraries
Delete existing libraries
Load installed packages into the R session
Update libraries
Q49
Q49 In R, what is the purpose of the source() function?
To load data from external sources
To execute R scripts from files
To generate reproducible random numbers
To integrate R with other programming languages
Q50
Q50 What does the apply() function do in R?
Modifies data frames
Applies a function over the margins of an array or matrix
Creates graphical plots
None of the above
Q51
Q51 Which statement best describes the role of user-defined functions in R?
They replace all basic functions of R
They are only for advanced users
They allow customization of tasks
They are not recommended
Q52
Q52 What is the function set.seed() used for in R?
To establish the starting point for loops
To control the reproducibility of random number generation
To seed the R environment with data
To initialize package settings
Q53
Q53 How do you create a simple function in R that takes one argument x and returns x squared?
def square(x) { return xx }
function(x) { return xx }
function(x) { xx }
square <- function(x) { x*x }
Q54
Q54 How do you ensure that a package is loaded only if it is already installed in R?
if (require(package)) library(package)
if (library(package))
if (installed.packages(package)) library(package)
if (require(package))
Q55
Q55 When defining a function in R, what is the effect of setting a default value for an argument?
It makes the argument optional
It makes the function faster
It limits the function to specific data types
It creates a constant
Q56
Q56 If a function fails because an argument is not correctly passed, what could be the probable issue?
Incorrect data type of argument
Missing function definition
Syntax error in function call
Incompatible library version
Q57
Q57 What should you check if a user-defined function in R isn't recognized in your script?
Whether the function is defined after it's called
Whether the package containing the function is loaded
Whether the script file is corrupted
Whether the R version is outdated
Q58
Q58 Which dplyr function is used to select columns from a data frame?
select()
filter()
arrange()
mutate()
Q59
Q59 What does the mutate() function in dplyr do?
Adds new variables to a data frame and preserves existing ones
Filters rows based on conditions
Sorts a data frame
Summarizes complex calculations
Q60
Q60 How does the filter() function in dplyr determine which rows to keep in a data frame?
By evaluating conditions set on columns
By matching patterns in row names
By comparing row indices
By data type of rows