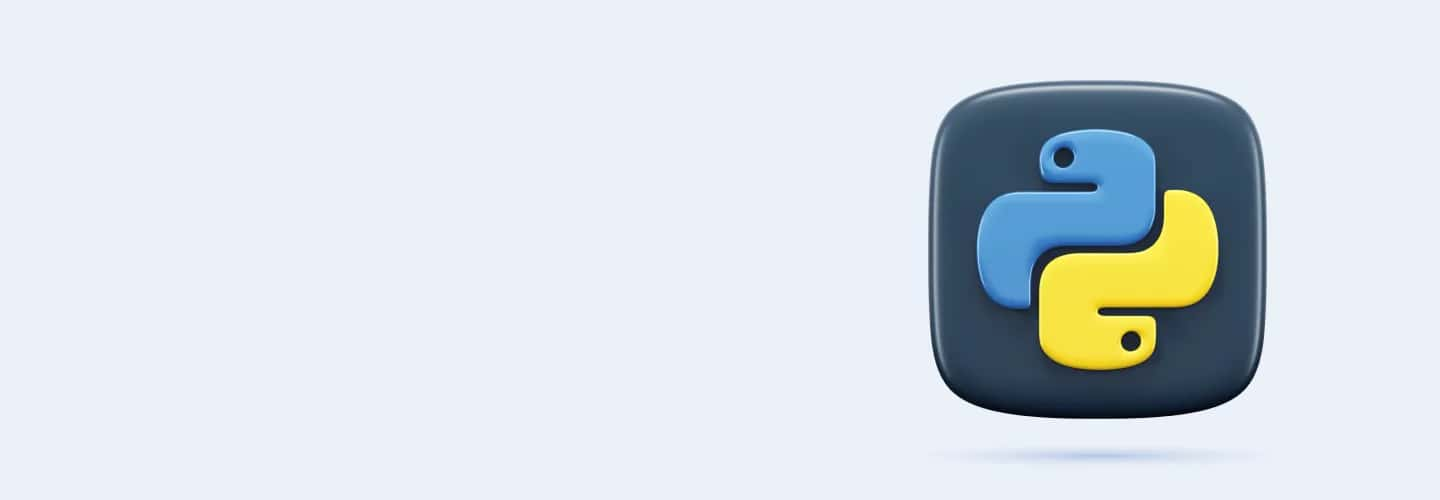
Q121
Q121 How does polymorphism enhance code reusability?
By allowing methods to perform different tasks based on the object
By using the same method in multiple classes
By changing the functionality of inherited methods
By encapsulating code in modules
Q122
Q122 Given a class Animal and a subclass Dog, which method demonstrates polymorphism?
Animal.speak() and Dog.speak() have different implementations
Dog has the same attributes as Animal
Dog uses a method from Animal without changing it
Animal has a method not present in Dog
Q123
Q123 Pseudocode:
Create a base class 'Shape', derived classes 'Circle', 'Square'; Implement method 'area' in both
Error, as 'area' cannot be implemented in 'Shape'
Circle' and 'Square' can't inherit from 'Shape'
area' method behaves differently in 'Circle' and 'Square'
'Shape' should not have an 'area' method
Q124
Q124 Identify the issue in this inheritance scenario:
class Bird(Animal):
def fly(self):
pass, class Penguin(Bird):
def fly(self):
print("Can't fly")
Birds don't have a 'fly' method by default
Penguins shouldn't inherit from 'Bird'
fly' method in 'Penguin' contradicts 'Bird'
No issue present
Q125
Q125 What is a decorator in Python?
A function that modifies the functionality of another function
A function that creates new functions
A syntax for defining anonymous functions
A tool for debugging functions
Q126
Q126 How do decorators contribute to cleaner code in Python?
By reducing the need for global variables
By providing a syntax for error handling
By allowing code reuse and adding functionality to existing code
By optimizing memory usage
Q127
Q127 What is a generator in Python?
A type of collection
A tool for creating iterators
A function that returns a single value
A module
Q128
Q128 How do generators differ from standard functions?
Generators can't take arguments
Generators don't return values
Generators yield values, maintaining state between each yield
Generators execute faster
Q129
Q129 What is the main difference between threading and multiprocessing in Python?
Threading is faster
Multiprocessing uses more memory
Threading involves parallel execution
Multiprocessing involves separate memory spaces for each process
Q130
Q130 When should you choose multiprocessing over threading in Python?
For I/O-bound tasks
For quick tasks
For CPU-bound tasks
When memory usage is a concern
Q131
Q131 What is a regular expression used for in Python?
Error handling
Data serialization
Pattern matching and text manipulation
Memory management
Q132
Q132 Which module in Python provides support for regular expressions?
sys
re
os
json
Q133
Q133 What does the regular expression ^a...s$ match?
Any seven-letter string starting with 'a' and ending with 's'
Any string starting with 'a' and ending with 's'
Any five-letter string starting with 'a' and ending with 's'
Any string containing 'a' and 's'
Q134
Q134 What will the following Python code return?
re.search(r"\d{3}", "The code 123 is valid")
Q135
Q135 Identify the issue in this regex:
re.findall(r"[A-Z+]", "Python+C+Java")
Incorrect use of escape character
Wrong pattern for matching uppercase letters
'+' should not be escaped
No issue
Q136
Q136 What is JSON primarily used for in Python?
Web development
Data serialization and communication between different systems
Numeric computations
File compression
Q137
Q137 In Python, which module is used to work with CSV files?
csv
json
excel
fileio
Q138
Q138 When working with Excel files in Python, which library is commonly used to read and write .xlsx files?
Pandas
Numpy
OpenPyXL
CSV
Q139
Q139 What does the following code do?
import json; json.loads('{"name": "John", "age": 30}')
Creates a JSON file
Parses a JSON string into a Python dictionary
Serializes a Python dictionary to JSON
Throws an error
Q140
Q140 Identify the error in this code for reading a CSV file:
import csv;
reader = csv.reader(open('file.csv', 'wb'))
The file mode should be 'rb', not 'wb'
csv.reader does not take a file object
The file 'file.csv' does not exist
Incorrect use of the csv module
Q141
Q141 What is the purpose of a virtual environment in Python programming?
Version control
To isolate project-specific dependencies
Web development
Data analysis
Q142
Q142 Which tool is used to install Python packages?
git
virtualenv
pip
setuptools
Q143
Q143 What is the key benefit of using requirements.txt in a Python project?
For documenting the code
To list all the packages and their versions used in the project
To improve the performance of the project
For version control
Q144
Q144 What is the primary use of the OS module in Python?
Networking
Web development
Interacting with the operating system
Data analysis
Q145
Q145 Which function in the OS module is commonly used to change the current working directory?
os.change_directory()
os.chdir()
os.setcwd()
os.update_directory()
Q146
Q146 How does the os.path.join() function benefit file path construction?
Increases file access speed
Ensures correct path format for the operating system
Encrypts the file path
Splits the file path into components
Q147
Q147 What does os.listdir('.') do?
Lists all Python files in the current directory
Creates a new directory
Lists all files and directories in the current directory
Deletes the current directory
Q148
Q148 Identify the error in this code:
import os;
os.rmdir('mydir') assuming 'mydir' contains files and subfolders.
Incorrect module imported
os.rmdir() cannot delete non-empty directories
Wrong syntax for removing directories
No error
Q149
Q149 What is PEP in the context of Python?
A Python enhancement proposal
A Python error protocol
A Python environment path
A Python executable program
Q150
Q150 Why is PEP 8 important in Python development?
It provides guidelines for error handling
It outlines the standard coding conventions
It defines the core functionality of Python
It lists Python's reserved words