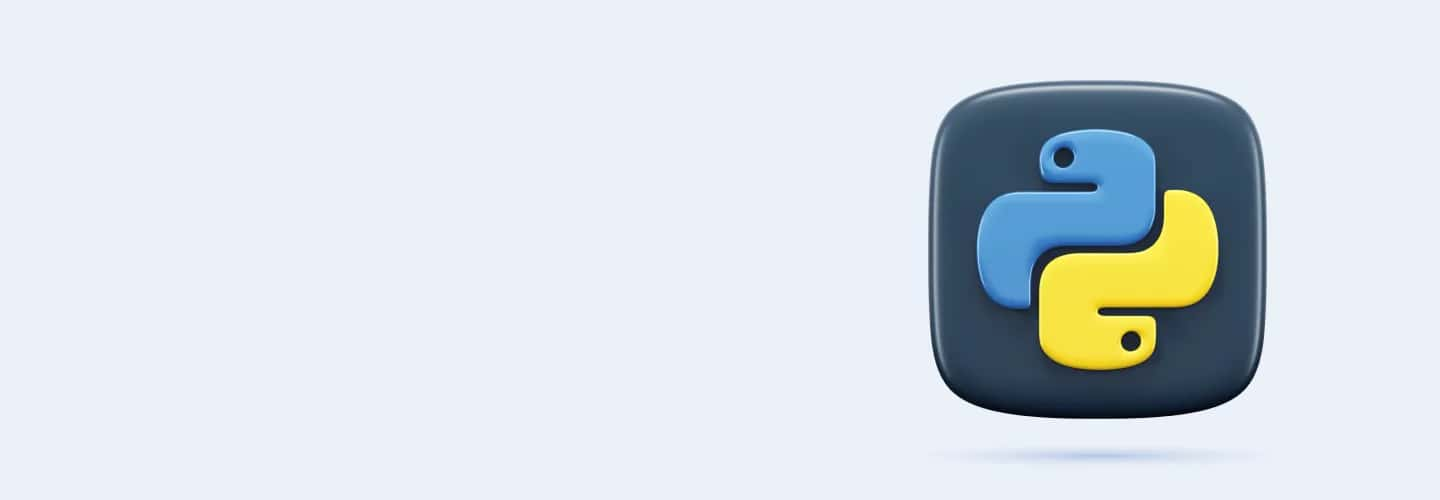
Q91
Q91 What is the result of this code?
f = open('file.txt', 'r');
print(f.readline())
Prints entire file
Prints the first line of file.txt
Error
Prints 'file.txt'
Q92
Q92 Pseudocode:
Open 'data.txt', read each line, print each line
Prints name of the file
Prints contents of data.txt
Error
No output
Q93
Q93 Identify the error:
file = open('data.txt', 'r');
data = file.read();
file.close()
File not closed properly
Incorrect mode for reading
No error
Error in file.read()
Q94
Q94 Find the mistake:
with open('data.txt', 'r') as file:
data = file.readlines();
print(data[2])
Wrong function used
Error in print statement
Index error
No error
Q95
Q95 In Python, what is the purpose of the try statement?
To test a block of code for errors
To execute code regardless of errors
To generate an error
To declare variables
Q96
Q96 Which exception is raised by Python when a file you try to open does not exist?
FileNotFoundError
IOError
OSError
ValueError
Q97
Q97 When is the else clause in a try-except block executed?
Always after the try block
When no exceptions are raised
When an exception is handled
It is never executed
Q98
Q98 What will be the output of the following code?
try: x = 1 / 0 except ZeroDivisionError:
print("Error")
0
1
Error
No output
Q99
Q99 Pseudocode:
Try to open a file, read its contents;
if file not found,
print error message
File contents are displayed
Error message is printed
No output
File is created and then read
Q100
Q100 Identify the error in this code:
try:
x = 1 / 0
except:
print("An error occurred")
Division by zero is not handled properly
Generic exception should not be used
Syntax error in try statement
No error
Q101
Q101 Find the mistake: try:
x = 1 / "0"
except ValueError:
print("Type error occurred")
Wrong exception type is caught
Should be ZeroDivisionError
It will raise a TypeError
No error
Q102
Q102 What is a list comprehension in Python?
A concise way to create lists
A method to iterate through lists
A type of Python comprehension
A special function for lists
Q103
Q103 What is the key difference between list comprehensions and generator expressions?
The syntax used
The type of brackets used
List comprehensions produce lists, while generator expressions produce generators
Execution speed
Q104
Q104 What will this list comprehension produce:
[x**2 for x in range(5)]
[0, 1, 2, 3, 4]
[1, 4, 9, 16, 25]
[0, 1, 4, 9, 16]
[1, 2, 3, 4, 5]
Q105
Q105 What does the following dictionary comprehension do?
{x: x*x for x in range(5)
if x%2 == 0}
Squares of all numbers from 0 to 4
Squares of odd numbers from 0 to 4
Squares of even numbers from 0 to 4
Cubes of even numbers from 0 to 4
Q106
Q106 Pseudocode:
Create a list of lowercase letters from a list of mixed case letters, 'A', 'b', 'C', 'd', 'E'
['A', 'b', 'C', 'd', 'E']
['a', 'b', 'c', 'd', 'e']
['A', 'C', 'E']
['b', 'd']
Q107
Q107 Identify the error in this code:
[x for x in range(10)
if x%2 = 0]
Syntax error in range function
Incorrect use of assignment operator
List comprehension is incorrectly formed
No error
Q108
Q108 What is a lambda expression in Python?
A one-time anonymous function
A named, reusable function
A loop structure
A data type
Q109
Q109 How is a lambda function different from a regular function defined using def in Python?
Lambda can only have one expression
Lambda can return multiple values
Lambda functions are faster
Lambda functions can't have arguments
Q110
Q110 What is the output of the following lambda expression?
(lambda x, y: x * y)(3, 4)
7
12
0
SyntaxError
Q111
Q111 Pseudocode:
Define a lambda to square a number, use it to get the square of 5
Returns 25
Returns 10
Syntax error
No output
Q112
Q112 Identify the error in this lambda expression:
lambda x, y:
if x > y
return x
else
return y
Incorrect syntax for if-else
Lambda function cannot use conditional
Lambda cannot return values
No error
Q113
Q113 What is a class in Python?
A blueprint for creating objects
A function inside an object
A variable inside an object
A specific object instance
Q114
Q114 In Python, what is 'self' in a class method?
A variable that holds the class name
A reference to the class itself
A reference to the instance that calls the method
A placeholder for future arguments
Q115
Q115 How is a class attribute different from an instance attribute in Python?
Class attributes are shared by all instances
Instance attributes can't be changed
Class attributes can't be accessed by instances
No difference
Q116
Q116 What is polymorphism in the context of object-oriented programming?
Changing the functionality of methods in subclasses
Creating multiple classes that inherit from a single class
The ability of different object types to be accessed through the same interface
Splitting a class into multiple smaller classes
Q117
Q117 Given the class definition:
class Dog:
def init(self, name):
self.name = name,
what does Dog('Buddy').name return?
Dog
name
Buddy
An error occurs
Q118
Q118 Pseudocode:
Define a class 'Car'
with a method 'drive';
Create an instance of 'Car';
Call 'drive' method
Error as 'drive' method is undefined
The 'drive' method of the 'Car' instance is executed
Nothing as 'Car' has no attributes
A new 'Car' object is created
Q119
Q119 Identify the error in this code:
class Animal:
def init(self, name):
name = name
Incorrect use of the constructor
name should be 'self.name'
The class should have more methods
No error
Q120
Q120 What is inheritance in object-oriented programming?
Sharing data between classes
Copying methods from one class to another
Deriving new classes from existing classes
Protecting data within a class