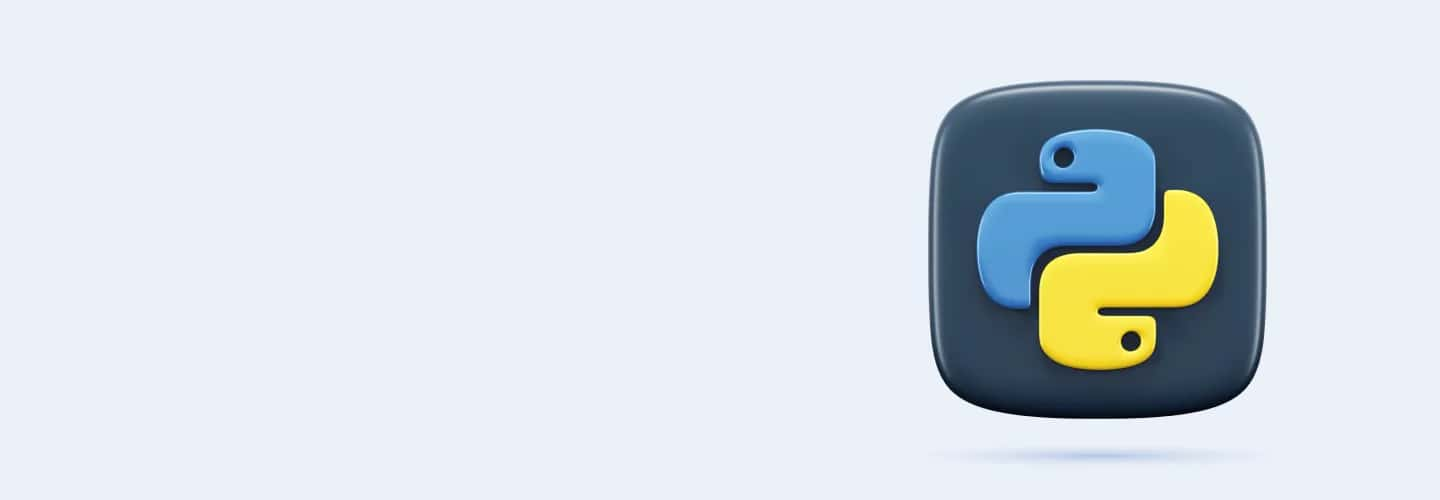
Q61
Q61 What is the result of the following code?
myList = [1, 2, 3];
myList[1] = 4;
print(myList)
[1, 2, 3]
[1, 4, 3]
Error
[4, 2, 3]
Q62
Q62 Pseudocode:
Initialize list with values [1,2,3];
Add 4 to the end;
Print list
Prints [1, 2, 3]
Prints [1, 2, 3, 4]
Error
Prints [4, 1, 2, 3]
Q63
Q63 Pseudocode:
Given a tuple (1,2,3),
print each element multiplied by 2
Prints 2, 4, 6
Prints 1, 2, 3
Error
No output
Q64
Q64 Identify the error:
myList = [1, 2, 3);
myList.append(4)
Syntax error with the list declaration
append() method is used incorrectly
No error
Lists cannot contain integers
Q65
Q65 Find the mistake:
myTuple = (1, 2, 3);
myTuple[1] = 4
Tuples are immutable
Wrong index used
Syntax error in tuple declaration
myTuple should be a list
Q66
Q66 What is a dictionary in Python?
An ordered collection of elements
A mutable collection of key-value pairs
An immutable collection of items
A collection of unique elements
Q67
Q67 How do you access the value associated with a key 'k' in a dictionary 'd'?
d('k')
d[k]
d.get('k')
All of the above
Q68
Q68 What happens if you try to access a non-existent key 'k' in a dictionary 'd' using d[k]?
Returns None
Returns an empty string
Raises a KeyError
Creates a new key 'k' with value None
Q69
Q69 How can you remove a key-value pair from a dictionary?
Using the del statement
Using the remove() method
Using the pop() method
Both A and C
Q70
Q70 In Python 3.7 and later, how are elements in a dictionary ordered?
By the order they were added
In ascending order of keys
In descending order of keys
Randomly
Q71
Q71 What is the output of the following code?
d = {'a': 1, 'b': 2};
print(d['b'])
1
2
b
KeyError
Q72
Q72 What will be the result of the following code?
d = {'a': 1, 'b': 2};
d['c'] = 3;
print(len(d))
2
3
Error
1
Q73
Q73 Pseudocode:
Initialize dictionary with {'a': 1, 'b': 2};
Update 'b' value to 3;
Print dictionary
Prints {'a': 1, 'b': 2}
Prints {'a': 1, 'b': 3}
Error
No output
Q74
Q74 Pseudocode:
Given a dictionary,
print each key-value pair
Prints keys only
Prints values only
Prints key-value pairs
Error
Q75
Q75 Identify the error:
d = {['first']: 1, ['second']: 2}
Syntax error with the dictionary
Lists can't be dictionary keys
No error
Dictionary keys must be strings
Q76
Q76 Find the mistake:
d = {'a': 1, 'b': 2};
print(d.get('c', 'Not Found'))
Syntax error in the dictionary
get() method used incorrectly
Key 'c' doesn't exist
No error
Q77
Q77 What is a function in Python?
A variable
A module
A block of code
An operator
Q78
Q78 In Python, what is a module?
A data type
A built-in function
A file with definitions
A code block
Q79
Q79 How do you import a module in Python?
using the import keyword
using the include keyword
using the require keyword
using the module keyword
Q80
Q80 What is the difference between arguments and parameters in Python functions?
No difference
Parameters define, arguments call
Arguments define, parameters call
Based on data type
Q81
Q81 What will the following function return:
def example():
return 5+5
5+5
10
0
None
Q82
Q82 What is the output of this code:
def add(x, y):
return x + y;
print(add(3, 4))
3
4
7
Error
Q83
Q83 Pseudocode:
Define a function that multiplies two numbers and returns the result
Returns sum
Returns product
No return
Syntax error
Q84
Q84 Pseudocode:
Function that checks if a number is positive, negative, or zero
Prints type of number
Returns type of number
No output
Error
Q85
Q85 Identify the error:
def my_func(x, y):
return x + y;
my_func(1)
Missing argument
Syntax error
Logic error
No error
Q86
Q86 Find the mistake:
import math;
print(math.sqr(4))
Incorrect module
Incorrect function name
Wrong number of arguments
No error
Q87
Q87 What is the purpose of the open() function in Python?
To read data from URL
To open a new Python file
To open a file
To create a Python module
Q88
Q88 Which file mode in Python allows you to read and write to a file?
r+
w+
a+
rb+
Q89
Q89 When using with open(file) as f, what does with do?
Ensures file is saved
Automatically closes the file
Opens file in write mode
Reads entire file
Q90
Q90 What does the following code do?
with open('file.txt', 'w') as file:
file.write("Hello World")
Reads from file.txt
Writes "Hello World" to file.txt
Deletes file.txt
Creates a new file