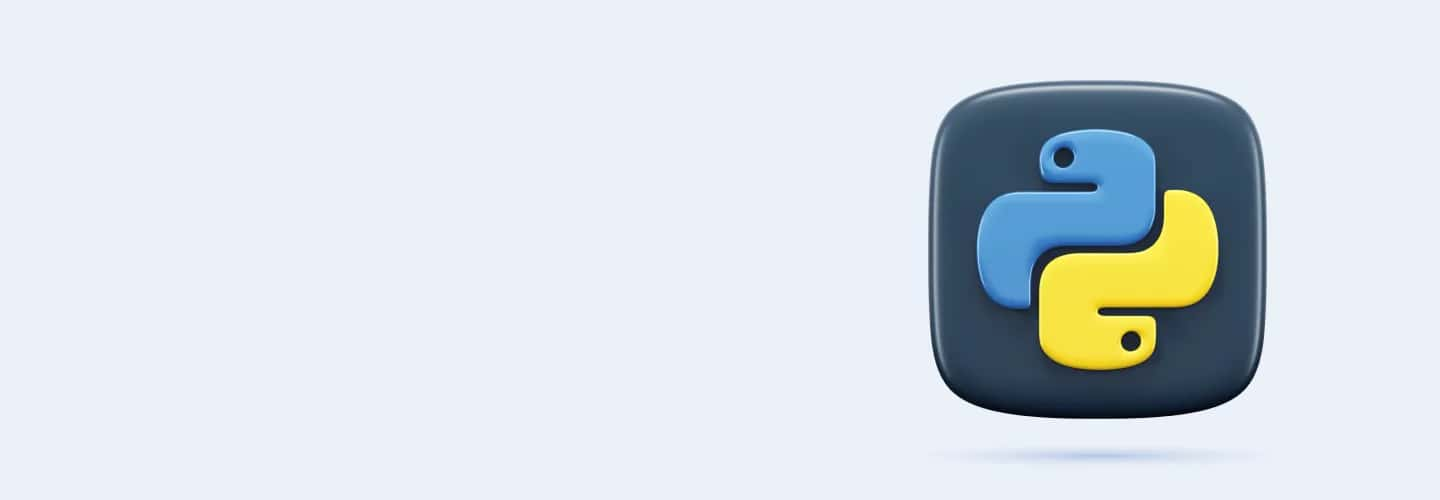
Q31
Q31 In Python, which keyword is used to check additional conditions if the previous conditions fail?
elif
else if
then
switch
Q32
Q32 What will happen if the condition in an if statement in Python evaluates to False?
The program will stop
It will execute the else block
It will raise an error
It will skip to the next if
Q33
Q33 Which of the following is a valid conditional statement in Python?
if a = 5:
if a == 5:
if a <> 5:
if (a = 5):
Q34
Q34 What is the outcome if the elif and else blocks are missing in an if statement?
Syntax error
The program will stop
Nothing, it's optional
Runtime error
Q35
Q35 What is the output of the following code?
x = 10;
if x > 5:
print("Greater")
Error
Greater
Nothing
Less
Q36
Q36 What will the following code print?
x = 10;
if x < 10:
print("Less");
else:
print("Not Less")
Less
Not Less
Error
Nothing
Q37
Q37 Pseudocode:
If age > 18,
print "Adult",
else
print "Minor"
Prints "Adult"
Prints "Minor"
Prints "Age"
No output
Q38
Q38 Pseudocode:
Check if a number is divisible by 2,
if yes
print "Even",
otherwise
"Odd"
Prints "Even"
Prints "Odd"
Error
No output
Q39
Q39 Pseudocode: What is printed when the temperature is 25 degrees?
If temperature > 30,
print "Hot",
elif temperature > 20,
print "Warm",
else
"Cold"
Prints "Hot"
Prints "Warm"
Prints "Cold"
No output
Q40
Q40 Identify the error:
if x > 10
print("Greater")
Missing parentheses around condition
No error
Missing colon after condition
Incorrect comparison operator
Q41
Q41 Find the mistake:
if x > 5:
print("Yes")
elif x < 10:
print("No")
Syntax error in the if block
Missing colon after elif
Missing else between blocks
No error
Q42
Q42 What is the use of a for loop in Python?
To repeat a block a fixed number of times
To check a condition
To declare variables
To handle exceptions
Q43
Q43 Which statement immediately terminates a loop in Python?
break
continue
exit
stop
Q44
Q44 What does the continue statement do inside a loop?
Pauses the loop
Stops the loop
Skips the current iteration
Exits the program
Q45
Q45 In a while loop, what happens if the condition never becomes False?
The loop runs forever
The loop stops after 10 iterations
Syntax error
The loop doesn't start
Q46
Q46 What is the difference between a for loop and a while loop in Python?
for is used for fixed iterations
while is faster than for
for can't use conditional statements
There is no difference
Q47
Q47 What is the output of the following code?
for i in range(3):
print(i)
"0 1 2"
"1 2 3"
"0 1 2 3"
"Error"
Q48
Q48 What will the following code print?
i = 5;
while i > 0:
print(i);
i -= 1
5 4 3 2 1
5 4 3 2
1 2 3 4 5
Infinite loop
Q49
Q49 Pseudocode:
Set x = 10;
While x > 0,
decrement x by 1,
print x
Prints numbers from 10 to 1
Prints numbers from 1 to 10
Prints numbers from 9 to 0
Infinite loop
Q50
Q50 Pseudocode:
For each element in list [1, 2, 3],
print element
Prints 1 2 3
Prints 3 2 1
Prints [1, 2, 3]
No output
Q51
Q51 Pseudocode:
For numbers 1 to 5,
print number
if it's odd
Prints 1 3 5
Prints 2 4
Prints all numbers 1 to 5
No output
Q52
Q52 Pseudocode:
For i = 0 to 4,
print "Python"
if i is even
Prints "Python" twice
Prints "Python" three times
Prints "Python" four times
No output
Q53
Q53 Identify the error:
for i in range(5)
print(i)
Missing colon after range(5)
Missing parentheses around print
Syntax error in range
No error
Q54
Q54 Find the mistake:
while True:
print("Looping");
break
Infinite loop
No error
The loop will never break
Incorrect use of print statement
Q55
Q55 What is the main difference between a list and a tuple in Python?
Syntax
Data type
Mutability
Method of declaration
Q56
Q56 How do you access the last element of a list named myList?
myList[0]
myList[-1]
myList[len(myList)]
myList[-2]
Q57
Q57 In Python, how can you combine two lists, list1 and list2?
list1 + list2
list1.append(list2)
list1.combine(list2)
list1.extend(list2)
Q58
Q58 What does myList[::-1] do?
Reverses myList
Copies myList
Removes the last element from myList
Sorts myList in descending order
Q59
Q59 How is memory allocation for lists and tuples different in Python?
Lists use more memory than tuples
Tuples use more memory than lists
Both use the same amount of memory
Memory usage is random and unpredictable
Q60
Q60 What will be the output of the following code?
myTuple = (1, 2, 3);
print(myTuple[1])
1
2
3
Error