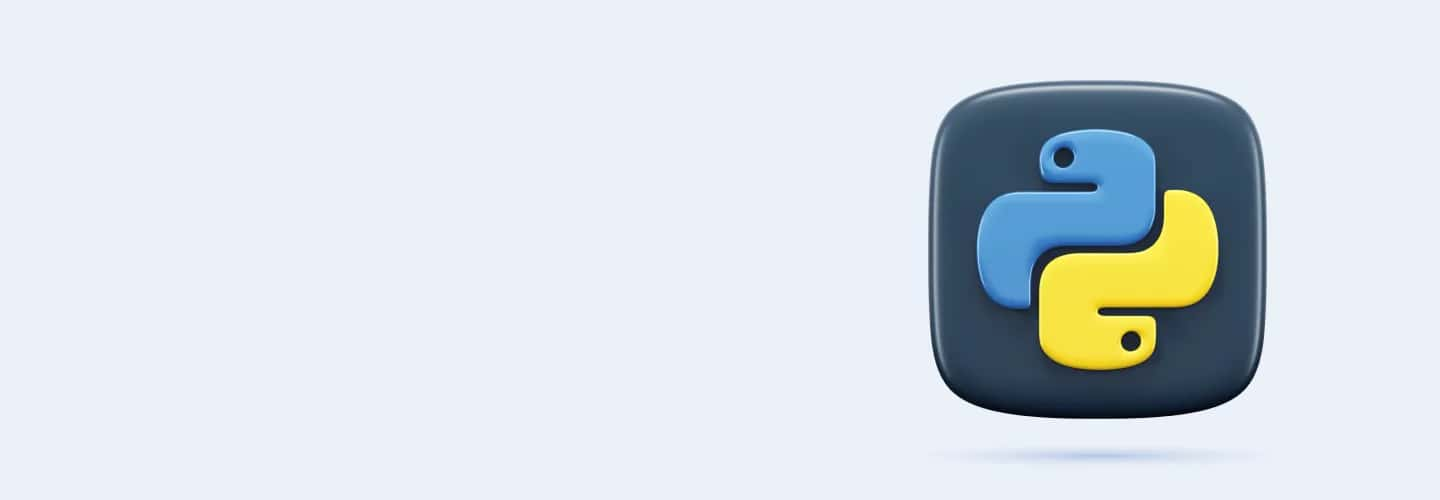
Q1
Q1 Python is known as:
A compiled language
An interpreted language
A machine language
An assembly language
Q2
Q2 Which version of Python removed the print statement?
Python 1.x
Python 2.x
Python 3.x
Python 4.x
Q3
Q3 Which of the following is a valid Python comment?
Q4
Q4 Python is a:
Low-level language
High-level language
Middle-level language
Machine-level language
Q5
Q5 Which of these data types does Python not natively support?
Lists
Tuples
Arrays
Dictionaries
Q6
Q6 Which of the following is a mutable data type in Python?
String
Tuple
List
Integer
Q7
Q7 What data type would you use to store a whole number in Python?
int
float
str
bool
Q8
Q8 Which of the following is not a Python built-in data type?
dict
array
set
frozenset
Q9
Q9 What will be the data type of the variable x after this assignment:
x = 3.5?
int
float
str
complex
Q10
Q10 Which of the following is an immutable data type?
Lists
Dictionaries
Tuples
Sets
Q11
Q11 What is the output of the following code snippet:
print(type("Hello, World!"))
Q12
Q12 What does the following code output:
x = [1, 2, 3];
print(type(x) == list)
TRUE
FALSE
Error
None
Q13
Q13 What will be the output of the following pseudocode?
Initialize x as 10;
If x is of type int,
print "Integer"
else
print "Not Integer"
Integer
Not Integer
Error
None
Q14
Q14 Pseudocode:
Variable
x = "Python";
Check if x is a string,
if yes
print "String",
otherwise
"Not a String"
String
Not a String
Error
None
Q15
Q15 Evaluate this pseudocode:
Set x = [1, 2, 3];
If x is a list, print length of x, else print "Not a list"
3
Not a list
Error
0
Q16
Q16 Identify the error in this code:
x = [1, 2, 3];
print(x)
Syntax error in variable x
Missing parenthesis in print
Missing bracket in x
No error
Q17
Q17 Find the mistake:
x = (1, 2, 3);
x[1] = 4;
print(x)
Tuples are immutable
x[1] should be x[2]
Syntax error
No error
Q18
Q18 Which function is used to read input from the console in Python?
input()
read()
scan()
getInput()
Q19
Q19 What is the default type of data returned by the input() function in Python 3.x?
int
string
boolean
list
Q20
Q20 Which function in Python is used to display data as output?
display()
print()
show()
output()
Q21
Q21 What is the purpose of the end parameter in the print() function?
To add a space at the end
To end the script
To specify the string appended after the last value
To break the line
Q22
Q22 What does the sep parameter do in the print() function?
Separates lines
Specifies separator between values
Separates syntax errors
None of these
Q23
Q23 What will be the output of
print("Python", "Programming", sep="-")
Python-Programming
Python Programming
PythonProgramming
Python,Programming
Q24
Q24 What is the output of
print("Hello", end='@');
print("World")
HelloWorld
Hello@World
Hello World
Hello@ World
Q25
Q25 Pseudocode:
Ask user for number, multiply by 2, print result
Reads number, prints double
Reads string, prints same
Error in input
No output
Q26
Q26 Pseudocode:
Print each element in list [1, 2, 3] with a space in between
123
37623
[1, 2, 3]
37623
Q27
Q27 Pseudocode:
Initialize a variable with "Python", print first and last character
Prints "Pn"
Prints "Py"
Prints "Python"
Error
Q28
Q28 Identify the error:
print("Hello World!"))
Missing quotation marks
Extra parenthesis
Missing parenthesis
No error
Q29
Q29 Find the mistake:
user_input = input("Enter a number: ");
print("You entered: ", int(user_input))
Syntax error
No error
int() should be str()
input() function used incorrectly
Q30
Q30 What is the purpose of an if statement in Python?
To loop through a sequence
To execute a block conditionally
To define a function
To handle exceptions