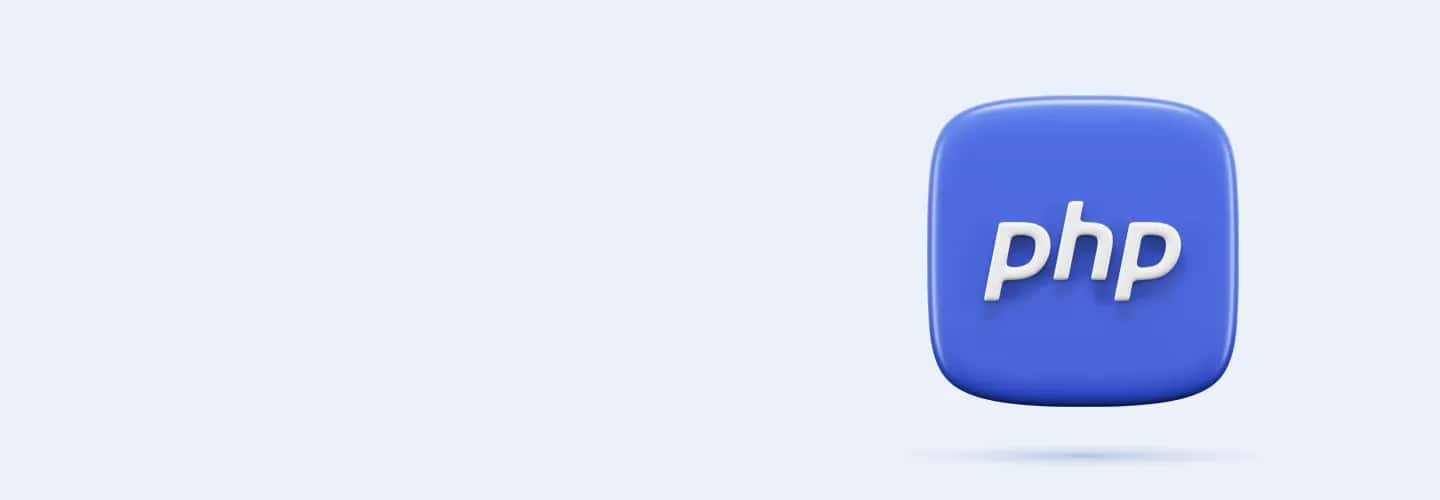
Q121
Q121 In PHP development, what is the purpose of using a coding standard like PSR (PHP Standards Recommendations)?
To enforce a specific framework's best practices
To ensure compatibility with PHP versions
To standardize coding style and practices for better readability and maintainability
To optimize performance
Q122
Q122 What is the best practice for handling errors in PHP to maintain code readability and manageability?
Using die() or exit() statements
Suppressing errors with the @ operator
Implementing structured exception handling with try-catch blocks
Logging errors to a file and displaying a generic error message to the user
Q123
Q123 How should sensitive configuration information (like database credentials) be managed in PHP applications?
Hardcoded in the PHP files
Stored in a separate configuration file and ignored from version control systems
Stored in the database
Encoded and embedded within the code
Q124
Q124 Identify the best practice when naming variables and functions in PHP.
Using short, non-descriptive names for brevity
Using all-uppercase letters for clarity
Using meaningful, descriptive names following a consistent naming convention
Varying naming conventions throughout the application
Q125
Q125 What is a common pitfall to avoid when using global variables in PHP?
Using them to store user input directly
Declaring them in every file
Using them extensively throughout the application
Initializing them in every function
Q126
Q126 What is AJAX primarily used for in web applications?
To create faster websites
To reload the entire webpage
To communicate with the server asynchronously
To increase server load
Q127
Q127 How does PHP typically respond to an AJAX request?
By reloading the page with new content
By sending a JavaScript function
By returning data, often in JSON or XML format
By redirecting the user to a different page
Q128
Q128 What is necessary on the client side to initiate an AJAX request to a PHP backend?
A PHP script on the client side
A form submission event
An XMLHttpRequest or a similar JavaScript API
A direct link to the PHP file
Q129
Q129 In the context of AJAX, what is the role of the XMLHttpRequest object in JavaScript?
To create new XML files
To send requests to and receive responses from a web server
To parse XML data only
To reload the webpage
Q130
Q130 What PHP method is generally used to access data sent via an AJAX POST request?
$_GET[]
$_POST[]
$_REQUEST[]
$_AJAX[]
Q131
Q131 How can you parse JSON data sent in an AJAX request to a PHP script?
Using json_decode() in PHP
Using parseJSON() in PHP
Using JSON.parse() in PHP
Using eval() in PHP
Q132
Q132 Identify the issue in this PHP snippet handling an AJAX request:
$data = json_decode($_POST['data']);
The $_POST array doesn't handle JSON data correctly
The json_decode() function is used incorrectly
There is no issue
The data should be accessed from $_GET instead
Q133
Q133 Spot the mistake in this AJAX request using jQuery to a PHP script:
$.ajax({
type: 'POST',
url: 'script.php',
data: { value: 'test' }
});
The type should be GET
The data object is formatted incorrectly
There is no mistake
The url should point to an HTML file
Q134
Q134 What is the primary purpose of a templating engine in PHP?
To increase PHP execution speed
To manage databases
To separate HTML from PHP logic
To encrypt data
Q135
Q135 Which popular templating engine is often used with Laravel?
Smarty
Blade
Twig
Mustache
Q136
Q136 How do templating engines generally output dynamic content?
By compiling templates into PHP code
By using AJAX calls
By embedding JavaScript
By direct PHP scripting in HTML files
Q137
Q137 What is the advantage of using template inheritance in PHP templating engines?
To allow multiple PHP versions
To reuse code in different parts of an application
To improve PHP performance
To enable better database integration
Q138
Q138 What is the correct way to display a variable in a Twig template?
Q139
Q139 In a Blade template, how do you include a sub-template (like a header or footer) into a main template?
Q140
Q140 Identify the error in this PHP use of a templating engine:
{{ echo $variable; }}
Incorrect syntax for variable output
Misuse of the echo command
No error
The variable should be inside PHP tags
Q141
Q141 Spot the mistake in this Blade template code:
@foreach($items as $item)
{{ $item }}
@endforUsing @endfor instead of @endforeach
Incorrect variable interpolation
No mistake
The syntax of @foreach is incorrect
Q142
Q142 How can PHP be integrated into an HTML file?
Using a separate PHP file for the backend
Embedding PHP code within HTML using PHP tags
Linking PHP files using the tag
Including PHP code in CSS files
Q143
Q143 What is the purpose of echoing HTML code in a PHP script?
To create a new HTML file
To send HTML content to the browser
To store HTML content in a variable
To style the PHP script
Q144
Q144 Can CSS be used to style HTML elements generated by PHP?
Yes, just like any other HTML elements
No, PHP-generated HTML elements cannot be styled with CSS
Only if the CSS is embedded in PHP
Only with inline CSS
Q145
Q145 What is the best practice for separating PHP logic from HTML/CSS in a web application?
Mixing PHP and HTML/CSS in the same file
Using PHP solely for backend processing and HTML/CSS for the frontend
Using inline PHP in CSS files
Embedding CSS in PHP files
Q146
Q146 What is the correct way to set the value of an HTML input field using PHP?
Q147
Q147 How can you dynamically set the class of an HTML element based on a PHP condition?
Q148
Q148 In a PHP script, how can you generate a list of HTML checkboxes from an array of values?
Q149
Q149 Identify the error in this PHP code used within HTML:
name ?>
The echo statement is unnecessary inside PHP tags
The variable $user->name is incorrectly formatted
There is no error
The semicolon is missing after echo $user->name
Q150
Q150 Spot the mistake in this PHP and HTML integration:
<button type='submit' >Submit
The if statement is incorrect
The echo statement should not be used
The syntax for the disabled attribute is incorrect
There is no mistake