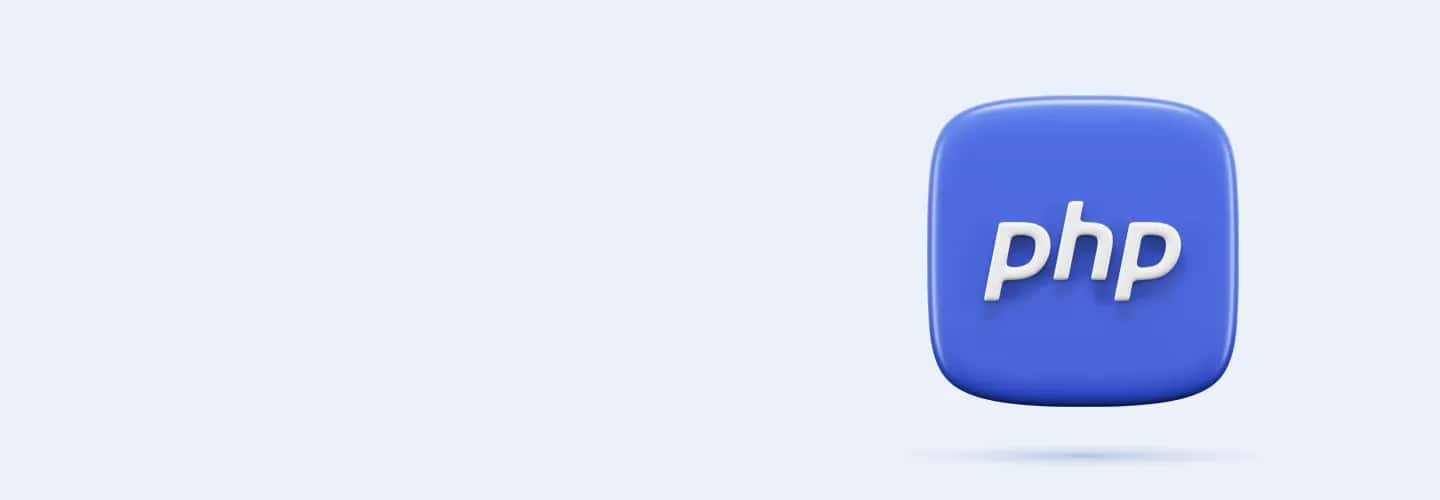
Q91
Q91 What is Cross-Site Request Forgery (CSRF) and how can it be prevented in PHP?
A type of attack where a malicious website performs actions on behalf of a user on another website
Using SSL certificates
Validating user input
Using tokens in forms
Q92
Q92 How can htmlspecialchars() function in PHP help in preventing security risks?
By encrypting data
By suppressing error messages
By converting special characters to HTML entities, thus preventing XSS attacks
By validating user input
Q93
Q93 In PHP, how can you securely handle file uploads to prevent malicious files from being uploaded?
By checking the file extension only
By limiting the file size
By validating the MIME type and checking file extensions, and storing files outside the web directory
By renaming files upon upload
Q94
Q94 Identify the security flaw in this PHP code snippet:
if (isset($_GET['user_id'])) {
$user_id = $_GET['user_id']; // Perform database query }
The user input is not sanitized before being used
No error in the code
The user ID should be stored in a session
The user ID should be encrypted
Q95
Q95 Spot the vulnerability in this PHP session handling:
session_start();
if (!isset($_SESSION['user'])) {
header('Location: login.php');
}
The session is not regenerated upon login
The session ID is not stored securely
There is no vulnerability in this code
The header location is not absolute
Q96
Q96 What is the primary purpose of cookies in web development?
To store server data
To store client-side, persistent user data
To improve network speed
To encrypt data
Q97
Q97 How are PHP sessions different from cookies?
Sessions are stored on the client-side, while cookies are stored on the server-side
Sessions and cookies are the same
Sessions are stored on the server-side, while cookies are stored on the client-side
Sessions encrypt data automatically
Q98
Q98 In PHP, what function is used to start a session?
session()
session_start()
start_session()
begin_session()
Q99
Q99 What is a session hijacking attack?
An attack where the attacker steals the session cookie
An attack where the server hijacks a client's session
A brute force attack
A SQL injection attack
Q100
Q100 How can you increase the security of PHP sessions?
By using SSL and storing sessions in a database
By increasing the session timeout
By disabling cookies
By using longer session IDs
Q101
Q101 What will be the output of the following PHP code?
setcookie("user", "John Doe", time() + 3600); echo $_COOKIE["user"];
John Doe
An error
Nothing
The current time + 3600
Q102
Q102 In PHP, how can you delete a cookie?
By setting its value to null
By using the delete_cookie() function
By setting its expiration date in the past
By unsetting it in $_COOKIE
Q103
Q103 Consider this PHP code:
session_start();
$_SESSION['user'] = 'Alice'; session_destroy();
echo $_SESSION['user'];
What is output?
Alice
An error
Nothing
The session ID
Q104
Q104 Identify the error in this PHP cookie code:
setcookie("user", "Alice", time() - 3600);
The cookie is being set with a past expiration time
The cookie value is incorrect
There is no error in the code
The cookie name is incorrect
Q105
Q105 Spot the mistake in this PHP session handling code:
session_start(); $_SESSION = array(); session_destroy();
Not using session_unset() before session_destroy()
Incorrect use of session_start()
No mistake
The session array should not be emptied
Q106
Q106 What is the main advantage of using a PHP framework like Laravel or Symfony?
Standardized coding practices
Faster execution of scripts
Automatic HTML parsing
Increased PHP script size
Q107
Q107 How does the MVC (Model-View-Controller) architecture in frameworks like Laravel and Symfony benefit web application development?
It enhances the UI/UX design
It speeds up the database read/write operations
It separates business logic, presentation, and data access
It reduces server load
Q108
Q108 In Laravel, what is a "Route" used for?
To optimize database queries
To create links to different pages
To define URLs and their corresponding actions in the application
To manage user sessions
Q109
Q109 Given a Laravel blade template, how can you display a variable passed from a controller?
Q110
Q110 In Symfony, what is the purpose of a "Service Container"?
To manage user authentication
To store and retrieve session data
To automatically inject dependencies into classes
To handle database migrations
Q111
Q111 Identify the issue in this Symfony controller method:
public function index() {
return $this->render('index.html.twig');
}
The method doesn't specify a route
The template file extension should be .blade.php
The method must return a Response object
No issue in the code
Q112
Q112 Spot the mistake in this Laravel Eloquent query:
$users = User::where('age', '>', 30)->get();
The get() method is used incorrectly
The where clause is incorrect
There is no mistake
The model User is likely not defined
Q113
Q113 What is a RESTful API in the context of web services?
An API that uses XML for data exchange
An API that allows only GET requests
An API designed around the principles of REST (Representational State Transfer)
An API that requires SOAP protocol
Q114
Q114 What is the primary role of JSON in web services developed with PHP?
To structure the layout of a web page
To act as a query language for databases
To format and transfer data between client and server
To encrypt data during transmission
Q115
Q115 How can you send a JSON response from a PHP script?
json_encode()
json_response()
header("Content-Type: application/json"); echo json_encode($data);
send_json($data);
Q116
Q116 In PHP, how can you consume a RESTful API using cURL?
Using file_get_contents() with the API URL
Using the cURL functions to make a request and receive a response
Using JSON encoding and decoding
Using simple XML loading functions
Q117
Q117 Identify the common error in this API request code in PHP:
$response = file_get_contents('https://api.example.com/data'
);
Not checking for a false response
Incorrect URL format
Not setting a user agent
The function file_get_contents cannot be used for API requests
Q118
Q118 Spot the mistake in this PHP code for sending a POST request using cURL:
curl_setopt($ch, CURLOPT_POST, true); curl_exec($ch);
Not setting the CURLOPT_POSTFIELDS option
The CURLOPT_POST option is incorrectly used
curl_exec() is used incorrectly
No mistake in the code
Q119
Q119 What is the significance of using the PHP Data Objects (PDO) extension for database access?
It provides faster query execution
It is specific to MySQL databases
It offers a secure, consistent way to interact with different databases using the same interface
It automatically creates database schemas
Q120
Q120 Why is it recommended to avoid using the PHP closing tag ?> at the end of a file?
To prevent syntax errors
To improve performance
To prevent accidental whitespace or new lines after the tag, which can cause output buffering issues
To conform to PSR standards