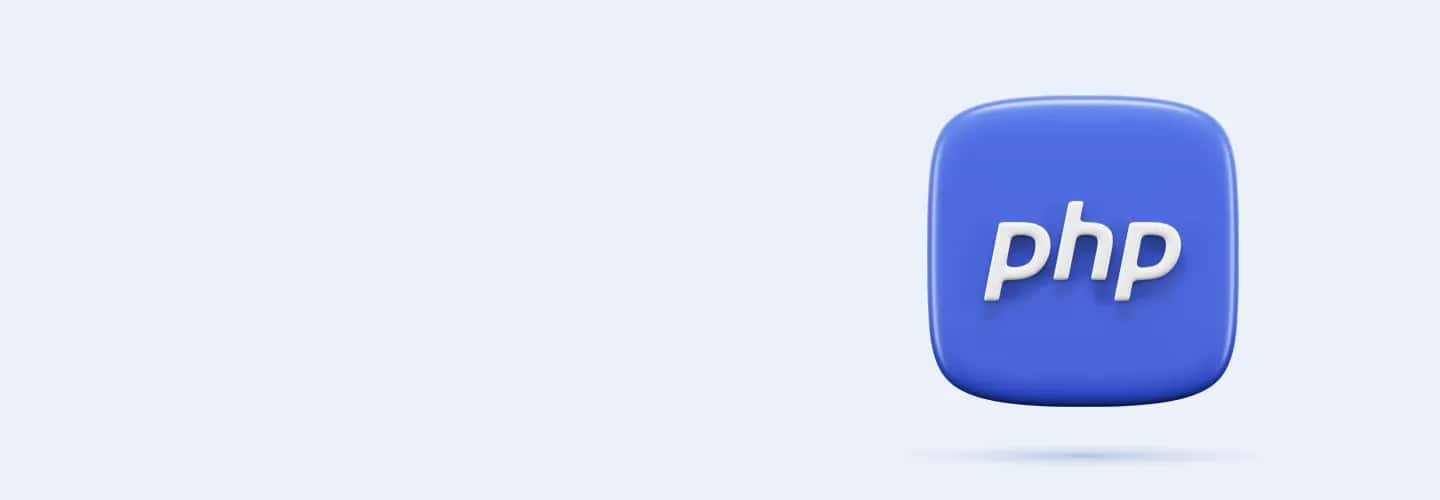
Q61
Q61 Identify the issue in this PHP code:
$str = 'Hello'; echo $str[10];
Trying to access an undefined index
Syntax error
No error, it will print 'Hello'
It will print a space
Q62
Q62 Find the error in the following PHP code:
$arr = array("one", "two", "three");
foreach ($ar as $val) {
echo $val;
}
Typo in the variable name inside foreach
Syntax error in array declaration
No error in the code
Error in echo statement
Q63
Q63 What keyword is used to declare a class in PHP?
class
object
new
struct
Q64
Q64 What is the purpose of the __construct() method in a PHP class?
To configure a server
To create an object from the class
To initialize an object's properties
To delete an object
Q65
Q65 In PHP, which keyword is used to inherit a class from another class?
extends
inherits
uses
implements
Q66
Q66 What does the final keyword signify when applied to a method in PHP?
The method cannot be overridden
The method is the last in the class
The method is static
The method is the most important in the class
Q67
Q67 How is a static property accessed in a PHP class?
With the new keyword
With the -> operator
Using the class name and ::
Using the $this keyword
Q68
Q68 What will be the output of the following PHP code?
class Test {
public $prop = 'Hello';
}
$obj = new Test();
echo $obj->prop;
Hello
Test
prop
Error
Q69
Q69 Consider the PHP class:
class Circle {
private $radius;
public function __construct($r) {
$this->radius = $r;
}
public function getArea() {
return pi() *
$this->radius * $this->radius; }
}
$circle = new Circle(3);
echo $circle->getArea();
The area of a circle with radius 3
Syntax error
No output
Error due to private property access
Q70
Q70 What is the result of calling the following PHP code?
class Animal {
protected $sound = "No sound";
public function makeSound() {
return $this->sound;
}
}
class Dog extends Animal {
protected $sound = "Bark";
}
$dog = new Dog();
echo $dog->makeSound();
Bark
No sound
Error
Dog
Q71
Q71 Identify the error in the following PHP class definition:
class Car {
function __construct() {
$this->model = '';
}
public function getModel() {
return model;
}
}
Undefined variable model
Syntax error in constructor
Missing property declaration
No error in the code
Q72
Q72 Find the mistake in this PHP code involving object-oriented principles:
class Book { public $title; public function __construct(title) { $this->title = title; } }
Missing $ before parameter in constructor
Syntax error in property declaration
No error in the code
Error in method definition
Q73
Q73 In PHP, which operator is used at the beginning of an expression to suppress error messages that it may generate?
@
#
$
!
Q74
Q74 What is the purpose of the try and catch block in PHP?
To detect and handle syntax errors
To manage program flow
To handle exceptions and errors
To debug code
Q75
Q75 Which PHP function is used to set a custom error handler?
set_error_handler()
error_report()
handle_error()
trigger_error()
Q76
Q76 What is the difference between exceptions and errors in PHP?
Exceptions can be caught and handled, errors cannot
Errors are for system-level issues, exceptions are for script issues
Exceptions are fatal, errors are not
There is no difference
Q77
Q77 What will happen if an exception is not caught in PHP?
The script will continue running
A fatal error will occur
The exception will be ignored
The script will pause
Q78
Q78 Given the code:
$num = -1;
try {
if($num < 0) {
throw new Exception("Negative number");
}
} catch (Exception $e) {
echo $e->getMessage();
}
What will be output?
Negative number
Nothing
Exception in script
Error
Q79
Q79 Identify the error in this PHP error handling code:
try {
//code } catch() {
echo "Error occurred";
}
Empty catch block
Missing exception type in catch block
Syntax error in try block
No error in the code
Q80
Q80 Spot the mistake in this PHP exception handling:
try {
$value = 10/0;
}
catch (Exception $e) {
echo "Caught exception: ", $e->getMessage();
}
Dividing by zero is not caught
Incorrect message concatenation
No mistake, it will catch and display the exception
The variable $e is not defined
Q81
Q81 In PHP, which function is used to open a file?
fopen()
open_file()
file_open()
open()
Q82
Q82 Which file mode in PHP can be used to open a file for both reading and writing, and places the file pointer at the beginning?
r+
w+
a+
x+
Q83
Q83 What does the PHP file_get_contents() function do?
Reads the entire file into a string
Deletes the specified file
Creates a new file
Writes content to a file
Q84
Q84 In PHP, how do you check if a file exists and is readable?
Using is_readable() and file_exists()
Using file_exists()
Using can_read()
Using fopen() and checking if it's false
Q85
Q85 What is the result of the following PHP code?
$file = fopen("test.txt", "w"); fwrite($file, "Hello, World!"); fclose($file);
A new file named "test.txt" is created with "Hello, World!" inside
An error occurs
Nothing happens
The file "test.txt" is deleted
Q86
Q86 In PHP, what does the file_put_contents() function do when the FILE_APPEND flag is used?
Replaces the content of the file
Deletes the file and creates a new one
Appends content to the end of the file
Creates a new file
Q87
Q87 Identify the error in this PHP file handling code:
$file = fopen("nonexistent.txt", "r"); fwrite($file, "Data"); fclose($file);
The file does not exist
Incorrect mode for fwrite
Syntax error
No error in the code
Q88
Q88 Spot the mistake in this PHP code for reading a file:
$file = fopen("file.txt", "r");
$data = fread($file, filesize("file.txt"));
fclose($file); echo $data;
There is no mistake
Missing error handling for file opening
Incorrect use of filesize()
The file is not closed properly
Q89
Q89 What is the primary purpose of using prepared statements in PHP?
To optimize query execution speed
To improve code readability
To protect against SQL injection attacks
To handle large datasets
Q90
Q90 In PHP, what security measure should be implemented to protect sensitive data, like passwords, stored in a database?
Storing them as plain text with unique identifiers
Encrypting them using reversible encryption
Hashing them using a cryptographic hash function
Compressing them before storage