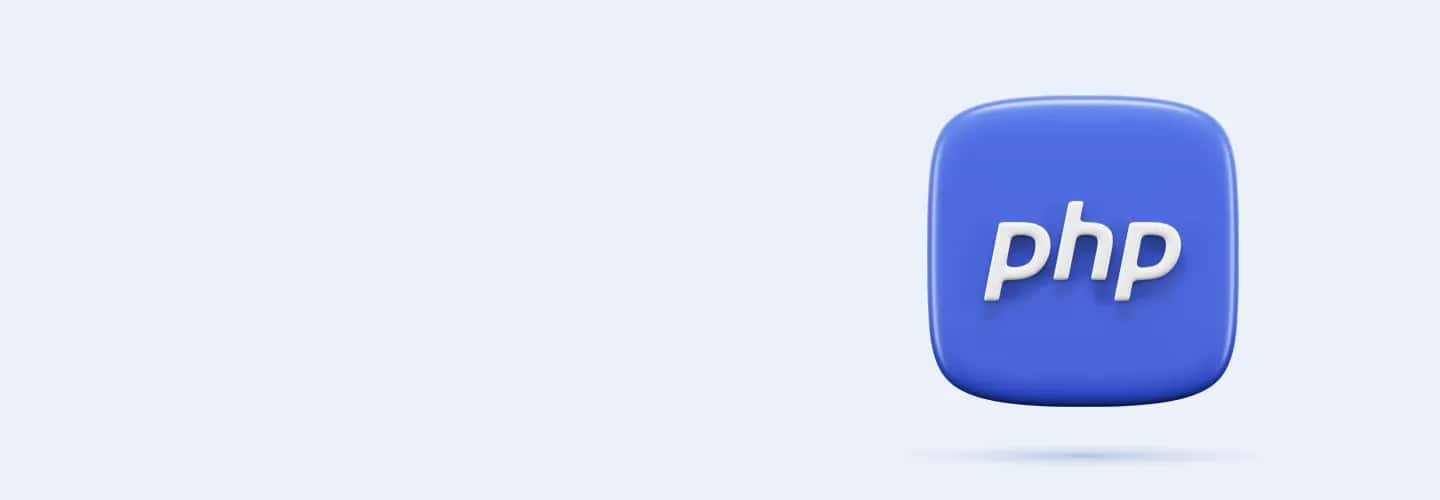
Q31
Q31 Consider the PHP code:
$stmt = $mysqli->prepare("SELECT * FROM users WHERE id = ?");
$stmt->bind_param("i", $id);
What does the "i" in bind_param() signify?
Integer data type
Incremental value
Input parameter
Invalid parameter
Q32
Q32 In a PHP script using MySQLi, what does the close() method do when applied to a mysqli object?
Closes the database connection
Saves changes to the database
Closes the PHP script
Resets all variables in the script
Q33
Q33 Identify the error in the following PHP code:
$mysqli = new mysqli("localhost", "user", "password", "database"); $result = $mysqli->query("SELECT * FROM users WHERE id = '$id'");
No error in the code
Missing database connection error handling
Improper string concatenation in the query
SQL injection vulnerability
Q34
Q34 Spot the error in this PHP database connection code:
$mysqli = new mysqli("localhost", "user", "password"); $mysqli->select_db("database");
Missing database in the constructor
Improper method to select a database
No error in the code
Syntax error in mysqli instantiation
Q35
Q35 Which PHP statement is used to execute the same code a specified number of times?
foreach
while
for
do-while
Q36
Q36 In PHP, the switch statement is used as an alternative to which other control structure?
if
while
for
foreach
Q37
Q37 What is the purpose of the break statement in PHP loops?
To pause the loop execution
To exit the loop
To skip the current iteration
To continue loop execution
Q38
Q38 In PHP, what will happen if you forget to increment the counter in a while loop?
The loop will run indefinitely
The loop will exit immediately
The loop will throw an error
The loop will skip iterations
Q39
Q39 Which looping structure is best for iterating over an associative array in PHP?
for
foreach
while
do-while
Q40
Q40 What will be the output of the following PHP code?
$i = 0;
while($i < 3) {
echo $i;
$i++;
}
?>
012
123
210
An error
Q41
Q41 Consider the following PHP code:
for ($i = 1; $i <= 5; $i++) {
if ($i == 3) {
continue;
}
echo $i;
}
?>
What will be the output?
1245
12345
1234
Error
Q42
Q42 Identify the error in this PHP foreach loop:
foreach($array as $value) {
echo $value
}
?>
Missing semicolon after echo
Syntax error in foreach
Missing $ before array
No error in the code
Q43
Q43 Spot the mistake in this PHP while loop:
$i = 0;
while ($i <= 5) {
echo $i
}
$i++;
?>
Infinite loop
Missing increment inside loop
Missing semicolon after echo
Syntax error in while
Q44
Q44 Find the error in this PHP code involving a for loop:
echo $i;
}
?>
Syntax error in for loop
Missing semicolon after echo
No error in the code
Infinite loop
Q45
Q45 What is the keyword used to define a function in PHP?
function
def
create
func
Q46
Q46 What is a primary purpose of a function in PHP?
To repeat a block of code multiple times
To stop the execution of a script
To organize and reuse code
To declare variables
Q47
Q47 In PHP, what is a function parameter that is given a default value called?
Mandatory parameter
Optional parameter
Dynamic parameter
Static parameter
Q48
Q48 What is the scope of a variable declared inside a PHP function?
Global
Local
Static
Universal
Q49
Q49 Which of the following statements about PHP anonymous functions is true?
They must have a name
They cannot use external variables
They are a type of PHP class
They can be assigned to a variable or passed as an argument
Q50
Q50 What will be the output of this PHP function call?
function add($x, $y) {
return $x + $y;
}
echo add(2, 3);
5
23
Error
Nothing
Q51
Q51 Given the PHP function:
function isEven($num) {
return $num % 2 == 0;
}
What will isEven(5) return?
true
false
5
null
Q52
Q52 Consider the following PHP function:
function multiply(&$value) {
$value *= 2;
}
$num = 10;
multiply($num);
What will be the value of $num after the function call?
10
20
Error
Null
Q53
Q53 Identify the error in this PHP function definition:
function multiply($x, $y) {
return $x * $y return $x + $y;
}
Multiple return statements
Missing semicolon
Syntax error in return statements
No error in the code
Q54
Q54 Spot the mistake in this PHP function:
function getSum($arr) {
$sum = 0;
foreach($arr as $num) {
$sum += $num;
}
return $sum; } What will getSum([1, 2, 3]) return?
Nothing, there is a syntax error
6
Error due to incorrect parameter type
3
Q55
Q55 In PHP, which function is used to count the number of elements in an array?
count()
get_length()
size()
array_size()
Q56
Q56 What is the purpose of the explode() function in PHP?
To combine array elements into a string
To split a string into an array
To search for a string in an array
To sort an array
Q57
Q57 In PHP, which function is used to join array elements with a string?
join()
merge()
concat()
array_join()
Q58
Q58 Which of the following is a correct way to declare a multidimensional array in PHP?
$array = array(array(1, 2, 3), array(4, 5, 6));
$array = [array(1, 2, 3), array(4, 5, 6)];
$array = {(1, 2, 3), (4, 5, 6)};
$array = {array(1, 2, 3), array(4, 5, 6)};
Q59
Q59 What will be the output of the following PHP code?
$array = ['a', 'b', 'c']; echo $array[1];
a
b
c
Error
Q60
Q60 Consider this PHP code:
$str = "Hello World";
$array = str_split($str, 3);
print_r($array);
What does print_r($array); output?
Array ( [0] => Hel [1] => lo [2] => Wo [3] => rld )
Array ( [0] => Hello [1] => World )
Array ( [0] => H [1] => e [2] => l [3] => l [4] => o [5] => [6] => W [7] => o [8] => r [9] => l [10] => d )
Error