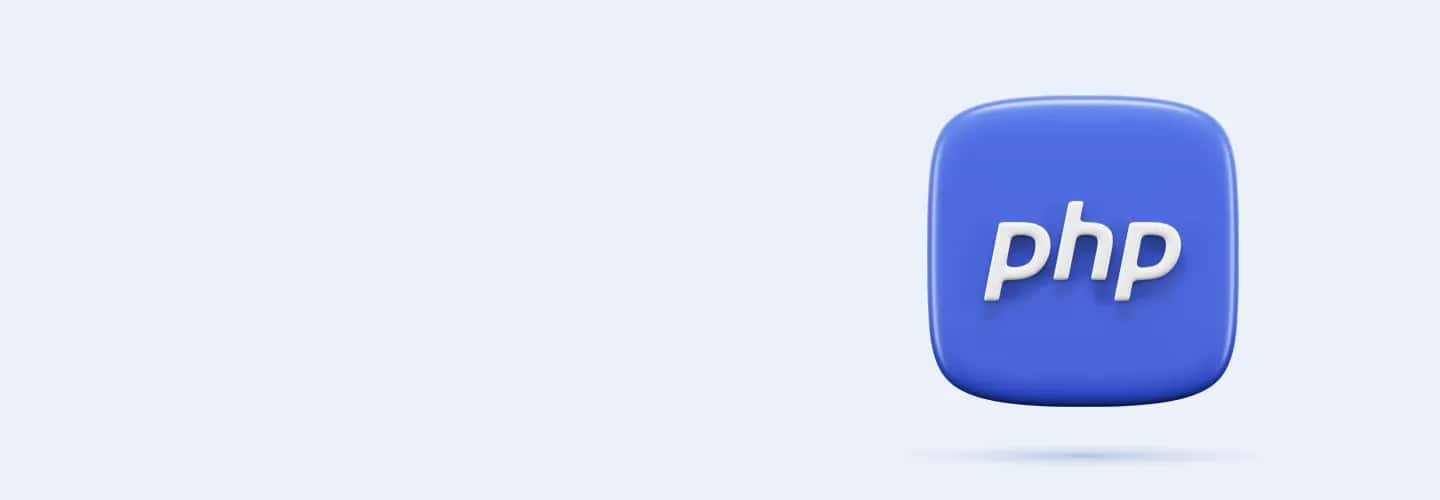
Q1
Q1 Which of the following is a correct PHP tag?
Q2
Q2 PHP can be embedded in which of the following types of documents?
HTML only
CSS only
JavaScript only
HTML, CSS, and JavaScript
Q3
Q3 In PHP, which character is used at the end of each statement?
:
;
,
.
Q4
Q4 How do you write comments in PHP?
Using // for single line and /* */ for multiple lines
Using #
Using
Using **
Q5
Q5 What is the correct way to declare a variable in PHP?
int $varName
$varName
var $varName
declare $varName
Q6
Q6 Which of the following is a correct way to define a constant in PHP?
define('CONST_NAME', 'Value');
const CONST_NAME = 'Value';
Both a and b
None of the above
Q7
Q7 What will be the output of the following PHP code?
6
123
Error
1
Q8
Q8 Which of the following data types is not supported in PHP?
String
Array
Object
Character
Q9
Q9 What will be the output of the following PHP code?
$x = "Hello";
$y = 'World';
echo $x . " " . $y;
?>
HelloWorld
Hello World
Hello+World
Error
Q10
Q10 Consider the PHP array:
$arr = array(1, 2, 3, 4);
What is the correct way to access the third element?
$arr[2]
$arr[3]
$arr[2]
$arr(3)
Q11
Q11 Identify the error in this PHP code snippet:
Missing semicolon
Syntax error in echo
Variable declaration error
No error in the code
Q12
Q12 Spot the error in the following PHP code:
for ($i = 0; $i <= 10; $i++) {
echo $i; } echo $i;
?>
Undefined variable outside loop
Missing semicolon
No error in the code
Syntax error in loop
Q13
Q13 What method should be used to send sensitive data in a form?
GET
POST
PUT
DELETE
Q14
Q14 Which superglobal array in PHP contains information about headers, paths, and script locations?
$_GET
$_POST
$_SERVER
$_FILES
Q15
Q15 In a form, to group multiple elements that belong together, which HTML tag is used?
Q16
Q16 How do you access form data sent via the GET method in PHP?
Using the $_REQUEST superglobal
Using the $_GET superglobal
Using the $_POST superglobal
Using the $_SERVER superglobal
Q17
Q17 What is the correct way to check if a form has been submitted in PHP?
Checking if $_POST is set
Checking if $_GET is set
Checking if $_SERVER['REQUEST_METHOD'] is POST
Checking if $_REQUEST is set
Q18
Q18 Which attribute in a form input element specifies the field's initial value?
value
type
name
placeholder
Q19
Q19 Given a form with method="post", which PHP array will contain the form's submitted data?
$_GET
$_POST
$_REQUEST
$_SERVER
Q20
Q20 What will be the output of the following PHP code if a user submits a form with an input named email?
if (isset($_POST['email'])) {
echo $_POST['email'];
}
?>
The value of the email input field
NULL
An empty string
An error message
Q21
Q21 In PHP, how do you securely access a form value sent via POST to prevent XSS attacks?
Using htmlspecialchars($_POST['value'])
Using $_POST['value'] directly
Using strip_tags($_POST['value'])
Using mysqli_real_escape_string($_POST['value'])
Q22
Q22 Identify the issue in this PHP code for handling a form input:
if (isset($_GET['submit'])) {
echo $_POST['name'];
}
?>
Incorrect use of $_GET and $_POST
No issue
Syntax error
The input name should be 'submit' instead of 'name'
Q23
Q23 Spot the error in the following PHP form handling code:
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
echo $_POST[name];
}
?>
Missing quotes around array key name
No error in the code
Syntax error in if statement
Missing semicolon in echo statement
Q24
Q24 Which PHP function is used to connect to a MySQL database?
mysql_connect()
mysqli_connect()
connect_db()
db_connect()
Q25
Q25 In PHP, which function is used to execute a SQL query against a MySQL database?
mysql_query()
mysqli_query()
execute_sql()
run_query()
Q26
Q26 When retrieving data from a MySQL database, which PHP function is used to fetch a row as an associative array?
mysqli_fetch_assoc()
mysqli_fetch_array()
mysqli_fetch_row()
mysqli_fetch_all()
Q27
Q27 What does the PHP mysqli_real_escape_string() function do?
Sanitizes input for use in a SQL query
Converts special characters to HTML entities
Encrypts a string
Splits a string into an array
Q28
Q28 Which of the following is an appropriate use of PHP Data Objects (PDO)?
Connecting to multiple database types
Creating HTML content
Parsing JSON data
Managing PHP sessions
Q29
Q29 What is the main advantage of using prepared statements in PHP for database queries?
Increased query execution speed
Reduced memory usage
Enhanced security against SQL injection attacks
Simplified syntax
Q30
Q30 What does the connect_errno property of a mysqli object indicate in PHP?
It indicates the error number of the last connection attempt
It returns the total number of failed connection attempts
It is a boolean value indicating whether the connection was successful
It stores the MySQL server version