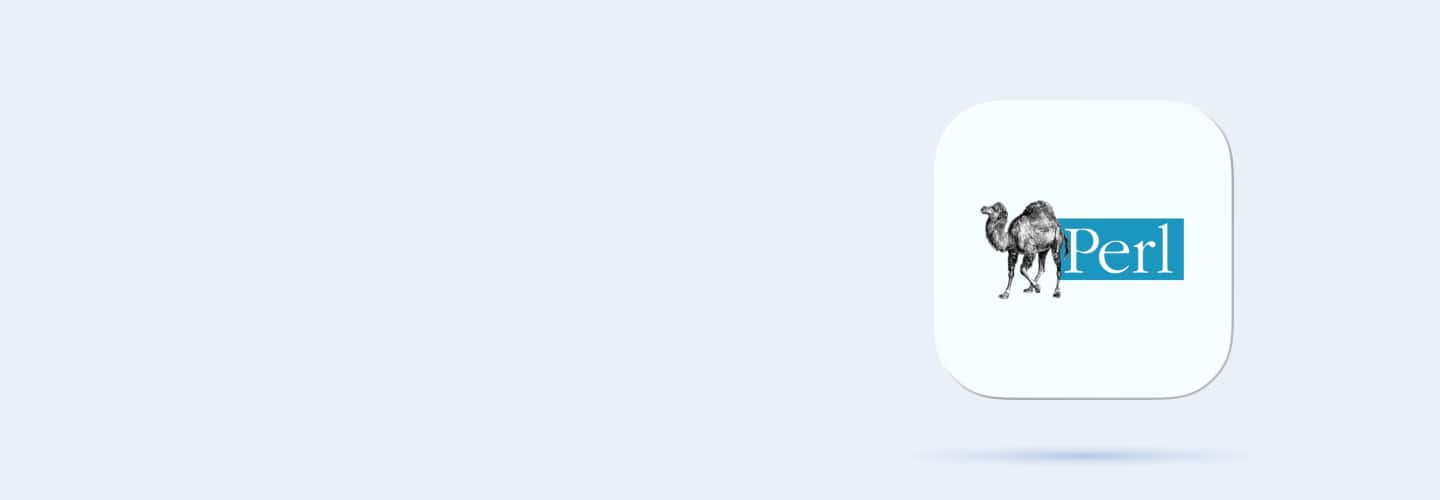
Q61
Q61 What will if ("hello" =~ /h.llo/) return?
True
False
Error
Undefined
Q62
Q62 What does $string =~ s/\d+/XXX/g; do?
Replaces all numbers with "XXX"
Removes all numbers
Finds numbers in a string
Counts the digits in a string
Q63
Q63 What will $string =~ tr/a-z/A-Z/; do in Perl?
Convert lowercase to uppercase
Remove all lowercase letters
Replace spaces with underscores
Find occurrences of vowels
Q64
Q64 What will be the output of $string = "abc123"; $string =~ /(\d+)/; print "$1";?
abc
123
Error
Empty string
Q65
Q65 Why does if ($text =~ /word/) sometimes fail unexpectedly?
Perl does not support regex
The variable is undefined
Regex match is case-sensitive by default
Regex must always use s///
Q66
Q66 Why does if ($str =~ /^test/) fail to match " mytest"?
The regex is incorrect
The caret ^ matches only the start of the string
Regex does not support spaces
The /i flag is missing
Q67
Q67 Why does if ($data =~ /\bword\b/) fail when searching for "word" inside "password"?
Perl does not support \b
\b requires spaces around words
\b matches word boundaries
"password" is an exception in Perl regex
Q68
Q68 Which operator is used to open a file in Perl?
<>
open
read
file
Q69
Q69 What does the die function do in Perl file handling?
Terminates execution with an error message
Continues execution after error
Closes the file
Deletes a file
Q70
Q70 What mode should be used to open a file for appending in Perl?
>file
>>file
<>>file
Q71
Q71 What does
Opens a file
Reads from a file
Deletes a file
Closes a file
Q72
Q72 What does binmode do when working with filehandles?
Opens a file in binary mode
Converts a file to text
Encodes file contents
Deletes a file
Q73
Q73 What will be the output of the following Perl code?
open(my $fh, "<", "file.txt") or die "Cannot open file";
Opens file.txt for reading
Opens file.txt for writing
Appends to file.txt
Throws an error
Q74
Q74 How do you correctly close a file in Perl?
end $file;
stop $file;
close $fh;
exit $file;
Q75
Q75 What will the following code do?
open(my $fh, ">", "output.txt"); print $fh "Hello, World!"; close $fh;
Creates output.txt and writes "Hello, World!"
Appends "Hello, World!" to output.txt
Throws an error
Reads from output.txt
Q76
Q76 What does this Perl statement do?
open(my $fh, "<", "file.txt") or warn "File not found";
Opens file.txt for reading
Opens file.txt for writing
Prints a warning if the file cannot be opened
Deletes file.txt
Q77
Q77 Why does the following Perl script fail?
open(my $fh, "<", "nonexistent.txt") or die "File error";
The file does not exist
Perl does not support file handling
The file is already open
Incorrect syntax
Q78
Q78 Why does a file handle sometimes not close properly in Perl?
The file is locked
The close function is not used
Perl does not allow closing files
The file has too much data
Q79
Q79 What is a common reason for a "Permission Denied" error in Perl file handling?
File is already open
Incorrect file path
Trying to write to a read-only file
File contains binary data
Q80
Q80 What symbol is used to define an array in Perl?
%
@
$
&
Q81
Q81 What is the correct way to access the first element of an array in Perl?
array[0]
@array[0]
$array[0]
array->0
Q82
Q82 How can you find the number of elements in an array?
count(@array)
scalar @array
size(@array)
length(@array)
Q83
Q83 How are hash keys and values stored in Perl?
As ordered lists
As key-value pairs
As arrays
As objects
Q84
Q84 What function is used to extract all keys from a hash?
keys()
values()
extract_keys()
get_keys()
Q85
Q85 What is the output of @arr = (1, 2, 3); print $arr[1];?
1
2
3
Error
Q86
Q86 What will the following Perl code do?
%hash = ("a" => 1, "b" => 2); print $hash{"b"};
Prints 1
Prints 2
Prints b
Throws an error
Q87
Q87 What will happen if you attempt to access a hash key that does not exist?
Returns undef
Throws an error
Returns 0
Returns an empty string
Q88
Q88 What does push(@array, 5); do?
Adds 5 to the end of @array
Removes 5 from @array
Replaces the first element with 5
Deletes @array
Q89
Q89 Why does print @array; sometimes produce unexpected output?
@array must be enclosed in double quotes
The array contains undefined values
Perl does not support printing arrays
Arrays must be printed with join()
Q90
Q90 What happens if you attempt to delete a hash key that does not exist?
Throws an error
Nothing happens
Deletes a random key
Resets the hash