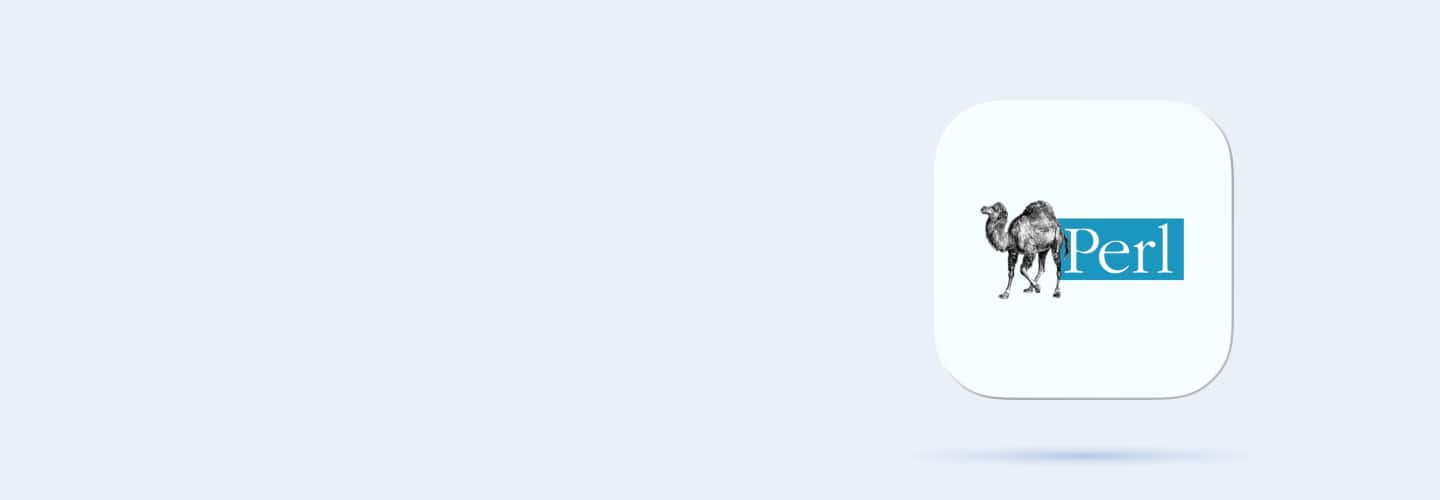
Q31
Q31 What will be the output of my $x = "abc" + 10; print $x;?
abc10
10
Error
0
Q32
Q32 Which of the following is a valid conditional statement in Perl?
if-else
when
try-catch
foreach
Q33
Q33 What keyword is used to exit a loop prematurely in Perl?
stop
break
exit
last
Q34
Q34 Which loop is best for iterating over an array in Perl?
while
for
foreach
do-while
Q35
Q35 What is the default condition for an unless statement in Perl?
True
False
Undefined
Nonzero
Q36
Q36 What is the purpose of the redo statement in Perl loops?
Repeats the loop from the beginning without re-evaluating the condition
Exits the loop immediately
Skips the next iteration
Restarts the script
Q37
Q37 Which of the following control structures is unique to Perl?
do-while
foreach
given-when
switch-case
Q38
Q38 What will be the output of for (my $i = 0; $i < 3; $i++) { print "$i "; }?
1 2 3
0 1 2
0 1 2 3
Error
Q39
Q39 What does the following Perl code do?
while ($count < 5) { print "$count "; $count++; }
Prints 1 to 5
Prints 0 to 4
Prints 0 to 5
Throws an error
Q40
Q40 What will happen if a loop has a missing condition in Perl?
It runs infinitely
It throws an error
It executes once
It skips to the next loop
Q41
Q41 Why does this code if ($x = 5) { print "True"; } always print "True"?
The variable is uninitialized
The comparison is incorrect
The assignment operator is used instead of comparison
The loop is infinite
Q42
Q42 What is the effect of using next inside a loop in Perl?
Exits the loop
Skips the current iteration and moves to the next one
Throws an error
Repeats the iteration
Q43
Q43 What is the common cause of an infinite loop in Perl?
Using last inside the loop
Forgetting to increment a loop variable
Using redo incorrectly
Using next outside a loop
Q44
Q44 What keyword is used to define a subroutine in Perl?
sub
function
def
method
Q45
Q45 How does a Perl subroutine return a value?
Using return statement
Automatically returns the last evaluated expression
Both A and B
Perl subroutines do not return values
Q46
Q46 How are arguments passed to a subroutine in Perl?
Using parentheses
Through the special @_ array
Using the global variable $$args
Directly as individual variables
Q47
Q47 What does shift do inside a Perl subroutine?
Shifts the first value off an array
Moves the last value to the beginning
Removes all elements of an array
Adds a new value to an array
Q48
Q48 What is the default return value of a Perl subroutine if no explicit return is given?
0
undef
Empty string
The last evaluated expression
Q49
Q49 Which of the following is NOT true about Perl subroutines?
They can modify global variables
They can accept parameters
They always return a value
They are always executed in a new scope
Q50
Q50 What will be printed by this Perl subroutine?
sub greet { my $name = shift; print "Hello, $name!\n"; } greet("Alice");
Hello, Alice!
Hello, World!
Alice! Hello,
Syntax error
Q51
Q51 How do you call a Perl subroutine named calculate and pass two arguments (5,10)?
calculate 5, 10;
calculate(5, 10);
sub calculate(5,10);
call calculate 5, 10;
Q52
Q52 What will the following Perl subroutine return?
sub add { my ($a, $b) = @_; return $a + $b; } print add(2,3);
2
3
5
Error
Q53
Q53 A Perl subroutine is modifying a global variable unexpectedly. What is the likely cause?
The subroutine is modifying @_ directly
The variable was not declared with my
A return statement is missing
Perl subroutines cannot modify variables
Q54
Q54 Why does this subroutine throw an error?
sub example { my $x = shift; print "Value: $x"; } example();
$x is uninitialized
Too many arguments passed
Syntax error
Subroutines require return values
Q55
Q55 Why does this code not print anything?
sub test { return; print "Hello"; } test();
print is inside a return statement
Perl suppresses print inside subroutines
The function call is incorrect
Perl does not allow return values in subroutines
Q56
Q56 What operator is used in Perl for regular expressions?
==
=~
!=
<>
Q57
Q57 What does the ^ symbol mean in a Perl regular expression?
Matches the end of a string
Matches the beginning of a string
Matches a digit
Matches any character
Q58
Q58 What does /abc/i do in Perl regular expressions?
Matches "abc" exactly
Matches "abc" case-insensitively
Matches "abc" only at the start of a string
Matches "abc" only in arrays
Q59
Q59 Which character is used in Perl regex to match any single character?
*
.
+
?
Q60
Q60 What is the purpose of (?: ... ) in Perl regex?
Creates a capturing group
Creates a non-capturing group
Matches an empty string
Matches a single character