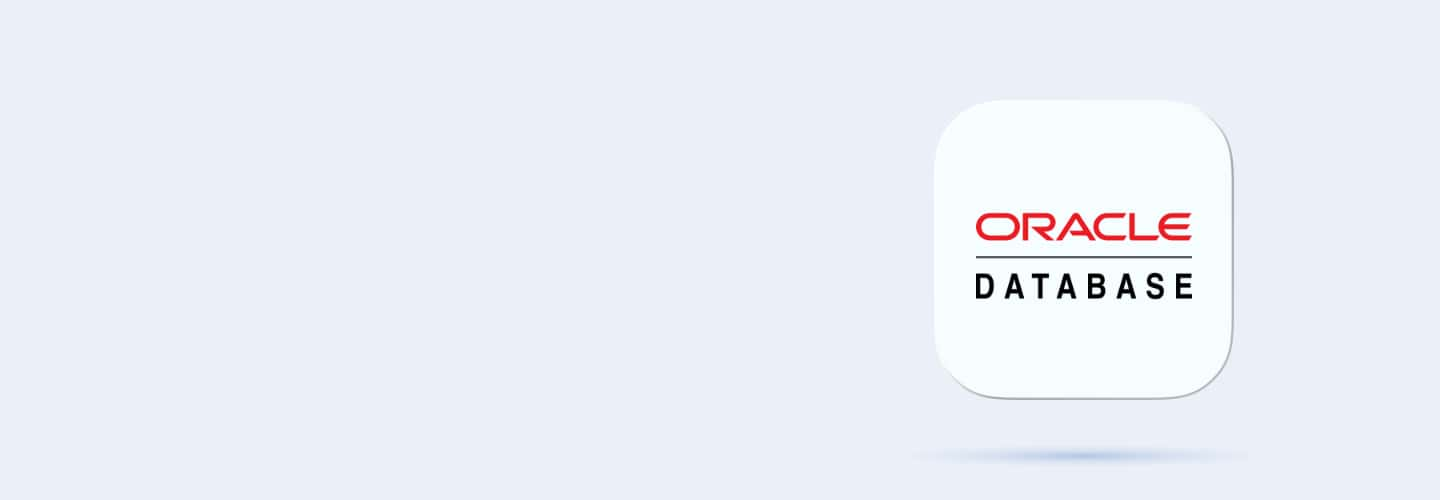
Q31
Q31 An SQL query fails with "ORA-01722: invalid number." What is the probable cause?
A string value compared to a number
Null value in the column
Column does not exist
Table does not exist
Q32
Q32 A query returns a "missing keyword" error. What could be the issue?
Missing a semicolon
Incorrect function syntax
Using a reserved keyword
All of the above
Q33
Q33 What is the purpose of PL/SQL in Oracle?
To execute SQL commands dynamically
To manage database users
To add procedural capabilities to SQL
To store data in tables
Q34
Q34 Which block structure is mandatory in a PL/SQL program?
DECLARE and BEGIN
BEGIN and END
DECLARE, BEGIN, and EXCEPTION
BEGIN, EXCEPTION, and END
Q35
Q35 What is a stored procedure in Oracle?
A precompiled collection of SQL and PL/SQL
A type of database table
A type of data index
A database view
Q36
Q36 Which PL/SQL keyword is used to handle exceptions?
CATCH
HANDLE
RAISE
EXCEPTION
Q37
Q37 What is the difference between a function and a procedure in PL/SQL?
A function must return a value; a procedure cannot
A procedure must return a value; a function cannot
Functions and procedures are the same
A function cannot have parameters
Q38
Q38 Which cursor type is used when the SELECT statement retrieves multiple rows?
Static
Dynamic
Explicit
Implicit
Q39
Q39 Write a PL/SQL block to assign a value to a variable and print it.
BEGIN var_name := 10; PRINT var_name; END;
DECLARE var_name NUMBER := 10; BEGIN DBMS_OUTPUT.PUT_LINE(var_name); END;
VAR var_name := 10; PRINT var_name;
DECLARE BEGIN PRINT var_name := 10; END;
Q40
Q40 Write a PL/SQL block to calculate the sum of two numbers.
BEGIN a := 10; b := 20; c := a + b; PRINT c; END;
DECLARE a NUMBER := 10; b NUMBER := 20; c NUMBER; BEGIN c := a + b; DBMS_OUTPUT.PUT_LINE(c); END;
DECLARE BEGIN a := 10; b := 20; c := a + b; PRINT c; END;
VAR a := 10; b := 20; c := a + b; PRINT c;
Q41
Q41 Write a PL/SQL block to iterate through numbers 1 to 5 and print each.
BEGIN FOR i IN 1..5 LOOP DBMS_OUTPUT.PUT_LINE(i); END LOOP; END;
DECLARE BEGIN FOR i IN 1..5 LOOP DBMS_OUTPUT.PUT_LINE(i); END LOOP; END;
DECLARE i NUMBER; BEGIN FOR i IN 1..5 LOOP DBMS_OUTPUT.PUT_LINE(i); END LOOP; END;
BEGIN LOOP i FROM 1 TO 5 PRINT i; END LOOP; END;
Q42
Q42 A PL/SQL block fails with the error "ORA-06502: numeric or value error." What is the likely cause?
Invalid column name
Datatype mismatch
Missing keyword
Incorrect table name
Q43
Q43 A PL/SQL block returns "ORA-01403: no data found." What should be done to handle this?
Add a WHEN NO_DATA_FOUND exception
Add a NULL value check
Use an IF statement
Use a CONTINUE statement
Q44
Q44 A PL/SQL procedure fails to execute due to "ORA-00904: invalid identifier." What could be the issue?
Variable not declared
Invalid table name
Incorrect datatype
None of the above
Q45
Q45 What is the primary purpose of a table in Oracle Database?
To store data in rows and columns
To define database schema
To optimize queries
To store indexes
Q46
Q46 What is the role of a view in Oracle Database?
To create backups
To restrict access to specific data
To optimize indexes
To store large amounts of data
Q47
Q47 Which type of index automatically creates when a primary key is defined?
Bitmap Index
Unique Index
Composite Index
B-Tree Index
Q48
Q48 How do materialized views differ from standard views in Oracle Database?
They are virtual tables
They store precomputed results
They only support SELECT queries
They are created automatically
Q49
Q49 Which command is used to drop a table in Oracle Database?
DELETE TABLE table_name;
DROP TABLE table_name;
REMOVE table_name;
TRUNCATE TABLE table_name;
Q50
Q50 What is the difference between a clustered and non-clustered index?
Clustered indexes sort data physically; non-clustered do not
Non-clustered indexes are faster
Clustered indexes do not sort data
Clustered indexes only work on primary keys
Q51
Q51 Write a query to create a table with columns id (NUMBER) and name (VARCHAR2).
CREATE TABLE test_table (id NUMBER, name VARCHAR2(50));
CREATE TABLE test_table (id INTEGER, name STRING);
CREATE test_table id NUMBER, name VARCHAR;
DEFINE TABLE test_table (id NUM, name CHAR);
Q52
Q52 Write a query to create an index on the "employee_id" column of the "employees" table.
CREATE INDEX emp_idx ON employees(employee_id);
CREATE TABLE emp_idx ON employees(employee_id);
CREATE INDEX employee_id ON employees;
INDEX emp_idx ON employees(employee_id);
Q53
Q53 Write a query to create a materialized view "mv_sales" to store aggregated sales data.
CREATE MATERIALIZED VIEW mv_sales AS SELECT SUM(sales) FROM transactions;
CREATE VIEW mv_sales AS SELECT SUM(sales) FROM transactions;
CREATE TABLE mv_sales AS SELECT SUM(sales) FROM transactions;
CREATE AGGREGATE VIEW mv_sales AS SELECT SUM(sales) FROM transactions;
Q54
Q54 A query fails with "ORA-00942: table or view does not exist." What is the probable cause?
Table name is misspelled
User lacks access privileges
Table does not exist
Insufficient privileges
Q55
Q55 An index is not being used in a query execution plan. What might be the cause?
The index is invalid
The index is fragmented
The query does not reference indexed columns
Query performance degraded
Q56
Q56 A materialized view fails to refresh with "ORA-12012." What could be the issue?
Incorrect query definition
Missing privileges
Lack of space
Query not optimized
Q57
Q57 What is a transaction in Oracle Database?
A unit of work that can be committed or rolled back
A type of SQL query
A data storage mechanism
A database backup process
Q58
Q58 Which statement begins a transaction in Oracle Database?
BEGIN TRANSACTION
START TRANSACTION
Implicitly starts with first DML statement
CREATE TRANSACTION
Q59
Q59 What is the purpose of the COMMIT statement in a transaction?
Save changes permanently
Undo all changes
Perform a backup
Lock the table
Q60
Q60 What happens when a ROLLBACK statement is executed?
Locks the database
Reverts changes made during the current transaction
Deletes all data
Clears all indexes