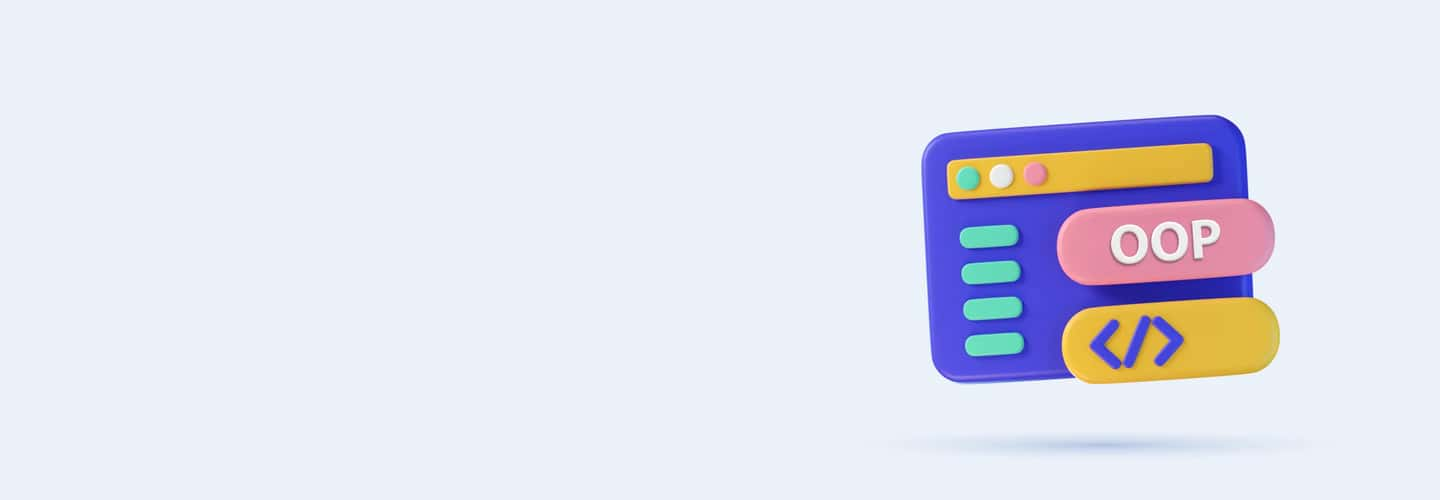
Q121
Q121 A developer struggles to add new operations to objects without modifying them.
Which design pattern can simplify the addition of new operations?
Strategy
Visitor
Decorator
Singleton
Q122
Q122 When debugging a large system of interacting objects, it's found that changing one object's state requires changes to others, and the interactions are complex and hard to follow. Which design pattern can help simplify managing these interactions?
Observer
Mediator
Composite
Strategy
Q123
Q123 A software system's performance suffers because it creates too many heavy-weight objects.
Which design pattern could be implemented to reduce the number of objects and improve performance?
Singleton
Flyweight
Prototype
Builder
Q124
Q124 What concept allows an object to change its behavior when its internal state changes?
Inheritance
Polymorphism
State
Strategy
Q125
Q125 Which concept refers to a class that cannot be instantiated and is designed to be subclassed?
Abstract class
Interface
Singleton
Concrete class
Q126
Q126 In OOP, what is dependency injection?
A design pattern where an object supplies the dependencies of another object
A technique for creating objects
A method for managing software dependencies
A programming paradigm for data encapsulation
Q127
Q127 How does the Composite design pattern work in object-oriented design?
It allows treating individual objects and compositions uniformly
It facilitates object aggregation into tree structures
It enables adding new components dynamically
It simplifies object cloning
Q128
Q128 What is the principle of "Separation of Concerns" in software engineering?
Dividing a program into distinct sections
Improving code readability and maintenance
Facilitating parallel development
Allowing easier testing and debugging
Q129
Q129 What does the Liskov Substitution Principle (LSP) state?
Subclasses should be substitutable for their base classes
Objects should be replaceable with instances of their subtypes
Both
Neither
Q130
Q130 How do generics in Java enhance software reusability?
By enabling type-safe code
By reducing the need for casting
By allowing operations on various types
By facilitating code maintenance and readability
Q131
Q131 What is the primary purpose of the Open/Closed Principle in software design?
To allow software entities to be extendable
To prevent modification of existing code
To encourage modular design
To support polymorphism
Q132
Q132 Given the concept of polymorphism, which Java feature allows methods to perform different operations based on the object that invokes them?
Method overloading
Method overriding
Dynamic method dispatch
Static method binding
Q133
Q133 How can reflection be used in Java?
To inspect class properties at runtime
To modify behavior of methods at runtime
To dynamically create objects
To invoke methods at runtime
Q134
Q134 In the context of advanced OOP, what is aspect-oriented programming (AOP)?
A paradigm for modularizing cross-cutting concerns
A technique for separating business logic from system services
A strategy for enhancing code modularity
A method for implementing polymorphism
Q135
Q135 What programming technique uses interfaces to expose the behavior of a class and provides a way to access its functionality through a reference?
Encapsulation
Polymorphism
Inversion of Control (IoC)
Data binding
Q136
Q136 When applying OOP principles, you encounter a situation where adding new types requires changes to existing code.
Which principle might help reduce the need for such changes?
Open/Closed Principle
Single Responsibility Principle
Liskov Substitution Principle
Dependency Inversion Principle
Q137
Q137 Debugging a system, you notice objects are not properly cleaned up, leading to memory leaks.
Which OOP concept can help manage resources more effectively to prevent such issues?
Garbage collection
Resource management
Automatic memory management
Resource pooling
Q138
Q138 A complex system is experiencing issues with changing the behavior of objects at runtime dynamically.
Which OOP concept or pattern could be utilized to simplify dynamic behavior modification?
State pattern
Strategy pattern
Decorator pattern
Behavioral pattern
Q139
Q139 When debugging, you find that changes to one part of the system unexpectedly affect another part.
This is likely due to improper use of which OOP concept?
Encapsulation
Inheritance
Polymorphism
Global variables
Q140
Q140 What is the primary purpose of unit testing in software development?
To validate that each unit of the software performs as designed
To check the performance of the software under load
To ensure the software meets client requirements
To assess the software's security features
Q141
Q141 Which of the following is NOT a characteristic of a good unit test?
It runs quickly
It requires access to external resources like a database
It can be run in isolation
It tests a single logical concept in the system
Q142
Q142 What does Test-Driven Development (TDD) entail?
Writing tests after developing the code
Writing tests before writing the code to be tested
Only writing tests for bugs that have been reported
None of the above
Q143
Q143 How does mocking in unit testing work?
By changing the code at runtime to simulate different behaviors
By using fake objects to simulate the behavior of real objects in controlled ways
By removing parts of the code not relevant to the test
None of the above
Q144
Q144 Which JUnit annotation is used to indicate a method should be run before each test method?
@BeforeClass
@Before
@BeforeEach
@PreTest
Q145
Q145 In unit testing, what is the purpose of an assertion?
To cause the test suite to fail if a condition is not met
To mark a test as incomplete or skipped
To document the expected behavior of a test
None of the above
Q146
Q146 How can you ensure that resources are properly released after a test runs in JUnit?
By annotating a cleanup method with @AfterEach
By using a try-finally block inside tests
By manually calling garbage collection after tests
By annotating with @AfterClass
Q147
Q147 What is a common strategy for testing a method that throws an exception under certain conditions?
Catching the exception in the test method and asserting its type
Using the assertThrows method provided by the testing framework
Ignoring the test case
None of the above
Q148
Q148 A unit test unexpectedly fails after recent changes to the codebase.
What is the first step in debugging this failure?
Reviewing the test's output and stack trace for clues
Rewriting the test to pass
Skipping the test until further notice
None of the above
Q149
Q149 After refactoring a class, several related unit tests fail. What is the most likely cause?
The refactoring introduced a bug in the code
The tests are no longer valid due to the changes in the code
Both
Neither
Q150
Q150 A complex system's unit tests are running significantly slower after adding new tests for a recently developed feature.
What might be causing the slowdown?
The new tests are computationally expensive
The tests are improperly sharing state, leading to interference
The setup for the new tests is overly complex
Excessive mocking or object creation in the new tests