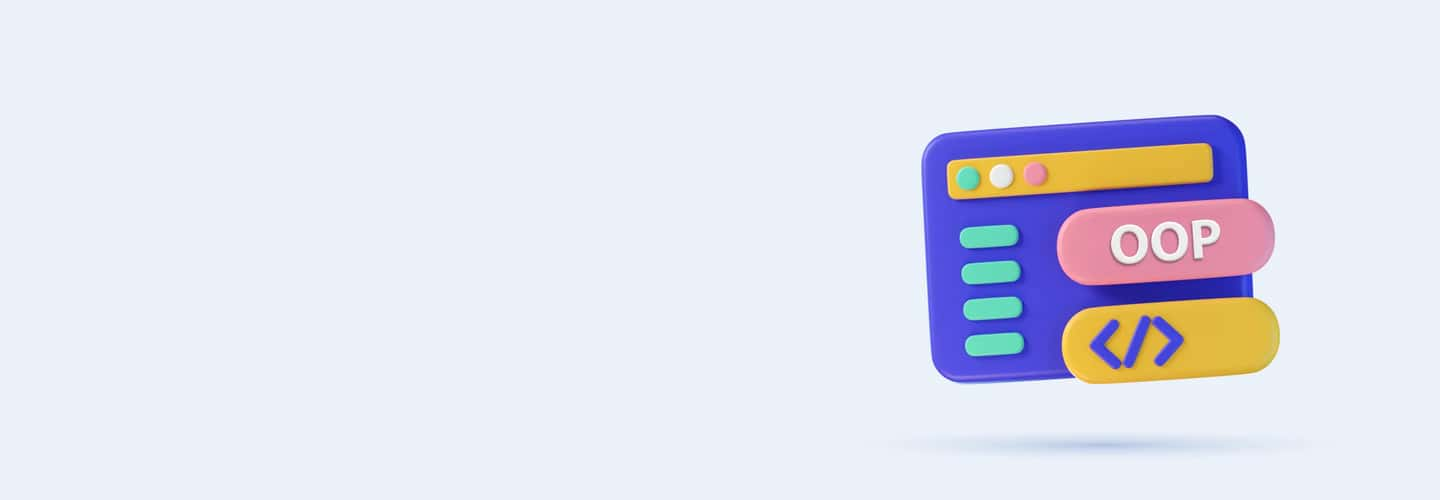
Q91
Q91 Consider the following code snippet:
try { // code that might throw an exception } catch (Exception e) { System.out.println(e.getMessage()); }.
What does e.getMessage() return?
The type of exception thrown
A detailed message about the exception thrown
The name of the class where the exception occurred
The stack trace of where the exception occurred
Q92
Q92 What is the result of attempting to compile and run the following code snippet?
class MyException extends Exception {}
public class Test {
public static void main(String[] args) {
try {
throw new MyException();
}
catch (MyException me) {
System.out.println("MyException caught");
}
catch (Exception e) {
System.out.println("Exception caught"); }
}
}
MyException caught
Exception caught
Compilation error
Runtime error
Q93
Q93 A developer encounters a NullPointerException.
What is a common cause for this exception?
Trying to access a method on a reference that points to null
Incorrect syntax in an if-statement
Accessing an out-of-bounds index in an array
Using an uninitialized variable
Q94
Q94 In debugging a Java program, you notice that an exception is caught, but there's no information about where it occurred.
What is a good practice to follow in the catch block for better debugging?
Throw the exception again
Print the exception stack trace using e.printStackTrace()
Ignore the exception
Log the exception message without the stack trace
Q95
Q95 What must be corrected in a try block that compiles but doesn't catch an exception it was meant to handle?
Ensure the exception is of the correct type that the catch block can catch
Move the code that does not throw the expected exception outside the try block
Replace the try block with a finally block
None of the above
Q96
Q96 What is the Java Collections Framework?
A framework that provides an architecture to store and manipulate a group of objects
A framework for creating collection objects like arrays
A Java framework for threading and concurrency
A set of classes for date and time manipulation
Q97
Q97 Which of the following is not part of the Java Collections Framework?
ArrayList
HashSet
HashMap
Array
Q98
Q98 Which interface represents a collection of objects where duplicates are not allowed?
List
Set
Map
Queue
Q99
Q99 What is the difference between List and Set interfaces in Java?
List allows duplicates and is ordered, while Set does not allow duplicates and is unordered
List is unordered and does not allow duplicates, while Set is ordered and allows duplicates
List and Set both allow duplicates and are ordered
List and Set neither allow duplicates nor are ordered
Q100
Q100 How does a HashMap in Java work internally?
By using arrays to store elements
By using a list to link keys to values
By hashing keys and storing entries in buckets based on hash codes
By organizing elements in a tree structure
Q101
Q101 In Java, which collection type should you use to ensure elements are sorted in natural order?
HashSet
LinkedHashSet
TreeSet
ArrayList
Q102
Q102 What is the primary advantage of using a LinkedHashMap over a HashMap?
LinkedHashMap is more efficient in terms of memory usage
LinkedHashMap allows lookup of values by their hash code
LinkedHashMap maintains insertion order of its elements
LinkedHashMap provides faster access and insertion times
Q103
Q103 What will the following Java code snippet print?
List
list.add("Java");
list.add("Python");
list.add("Java");
System.out.println(list.size());
2
3
4
None of the above
Q104
Q104 Which code snippet correctly iterates over a Map<String, Integer> and prints each key-value pair?
for (String key : map.keySet()) { System.out.println(key + " - " + map.get(key)); }
for (Map.Entry
for (Map.Entry
map.forEach((key, value) -> System.out.println(key + " - " + value));
Q105
Q105 How can you remove all null elements from a Java List
list.removeAll(Collections.singleton(null));
while(list.contains(null)) { list.remove(null); }
list.filter(Objects::nonNull);
for (String s : list) { if (s == null) { list.remove(s); } }
Q106
Q106 Given a List
list.replaceAll(i -> i * i);
list.stream().map(i -> i * i).collect(Collectors.toList());
for (int i = 0; i < list.size(); i++) { list.set(i, list.get(i) * list.get(i)); }
All of the above
Q107
Q107 A developer finds that modifications to a List are not reflected in an iterating loop, causing ConcurrentModificationException.
What common mistake might cause this issue?
Modifying the list within a foreach loop iterating over it
Using an iterator to modify the list without calling iterator.remove()
Iterating over the list with a standard for loop
None of the above
Q108
Q108 A NullPointerException is thrown when attempting to use a sort method on a List
What could be the cause?
The list is null
The list contains null elements
The comparator passed to the sort method is null
All of the above
Q109
Q109 When using a HashSet, a developer notices that it contains duplicate elements.
What is a possible explanation for this behavior?
The equals and hashCode methods are not overridden in the elements' class
The HashSet is incorrectly instantiated
A LinkedHashSet was used instead, which allows duplicates
The elements are of a type that does not support comparison
Q110
Q110 Why might adding elements to a HashMap result in those elements not being retrievable even with the correct key?
The keys used are mutable and were modified after insertion
The HashMap was not properly initialized
The get method is called with the wrong type of key
The HashMap is full
Q111
Q111 Which design pattern is used to create a single instance of a class throughout the application?
Factory Method
Singleton
Prototype
Builder
Q112
Q112 What is the main purpose of the Factory Method design pattern?
To create an instance of several derived classes
To ensure a class has only one instance
To construct complex objects step by step
To allow an object to alter its behavior when its internal state changes
Q113
Q113 What design pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation?
Observer
Visitor
Iterator
Composite
Q114
Q114 Which design pattern is primarily concerned with reducing the coupling between loosely related interacting objects?
Mediator
Adapter
Facade
Bridge
Q115
Q115 In which design pattern do objects represent operations to be performed on elements of another structure, and the visitor pattern allows new operations to be added without changing the elements on which it operates?
Observer
Strategy
Visitor
Chain of Responsibility
Q116
Q116 What design pattern describes an object that encapsulates how a set of objects interact?
Observer
Mediator
Chain of Responsibility
Command
Q117
Q117 Which pattern is best demonstrated by allowing different parsing strategies for an XML file and a JSON file, which can be switched at runtime?
Strategy
Observer
Decorator
Factory
Q118
Q118 In which design pattern is a component wrapped by another component to add new behaviors and responsibilities dynamically?
Decorator
Composite
Adapter
Proxy
Q119
Q119 What pattern can be recognized by a structure where objects are represented as tree structures to represent part-whole hierarchies, allowing clients to treat individual objects and compositions uniformly?
Composite
Flyweight
Bridge
Prototype
Q120
Q120 Which pattern involves a request being passed along a chain of objects until one of them handles the request?
Chain of Responsibility
Command
State
Observer