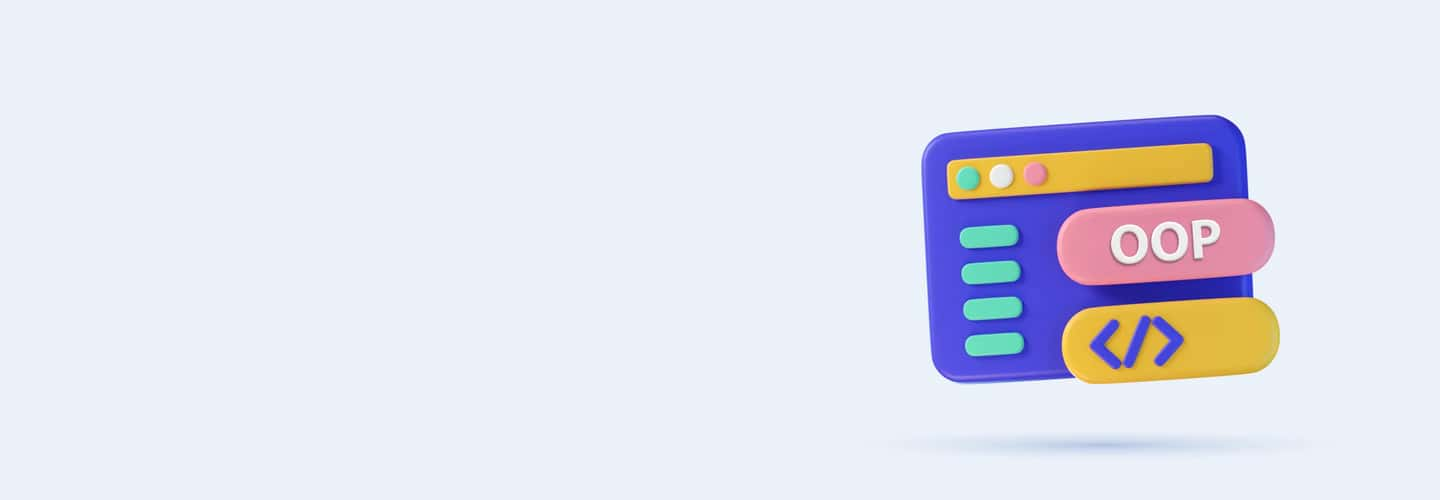
Q31
Q31 In the context of OOP, what is "method overriding"?
Defining a method in a subclass that already exists in the parent class with a different implementation
Increasing the visibility of a method in a subclass
Changing the return type of a method in a subclass
None of the above
Q32
Q32 What does the super keyword in Java do?
It is used to call superclass methods
It defines a superclass
It overrides a method in the superclass
It creates an instance of a superclass
Q33
Q33 Which of the following best describes "dynamic polymorphism"?
Compile-time method binding
The ability to create dynamic classes at runtime
Method overriding where method calls are resolved at runtime
The creation of objects at runtime
Q34
Q34 What is an "abstract class" in Java?
A class that cannot be instantiated and must be inherited
A class that can only contain abstract methods
A class without any implementation
A final class that cannot be extended
Q35
Q35 To achieve polymorphism in Java, one must use:
Interfaces only
Abstract classes only
Either interfaces or abstract classes
Direct class inheritance only
Q36
Q36 Which concept describes the ability of different classes to respond to the same method call in their own unique ways?
Encapsulation
Inheritance
Polymorphism
Composition
Q37
Q37 When does the "diamond problem" occur in programming?
When multiple interfaces are implemented
When a class inherits from two classes with the same method
When multiple classes inherit from a single class
None of the above
Q38
Q38 Which feature distinguishes interfaces from abstract classes in Java?
Interfaces cannot have any method implementations
Interfaces can have only final static fields
Abstract classes can contain non-final methods
Interfaces support multiple inheritance
Q39
Q39 What is the output of the following code snippet in Java?
class Animal {
public void sound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
public void sound() {
System.out.println("Bark");
}
}
public class Test {
public static void main(String[] args) {
Animal myAnimal = new Dog();
myAnimal.sound();
}
}
"Animal sound"
"Bark"
Compilation error
None of the above
Q40
Q40 Given the following Java code, which principle is demonstrated?
interface Flyable {
void fly();
}
class Bird implements Flyable {
public void fly() {
System.out.println("Bird flies");
}
}
class Airplane implements Flyable {
public void fly() {
System.out.println("Airplane flies");
}
}
Inheritance
Encapsulation
Polymorphism
Abstraction
Q41
Q41 In Java, which method signature indicates that a method is overriding a method from its superclass?
A method with the same name but a different return type
A method with the same name and parameter list as in the superclass
A static method with the same name as in the superclass
A method with the same name but different parameter types
Q42
Q42 What will happen if a subclass in Java tries to override a superclass method marked as final?
The subclass method will successfully override the superclass method
The compiler will generate an error
The superclass method will be hidden
None of the above
Q43
Q43 Given two classes, Base and Derived, where Derived extends Base and both classes have a method show() with different implementations.
How is polymorphic behavior achieved when calling show() on a Derived object referenced by a Base type?
By marking show() in Base as final
By using the static keyword in Derived's show() method
By overriding show() in Derived
None of the above
Q44
Q44 A programmer cannot access a superclass method from a subclass in Java.
What is the most likely reason?
The subclass does not extend the superclass
The method in the superclass is marked as private
The method in the superclass is static
None of the above
Q45
Q45 After refactoring a class hierarchy, a method call on a subclass object executes the superclass method instead of the overridden method.
What is the likely cause?
The overridden method in the subclass is marked as private
The method call is statically bound
The subclass method does not correctly override the superclass method due to a signature mismatch
None of the above
Q46
Q46 A Java program throws a ClassCastException when casting an object of a superclass to a subclass.
What is the most probable reason?
The object actually belongs to another unrelated class
The object is of the superclass type and cannot be cast down without an explicit check
The subclass is abstract
None of the above
Q47
Q47 In a Java application utilizing interfaces, a new class implements an interface but fails to provide implementations for all declared methods.
What error will this cause?
NullPointerException
InstantiationException
CompilationError
ClassCastException
Q48
Q48 Which of the following best describes encapsulation?
Combining data and methods into a single unit
Breaking down a complex problem into smaller parts
Allowing an object to perform many forms of behavior
Hiding the internal state of an object from the outside world
Q49
Q49 What is the primary purpose of abstraction in OOP?
To speed up the execution of programs
To reduce code complexity and increase reusability
To hide the internal implementation details and only show the features to the users
To ensure secure data manipulation without external interference
Q50
Q50 Which keyword in Java is used to hide a class member from classes in other packages?
private
protected
public
transient
Q51
Q51 In OOP, abstract methods are:
Methods that are fully implemented
Methods that are declared but not implemented
Methods that cannot be overridden
Methods that must be static
Q52
Q52 What does it mean when a class is declared as abstract?
The class cannot be instantiated directly and must be subclassed
The class does not contain any methods
The class is a final class and cannot have any subclasses
The class can only contain static methods
Q53
Q53 How can encapsulation be achieved in a class?
By declaring all variables as static
By declaring all class methods as final
By making member variables private and providing public getter and setter methods
By using abstract classes only
Q54
Q54 The process of minimizing the exposure of details from one part of a program to another is known as:
Encapsulation
Abstraction
Polymorphism
Inheritance
Q55
Q55 What is the difference between encapsulation and abstraction?
Encapsulation is about hiding the details, abstraction is about showing only essential features
Encapsulation is a design guideline, whereas abstraction is a programming concept
Encapsulation deals with data, and abstraction deals with classes
There is no significant difference
Q56
Q56 Which OOP feature provides a way to protect objects from unintended direct field access?
Inheritance
Polymorphism
Encapsulation
Abstraction
Q57
Q57 What will be the output of the following Java code snippet?
class Encapsulate {
private int num = 10;
public int getNum() {
return num;
}
} public class Test {
public static void main(String[] args) {
Encapsulate obj = new Encapsulate();
System.out.println(obj.getNum());
}
}
10
0
Compilation error
Runtime error
Q58
Q58 Given the following code, identify the abstraction technique used:
abstract class Shape {
abstract void draw();
}
class Rectangle extends Shape {
void draw() {
System.out.println("drawing rectangle");
}
}
Inheritance
Encapsulation
Polymorphism
Abstraction
Q59
Q59 Which of the following changes will make the code below correctly encapsulate the age property?
class Person {
int age; public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Change int age; to private int age;
Add static keyword to age
Remove the setter method
Change public methods to private
Q60
Q60 How can you ensure that a class adheres to the abstraction principle by not allowing instantiation but permitting subclassing?
Declare the class as final
Declare the class as static
Declare the class with private constructors
Declare the class as abstract