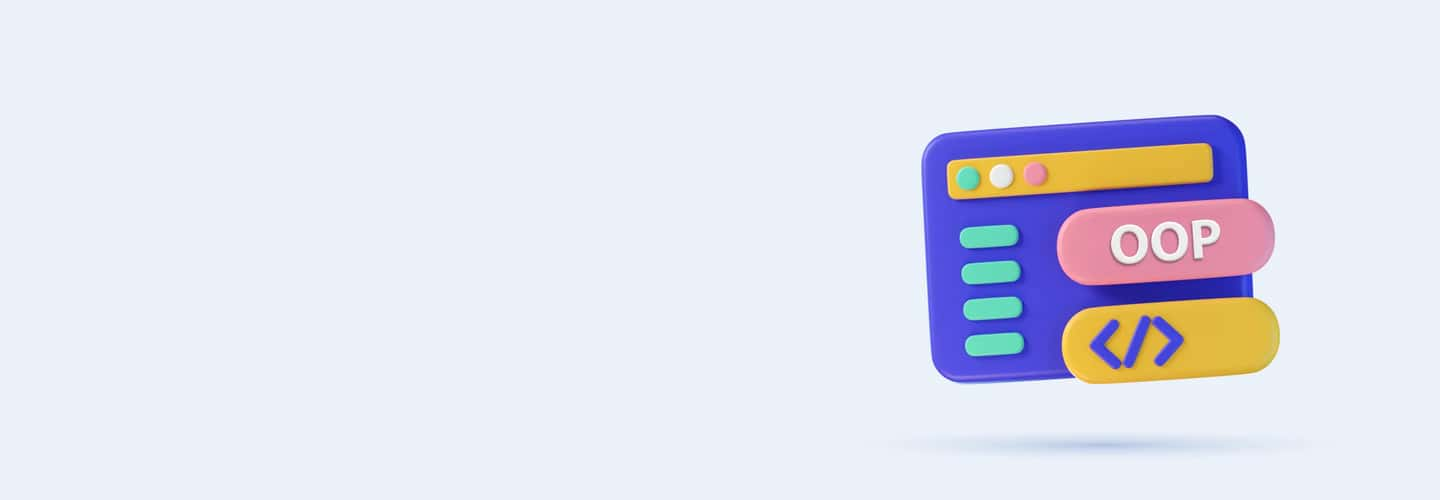
Q1
Q1 Which principle of OOP allows for the same function to be used in different ways?
Inheritance
Polymorphism
Encapsulation
Abstraction
Q2
Q2 What does OOP stand for?
Object-Oriented Programming
Objective Operation Procedure
Oriented Object Programming
Operational Object Programming
Q3
Q3 Which of the following is NOT a basic concept of OOP?
Classes
Objects
Functions
Inheritance
Q4
Q4 OOP aims to make software development more:
Error-prone
Flexible
Complicated
Sequential
Q5
Q5 Which concept of OOP encapsulates the properties (data) and behaviors (methods) of a real-world object into a single entity?
Polymorphism
Encapsulation
Inheritance
Abstraction
Q6
Q6 The concept of hiding the internal implementation details of a class and showing only its functionality is known as:
Encapsulation
Abstraction
Polymorphism
Inheritance
Q7
Q7 In OOP, an "object" is:
An instance of a class
A type of data structure
A programming technique
A method definition
Q8
Q8 What is the output of the following Python code?
class Animal:
def speak(self):
return "makes a sound"
class Dog(Animal):
def speak(self):
return "barks"
print(Dog().speak())
makes a sound
barks
SyntaxError
None
Q9
Q9 Which keyword is used to create a class in Java?
class
Class
object
Object
Q10
Q10 A programmer defines a class but fails to create an instance of that class. They try to call a method of the class directly.
What type of error will occur?
Compilation error
Runtime error
Logical error
No error
Q11
Q11 If a class Car has a method drive() that requires no arguments, which of the following calls is correct after creating a Car object named myCar?
Car.drive()
myCar.drive()
drive(myCar)
Car().drive()
Q12
Q12 What is an object in programming?
A piece of data
A runtime entity
A template for creating objects
A function
Q13
Q13 In the context of OOP, what does a class provide?
A blueprint for objects
A specific data type
A method implementation
A programming pattern
Q14
Q14 Which of the following is a characteristic of an object?
Encapsulation
Inheritance
Polymorphism
Identity
Q15
Q15 The process of creating an object from a class is known as:
Encapsulation
Instantiation
Inheritance
Abstraction
Q16
Q16 Which of the following best describes the concept of "state" in an object?
The behavior the object exhibits
The methods the object can execute
The data stored within the object
The class from which the object is derived
Q17
Q17 Which term best describes an instance variable of a class?
A variable defined within a method
A variable accessible by all instances of a class and shared across them
A variable unique to each instance of a class
A constant value that cannot be changed
Q18
Q18 What is the main difference between a class and an object?
A class is a blueprint, while an object is an instance of a class
A class is executable code, while an object is not
A class cannot exist without objects, while an object can exist without classes
A class is used only in object-oriented programming, while objects can be used in any programming paradigm
Q19
Q19 How do objects in OOP communicate with each other?
Through global functions
By calling methods on each other
Using special OOP communication operators
By accessing each other's memory directly
Q20
Q20 Which keyword is used in Java to create a new object?
new
class
object
this
Q21
Q21 Given the following Java code, what is the output?
class Box {
int width;
Box(int w) {
width = w;
}
}
public class Test {
public static void main(String[] args) {
Box myBox = new Box(5);
System.out.println(myBox.width);
}
}
5
0
Error
None
Q22
Q22 What is the result of compiling and running the following code in Java?
class Counter {
private int count = 0;
void increment() {
count++;
}
int getCount() {
return count;
}
}
Compilation error
Runtime error
0
None of the above
Q23
Q23 Which of the following is the correct way to define a constructor in Java?
public void ClassName() {}
ClassName() {}
public ClassName() {}
constructor ClassName() {}
Q24
Q24 Considering the principle of encapsulation, which option correctly makes age a private attribute and provides public getter and setter methods in a Java class?
private int age; public int getAge() { return age; } public void setAge(int a) { age = a; }
int age; public int readAge() { return age; } public void writeAge(int a) { age = a; }
private int age; public int age() { return age; } public void age(int a) { age = a; }
int age; public int getAge() { return age; } public void age(int a) { age = a; }
Q25
Q25 Identify the mistake in the following Java code snippet:
class Student {
private int score;
public static void setScore(int s) {
score = s;
}
}
score should not be private
s is not correctly passed to score
setScore cannot be static as it accesses an instance variable
None of the above
Q26
Q26 A programmer attempts to compile the following Java code but encounters an error:
class MyClass { void MyClass() { } }.
What is the mistake?
The constructor has a return type
The class name is incorrect
The constructor is defined as a method
None of the above
Q27
Q27 In the context of Java, what does the following error indicate?
java.lang.NullPointerException
An attempt to access a class file that does not exist
An attempt to use a reference that points to no location in memory
Incorrect syntax
None of the above
Q28
Q28 After adding a new feature to a class, a programmer notices that creating an object of that class now throws an error.
What is the MOST likely cause?
The class does not have a constructor
The new feature conflicts with existing methods
There is a syntax error in the new feature
The class was not compiled after adding the new feature
Q29
Q29 Which keyword is used in Java to inherit a class?
extends
implements
inherits
super
Q30
Q30 Polymorphism in OOP allows for:
objects to take on multiple forms
methods to execute concurrently
classes to encapsulate data and methods
objects to be instantiated without classes