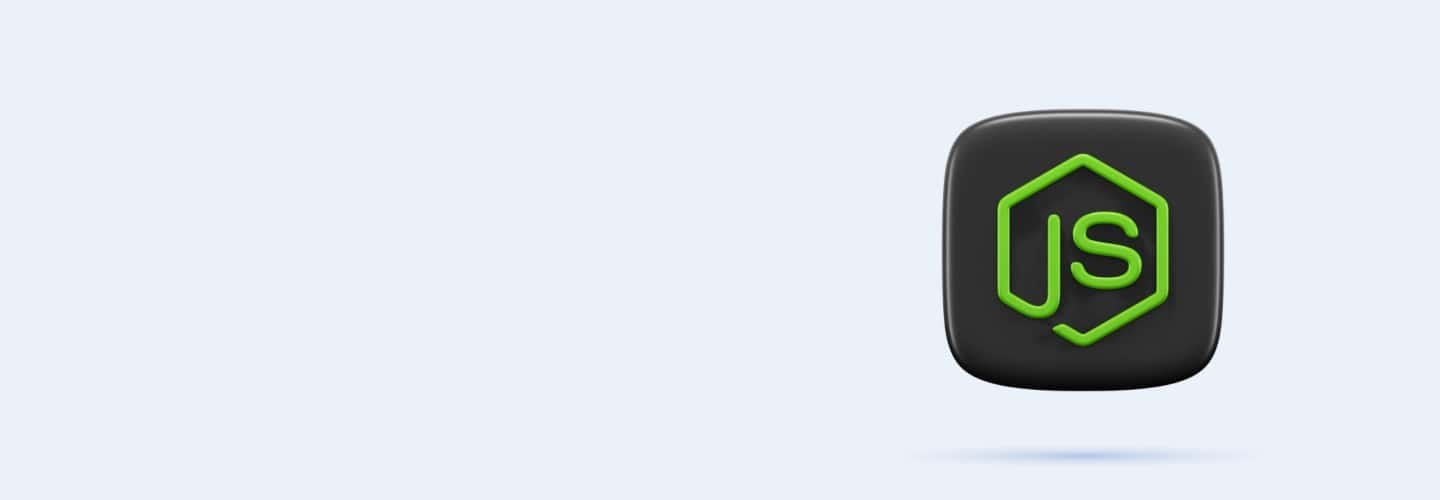
Q121
Q121 What should you check if an Express.js application is not handling errors properly?
Middleware order
Route definitions
Database connections
Server configuration
Q122
Q122 How can you debug an error that occurs in a Node.js application?
Using console.log()
Using a debugger
Using logging libraries
Using all of these methods
Q123
Q123 What is the best practice for handling promise rejections in Node.js?
Using .catch() method
Using try-catch block
Using .then() method
Ignoring rejections
Q124
Q124 What is the primary purpose of profiling in Node.js?
To improve security
To analyze performance
To handle HTTP requests
To manage database connections
Q125
Q125 Which built-in Node.js module is used for debugging?
fs
util
path
inspector
Q126
Q126 What is the V8 profiler used for in Node.js?
To profile JavaScript execution
To debug HTTP requests
To manage file operations
To handle database connections
Q127
Q127 Which tool can be used to visualize the performance profile of a Node.js application?
npm
node-gyp
chrome-devtools
express
Q128
Q128 How do you start the Node.js inspector?
node inspect app.js
node debug app.js
node start app.js
node profiler app.js
Q129
Q129 How do you capture a CPU profile in Node.js?
node --prof app.js
node --inspect app.js
node --cpu app.js
node --profile app.js
Q130
Q130 What should you check if a Node.js application is running slowly?
Memory usage
CPU usage
I/O operations
All of these
Q131
Q131 How can you debug memory leaks in a Node.js application?
Using a debugger
Using heap snapshots
Using CPU profiles
Using process.exit()
Q132
Q132 What is the best practice for logging in a Node.js application?
Using console.log()
Using a logging library
Using error-handling middleware
Using process.stdout
Q133
Q133 What is the primary purpose of testing in Node.js applications?
To improve performance
To find and fix bugs
To manage database connections
To handle HTTP requests
Q134
Q134 Which testing framework is commonly used in Node.js applications?
React
Express
Mocha
Nginx
Q135
Q135 What is the purpose of a test runner in Node.js?
To execute test cases
To handle HTTP requests
To manage database connections
To debug code
Q136
Q136 What is the advantage of using assertion libraries in testing?
To improve performance
To provide descriptive error messages
To manage HTTP requests
To handle file operations
Q137
Q137 How do you write a simple test case using Mocha?
describe('test', function() { it('should pass', function() { assert(true); }); });
test('should pass', function() { assert(true); });
mocha('test', function() { it('should pass', function() { assert(true); }); });
describe('test', function() { test('should pass', function() { assert(true); }); });
Q138
Q138 How do you mock a function using Sinon?
sinon.mock(functionName)
sinon.stub(object, 'method')
sinon.spy(functionName)
sinon.fake(functionName)
Q139
Q139 What should you do if a test case fails unexpectedly?
Check the test logic
Check the implementation code
Check the test environment
All of these
Q140
Q140 How can you debug a failing test case in Node.js?
Using console.log()
Using a debugger
Using a testing framework
Using process.exit()
Q141
Q141 What is the best practice for writing test cases in Node.js?
Writing long and complex tests
Testing multiple functionalities in one test
Writing small and focused tests
Ignoring edge cases
Q142
Q142 What is the primary purpose of deploying a Node.js application?
To run the application in a production environment
To improve development speed
To manage database connections
To handle HTTP requests
Q143
Q143 Which of the following is a common method for deploying Node.js applications?
FTP
SSH
Docker
SMTP
Q144
Q144 What is the purpose of using a process manager like PM2?
To write application code
To handle database operations
To manage application processes
To create user interfaces
Q145
Q145 What is a key benefit of using a reverse proxy like Nginx with a Node.js application?
To handle static files
To balance load and improve scalability
To manage database connections
To write application code
Q146
Q146 How do you start a Node.js application with PM2?
pm2 init app.js
pm2 start app.js
pm2 run app.js
pm2 serve app.js
Q147
Q147 How do you create a Dockerfile for a Node.js application?
FROM node:latest
COPY package*.json ./
RUN npm install
CMD ["node", "app.js"]
Q148
Q148 What should you check if a deployed Node.js application is not responding?
Application logs
Database connection
Network configuration
All of these
Q149
Q149 How can you monitor the performance of a deployed Node.js application?
Using console.log()
Using monitoring tools like New Relic or Datadog
Using a debugger
Using npm scripts
Q150
Q150 What is the best practice for handling environment variables in a Node.js application?
Hardcoding them in the application
Storing them in a .env file
Passing them as command-line arguments
Storing them in a database