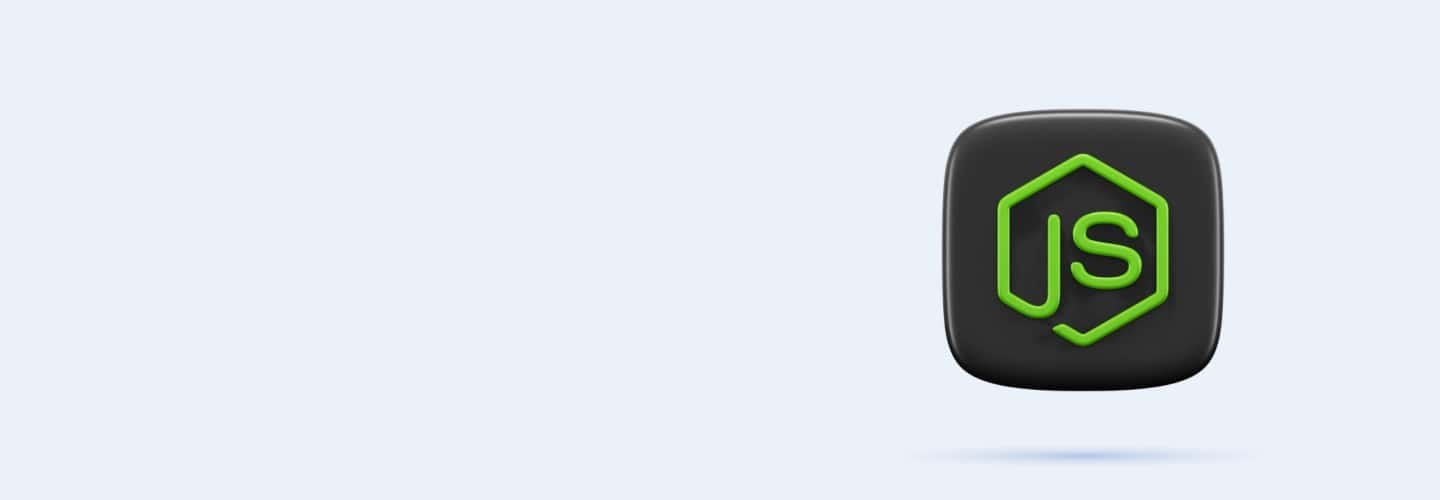
Q91
Q91 How do you handle errors in a REST API using Express.js?
app.use((err, req, res, next) => { res.status(500).json({ error: err.message }); })
app.use((req, res, next) => { res.status(500).json({ error: 'Server error' }); })
app.use((req, res, next) => { res.json({ error: 'Server error' }); })
app.use((err, req, res, next) => { res.send({ error: 'Server error' }); })
Q92
Q92 What should you check if a REST API is not returning the expected data?
API endpoint
HTTP method
Request payload
Response format
Q93
Q93 How can you debug a REST API request in Node.js?
Using console.log()
Using a debugger
Using Postman
Checking network tab in browser
Q94
Q94 What is a common cause of CORS errors in REST APIs?
Incorrect URL
Invalid JSON
Cross-origin requests
Server overload
Q95
Q95 Which module is commonly used for MongoDB integration in Node.js?
mongoose
mysql
sequelize
pg
Q96
Q96 What does the mongoose.connect() method do?
Closes the database connection
Opens a new database connection
Runs a database query
Initializes the Mongoose library
Q97
Q97 Which method is used to save a document to a MongoDB collection in Mongoose?
save
insert
create
update
Q98
Q98 What is a schema in Mongoose?
A function to run database queries
A middleware function
A blueprint for defining the structure of a document
A method for connecting to the database
Q99
Q99 What is the purpose of model validation in Mongoose?
To validate JavaScript code
To ensure data integrity
To check database connection
To manage middleware
Q100
Q100 How do you define a schema in Mongoose?
new mongoose.Schema()
mongoose.createSchema()
mongoose.initSchema()
new Schema()
Q101
Q101 How do you save a new document to a MongoDB collection using Mongoose?
const doc = new Model(data); doc.save();
Model.insert(data);
Model.create(data);
const doc = new Model(data); doc.insert();
Q102
Q102 How do you update an existing document in Mongoose?
Model.update(query, update)
Model.updateOne(query, update)
Model.modify(query, update)
Model.change(query, update)
Q103
Q103 What should you check if a MongoDB connection fails in Mongoose?
Connection string
Model definition
Schema validation
Middleware
Q104
Q104 How can you debug database queries in Mongoose?
Using a debugger
Using mongoose.set('debug', true)
Checking model definitions
Checking schema definitions
Q105
Q105 What is the primary purpose of JWT (JSON Web Token) in authentication?
To store session data
To encrypt passwords
To transmit information securely
To manage user roles
Q106
Q106 Which of the following is a common strategy for implementing authentication in Express.js applications?
OAuth
JWT
Session-based
Cookie-based
Q107
Q107 What is the main advantage of using OAuth for authentication?
Simplicity
Improved security
Speed
Ease of use
Q108
Q108 What is the purpose of the Passport.js library in a Node.js application?
To manage database connections
To handle routing
To authenticate requests
To parse JSON bodies
Q109
Q109 How do you initialize Passport.js in an Express.js application?
app.use(passport.init());
app.use(passport.start());
app.use(passport.initialize());
app.use(passport.begin());
Q110
Q110 How do you protect a route using JWT in Express.js?
app.use(jwtAuth);
app.get('/protected', jwtAuth, (req, res) => {...});
app.use(jwtAuth());
app.get('/protected', (req, res) => { jwtAuth(); ... });
Q111
Q111 How do you use Passport.js to authenticate users with Google OAuth?
passport.use(new GoogleStrategy(...));
passport.use(new OAuthStrategy(...));
passport.use(new GoogleAuth(...));
passport.use(new AuthStrategy(...));
Q112
Q112 What should you check if JWT authentication fails in your application?
Token expiry
Token signature
User permissions
Token payload
Q113
Q113 How can you debug issues with Passport.js authentication?
Check strategy configuration
Check middleware order
Check session settings
Check callback URL
Q114
Q114 What is the primary purpose of error handling in Node.js?
To manage database connections
To handle HTTP requests
To catch and manage runtime errors
To improve performance
Q115
Q115 Which module is commonly used for logging errors in a Node.js application?
fs
path
winston
http
Q116
Q116 What is the purpose of a try-catch block in JavaScript?
To handle asynchronous operations
To catch and handle exceptions
To manage HTTP requests
To handle database queries
Q117
Q117 How do you define a custom error in Node.js?
throw new Error('message')
console.error('message')
process.exit(1)
return new Error('message')
Q118
Q118 How do you handle errors in an Express.js middleware function?
Using try-catch blocks
Using if-else statements
Using error-handling middleware
Using callbacks
Q119
Q119 How do you propagate an error in a callback function in Node.js?
return error
throw error
next(error)
process.exit(1)
Q120
Q120 How do you handle uncaught exceptions in a Node.js application?
Using try-catch blocks
Using process.on('uncaughtException', callback)
Using a promise
Using setTimeout