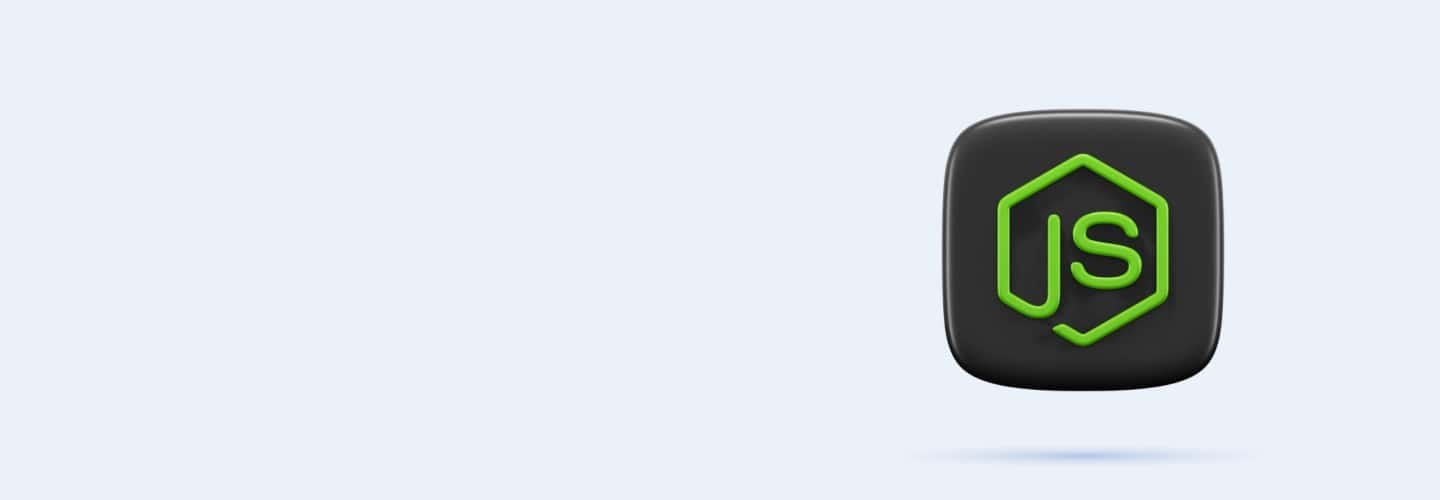
Q61
Q61 How can you debug an HTTP request error in Node.js?
Use console.log()
Use a debugger
Check the response headers
Check the HTTP method
Q62
Q62 What is Express.js primarily used for?
Building command-line tools
Creating web applications
Managing file systems
Handling HTTP requests and responses
Q63
Q63 How do you create a new Express application?
express()
express.createApp()
express.new()
express.init()
Q64
Q64 Which method is used to define a route in Express.js?
app.route()
app.router()
app.getRoute()
app.setRoute()
Q65
Q65 How can you handle HTTP POST requests in Express.js?
app.post()
app.get()
app.put()
app.delete()
Q66
Q66 What is middleware in Express.js?
Functions that execute after route handlers
Functions that execute before route handlers
Functions that handle file uploads
Functions that handle database connections
Q67
Q67 Which method is used to start a server in Express.js?
app.listen()
app.start()
app.run()
app.init()
Q68
Q68 How do you serve static files in Express.js?
app.use(express.static('public'))
app.serve('public')
app.static('public')
app.getStatic('public')
Q69
Q69 What is the output of the following code:
app.get('/', (req, res) => { res.send('Hello, World!'); });
Error
Hello, World!
Empty response
HTML document
Q70
Q70 How do you handle 404 errors in Express.js?
app.use((req, res, next) => { res.status(404).send('Not Found'); })
app.get((req, res, next) => { res.status(404).send('Not Found'); })
app.route((req, res, next) => { res.status(404).send('Not Found'); })
app.handle((req, res, next) => { res.status(404).send('Not Found'); })
Q71
Q71 What should you check if an Express.js route is not working?
Route path
Route method
Route handler
All of these
Q72
Q72 How can you debug an Express.js application?
Using a debugger
Using console.log()
Using nodemon
All of these
Q73
Q73 What is the best practice for error handling in Express.js?
Using try-catch blocks
Using error-handling middleware
Restarting the server
Ignoring errors
Q74
Q74 What is middleware in Express.js?
Functions that execute before route handlers
Functions that execute after route handlers
Functions that handle file uploads
Functions that handle database connections
Q75
Q75 How do you define middleware in Express.js?
app.middleware()
app.use()
app.define()
app.create()
Q76
Q76 What is a common use case for middleware in Express.js?
Handling static files
Routing requests
Logging requests
Rendering views
Q77
Q77 Which middleware function handles errors in Express.js?
app.error()
app.use()
app.catch()
app.handle()
Q78
Q78 How do you add a middleware function that logs request URLs?
app.use((req, res, next) => { console.log(req.url); next(); })
app.log((req, res, next) => { console.log(req.url); next(); })
app.request((req, res, next) => { console.log(req.url); next(); })
app.get((req, res, next) => { console.log(req.url); next(); })
Q79
Q79 How can you handle JSON request bodies in Express.js?
app.use(express.json())
app.handle(express.json())
app.request(express.json())
app.json()
Q80
Q80 How do you define error-handling middleware in Express.js?
app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something broke!'); })
app.use((err, req, res, next) => { console.error(err.stack); res.status(404).send('Not Found'); })
app.error((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something broke!'); })
app.catch((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something broke!'); })
Q81
Q81 What should you check if middleware is not being executed?
Middleware order
Middleware type
Middleware function name
Middleware parameters
Q82
Q82 How can you debug middleware execution in Express.js?
Using console.log()
Using a debugger
Checking middleware order
All of these
Q83
Q83 What does REST stand for?
Representational State Transfer
Real-time State Transfer
Remote Service Technology
Reliable State Transfer
Q84
Q84 Which HTTP method is typically used to create a resource in a REST API?
GET
POST
PUT
DELETE
Q85
Q85 Which status code indicates that a resource was successfully created?
200
201
400
404
Q86
Q86 Which HTTP method is used to update a resource in a REST API?
GET
POST
PUT
DELETE
Q87
Q87 What is the primary advantage of using REST APIs?
Improved security
Statelessness
Stateful operations
Enhanced compatibility with HTML
Q88
Q88 How do you define a route for a GET request in Express.js?
app.get('/path', callback)
app.post('/path', callback)
app.put('/path', callback)
app.delete('/path', callback)
Q89
Q89 How do you parse JSON request bodies in Express.js?
app.use(express.json())
app.use(bodyParser.json())
app.use(jsonParser())
app.use(parseJSON())
Q90
Q90 How do you send a JSON response in Express.js?
res.sendJSON(data)
res.json(data)
res.send(data)
res.responseJSON(data)